在Python中使用坐标画正方形的步骤:使用matplotlib库、定义正方形的顶点坐标、使用plot函数绘制正方形、显示绘制结果。 其中,使用matplotlib库是关键,因为它是Python中常用的绘图库,可以方便地绘制各种图形。下面将详细介绍如何使用这些步骤来绘制正方形。
一、使用matplotlib库
matplotlib 是Python中最流行的绘图库之一,它提供了丰富的功能,可以绘制各种图形。要使用matplotlib库,首先需要安装它。可以使用以下命令安装:
pip install matplotlib
安装完成后,可以在Python代码中导入matplotlib库。通常,我们会使用matplotlib.pyplot模块来进行绘图。
import matplotlib.pyplot as plt
二、定义正方形的顶点坐标
要绘制正方形,我们首先需要定义正方形的四个顶点坐标。假设我们要绘制一个边长为L的正方形,并且它的左下角顶点坐标为(x, y)。那么,正方形的四个顶点坐标分别为:
- (x, y) —— 左下角顶点
- (x + L, y) —— 右下角顶点
- (x + L, y + L) —— 右上角顶点
- (x, y + L) —— 左上角顶点
此外,为了绘制完整的正方形,我们还需要将左下角顶点再添加一次,使得绘图时能闭合。
x = 0
y = 0
L = 5
定义正方形的顶点坐标
vertices = [(x, y), (x + L, y), (x + L, y + L), (x, y + L), (x, y)]
三、使用plot函数绘制正方形
有了顶点坐标之后,可以使用matplotlib库的plot函数来绘制正方形。plot函数需要分别传入x坐标列表和y坐标列表。
# 提取顶点的x和y坐标
x_coords, y_coords = zip(*vertices)
使用plot函数绘制正方形
plt.plot(x_coords, y_coords, marker='o')
在这里,marker='o'
参数用于在顶点处绘制圆点,使得顶点更加明显。
四、显示绘制结果
最后,使用matplotlib库的show函数显示绘制结果。
# 显示绘制结果
plt.title("Square using Coordinates")
plt.xlabel("X-axis")
plt.ylabel("Y-axis")
plt.grid(True)
plt.axis("equal")
plt.show()
这段代码设置了图形的标题和坐标轴标签,并启用了网格和等比例坐标轴显示。这样,我们就可以清晰地看到绘制的正方形。
示例代码
将上述步骤整合到一起,完整的Python代码如下:
import matplotlib.pyplot as plt
定义正方形的顶点坐标
x = 0
y = 0
L = 5
vertices = [(x, y), (x + L, y), (x + L, y + L), (x, y + L), (x, y)]
提取顶点的x和y坐标
x_coords, y_coords = zip(*vertices)
使用plot函数绘制正方形
plt.plot(x_coords, y_coords, marker='o')
显示绘制结果
plt.title("Square using Coordinates")
plt.xlabel("X-axis")
plt.ylabel("Y-axis")
plt.grid(True)
plt.axis("equal")
plt.show()
运行这段代码,将会绘制出一个左下角顶点坐标为(0, 0),边长为5的正方形。
五、扩展:绘制任意位置和大小的正方形
以上示例中,正方形的左下角顶点坐标为(0, 0),边长为5。实际上,我们可以绘制任意位置和大小的正方形,只需修改顶点的初始坐标和边长即可。例如,绘制一个左下角顶点坐标为(2, 3),边长为7的正方形:
import matplotlib.pyplot as plt
定义正方形的顶点坐标
x = 2
y = 3
L = 7
vertices = [(x, y), (x + L, y), (x + L, y + L), (x, y + L), (x, y)]
提取顶点的x和y坐标
x_coords, y_coords = zip(*vertices)
使用plot函数绘制正方形
plt.plot(x_coords, y_coords, marker='o')
显示绘制结果
plt.title("Square using Coordinates")
plt.xlabel("X-axis")
plt.ylabel("Y-axis")
plt.grid(True)
plt.axis("equal")
plt.show()
这样,就可以绘制出一个左下角顶点坐标为(2, 3),边长为7的正方形。
六、更多绘图选项
matplotlib库提供了丰富的绘图选项,可以根据需要调整正方形的颜色、线型、线宽等参数。例如,可以使用以下代码绘制一个红色、虚线边框、线宽为2的正方形:
import matplotlib.pyplot as plt
定义正方形的顶点坐标
x = 0
y = 0
L = 5
vertices = [(x, y), (x + L, y), (x + L, y + L), (x, y + L), (x, y)]
提取顶点的x和y坐标
x_coords, y_coords = zip(*vertices)
使用plot函数绘制正方形
plt.plot(x_coords, y_coords, color='red', linestyle='--', linewidth=2, marker='o')
显示绘制结果
plt.title("Square using Coordinates")
plt.xlabel("X-axis")
plt.ylabel("Y-axis")
plt.grid(True)
plt.axis("equal")
plt.show()
这段代码使用了 color
参数设置线条颜色为红色,linestyle
参数设置线条样式为虚线,linewidth
参数设置线条宽度为2。
七、使用函数封装绘制正方形
为了方便多次调用绘制正方形的代码,可以将其封装成一个函数。这样,只需传入顶点坐标和边长,就可以绘制任意正方形。
import matplotlib.pyplot as plt
def draw_square(x, y, L, color='blue', linestyle='-', linewidth=1):
# 定义正方形的顶点坐标
vertices = [(x, y), (x + L, y), (x + L, y + L), (x, y + L), (x, y)]
# 提取顶点的x和y坐标
x_coords, y_coords = zip(*vertices)
# 使用plot函数绘制正方形
plt.plot(x_coords, y_coords, color=color, linestyle=linestyle, linewidth=linewidth, marker='o')
# 显示绘制结果
plt.title("Square using Coordinates")
