在Python中,使用相对路径的方法包括使用os模块、pathlib模块等。可以通过设置路径为相对路径的形式来实现。 其中,os模块提供了多种与操作系统相关的功能,而pathlib模块则是Python 3.4引入的面向对象的路径处理库。建议使用pathlib模块,因为它更加现代和便捷。下面将详细介绍如何使用这两种方法来实现相对路径的转换。
一、使用os模块
os模块是Python标准库中的一个模块,提供了一些与操作系统交互的函数和方法。下面是使用os模块实现相对路径的一些方法。
1、获取当前工作目录
在使用相对路径时,首先要知道当前工作目录。可以使用os.getcwd()
来获取当前工作目录。
import os
current_directory = os.getcwd()
print("Current Directory:", current_directory)
这个方法会返回当前工作目录的绝对路径。
2、构建相对路径
在知道当前工作目录之后,可以使用os.path.join()
来构建相对路径。例如:
import os
当前工作目录
current_directory = os.getcwd()
目标文件的相对路径
relative_path = os.path.join(current_directory, 'subdir', 'file.txt')
print("Relative Path:", relative_path)
3、转换为相对路径
如果已经有了一个绝对路径,想要转换成相对路径,可以使用os.path.relpath()
方法。例如:
import os
绝对路径
absolute_path = '/home/user/project/subdir/file.txt'
转换为相对路径
relative_path = os.path.relpath(absolute_path, '/home/user/project')
print("Relative Path:", relative_path)
4、检查路径是否存在
在操作文件路径之前,最好先检查路径是否存在,可以使用os.path.exists()
方法:
import os
检查路径是否存在
if os.path.exists(relative_path):
print("Path exists:", relative_path)
else:
print("Path does not exist:", relative_path)
5、示例:打开一个文件
下面是一个完整的示例,演示如何使用相对路径来打开一个文件:
import os
当前工作目录
current_directory = os.getcwd()
目标文件的相对路径
relative_path = os.path.join(current_directory, 'subdir', 'file.txt')
检查路径是否存在
if os.path.exists(relative_path):
# 打开文件
with open(relative_path, 'r') as file:
content = file.read()
print("File Content:", content)
else:
print("Path does not exist:", relative_path)
二、使用pathlib模块
pathlib模块是Python 3.4引入的一个模块,提供了一种面向对象的路径处理方法,更加现代和便捷。下面是使用pathlib模块实现相对路径的一些方法。
1、导入pathlib模块
首先需要导入pathlib模块:
from pathlib import Path
2、获取当前工作目录
可以使用Path.cwd()
来获取当前工作目录:
from pathlib import Path
current_directory = Path.cwd()
print("Current Directory:", current_directory)
3、构建相对路径
可以使用Path
对象来构建相对路径,例如:
from pathlib import Path
当前工作目录
current_directory = Path.cwd()
目标文件的相对路径
relative_path = current_directory / 'subdir' / 'file.txt'
print("Relative Path:", relative_path)
4、转换为相对路径
如果已经有了一个绝对路径,想要转换成相对路径,可以使用relative_to()
方法:
from pathlib import Path
绝对路径
absolute_path = Path('/home/user/project/subdir/file.txt')
转换为相对路径
relative_path = absolute_path.relative_to('/home/user/project')
print("Relative Path:", relative_path)
5、检查路径是否存在
可以使用exists()
方法来检查路径是否存在:
from pathlib import Path
检查路径是否存在
if relative_path.exists():
print("Path exists:", relative_path)
else:
print("Path does not exist:", relative_path)
6、示例:打开一个文件
下面是一个完整的示例,演示如何使用相对路径来打开一个文件:
from pathlib import Path
当前工作目录
current_directory = Path.cwd()
目标文件的相对路径
relative_path = current_directory / 'subdir' / 'file.txt'
检查路径是否存在
if relative_path.exists():
# 打开文件
with relative_path.open('r') as file:
content = file.read()
print("File Content:", content)
else:
print("Path does not exist:", relative_path)
三、相对路径的最佳实践
在实际开发中,使用相对路径可以使代码更加灵活和可移植。下面是一些使用相对路径的最佳实践:
1、使用pathlib模块
尽量使用pathlib模块来处理路径,因为它提供了一种更现代和便捷的路径处理方法。
2、避免硬编码路径
尽量避免在代码中硬编码绝对路径,而是使用相对路径。例如:
# 硬编码路径(不推荐)
absolute_path = '/home/user/project/subdir/file.txt'
使用相对路径(推荐)
from pathlib import Path
current_directory = Path.cwd()
relative_path = current_directory / 'subdir' / 'file.txt'
3、使用配置文件
将路径信息存储在配置文件中,避免在代码中硬编码路径。例如,可以使用JSON、YAML等格式的配置文件来存储路径信息。
4、处理路径异常
在操作文件路径时,要处理可能的异常情况。例如,文件不存在、权限不足等情况。可以使用try-except语句来处理这些异常:
from pathlib import Path
当前工作目录
current_directory = Path.cwd()
目标文件的相对路径
relative_path = current_directory / 'subdir' / 'file.txt'
try:
# 检查路径是否存在
if relative_path.exists():
# 打开文件
with relative_path.open('r') as file:
content = file.read()
print("File Content:", content)
else:
print("Path does not exist:", relative_path)
except Exception as e:
print("An error occurred:", e)
5、使用虚拟环境
在开发Python项目时,建议使用虚拟环境来管理依赖项和路径。虚拟环境可以隔离项目的依赖项,避免与系统依赖项发生冲突。可以使用venv
模块来创建和管理虚拟环境:
# 创建虚拟环境
python -m venv myenv
激活虚拟环境
source myenv/bin/activate
安装依赖项
pip install -r requirements.txt
四、常见问题解答
1、什么是相对路径?
相对路径是指相对于当前工作目录的文件或目录路径。与绝对路径不同,相对路径不包含完整的目录结构,而是从当前工作目录开始计算。
2、如何获取当前工作目录?
可以使用os.getcwd()
或Path.cwd()
来获取当前工作目录。例如:
import os
current_directory = os.getcwd()
print("Current Directory:", current_directory)
或
from pathlib import Path
current_directory = Path.cwd()
print("Current Directory:", current_directory)
3、如何构建相对路径?
可以使用os.path.join()
或Path
对象来构建相对路径。例如:
import os
current_directory = os.getcwd()
relative_path = os.path.join(current_directory, 'subdir', 'file.txt')
print("Relative Path:", relative_path)
或
from pathlib import Path
current_directory = Path.cwd()
relative_path = current_directory / 'subdir' / 'file.txt'
print("Relative Path:", relative_path)
4、如何转换为相对路径?
可以使用os.path.relpath()
或relative_to()
方法来转换为相对路径。例如:
import os
absolute_path = '/home/user/project/subdir/file.txt'
relative_path = os.path.relpath(absolute_path, '/home/user/project')
print("Relative Path:", relative_path)
或
from pathlib import Path
absolute_path = Path('/home/user/project/subdir/file.txt')
relative_path = absolute_path.relative_to('/home/user/project')
print("Relative Path:", relative_path)
5、如何检查路径是否存在?
可以使用os.path.exists()
或exists()
方法来检查路径是否存在。例如:
import os
relative_path = 'subdir/file.txt'
if os.path.exists(relative_path):
print("Path exists:", relative_path)
else:
print("Path does not exist:", relative_path)
或
from pathlib import Path
relative_path = Path('subdir/file.txt')
if relative_path.exists():
print("Path exists:", relative_path)
else:
print("Path does not exist:", relative_path)
总结
在Python中,使用相对路径可以使代码更加灵活和可移植。可以使用os模块或pathlib模块来实现相对路径的转换和操作。尽量使用pathlib模块,因为它提供了一种更现代和便捷的路径处理方法。在实际开发中,避免硬编码路径,将路径信息存储在配置文件中,并处理可能的异常情况。通过这些方法和最佳实践,可以更加高效地管理和操作文件路径。
相关问答FAQs:
如何在Python中实现相对路径的使用?
在Python中,实现相对路径的使用主要依赖于os
模块和pathlib
模块。你可以使用os.path
中的os.getcwd()
获取当前工作目录,并结合相对路径进行文件操作。而使用pathlib
模块,可以通过Path
对象轻松地处理路径,无论是相对路径还是绝对路径。比如,可以通过Path('./your_relative_path')
来创建一个相对路径的对象。
相对路径和绝对路径有什么区别?
相对路径是基于当前工作目录的路径,而绝对路径则是从根目录开始的完整路径。使用相对路径可以使代码在不同环境下更具灵活性,因为它依赖于当前目录的结构,而不需要硬编码具体的绝对路径。这在团队协作或代码共享时尤为重要,因为每个人的文件系统结构可能不同。
在Python中如何处理路径中的分隔符问题?
在处理路径时,建议使用os.path.join()
或pathlib
模块来自动处理路径分隔符。不同操作系统使用不同的路径分隔符(如Windows的\
和Unix/Linux的/
),使用这些函数可以避免因为手动拼接路径而引发的错误。此外,pathlib
模块提供的Path
对象会根据操作系统自动选择正确的分隔符,使路径操作更加简便和安全。
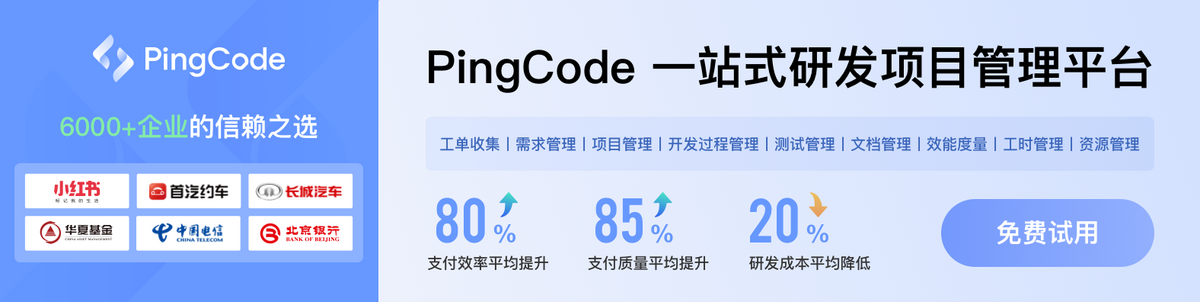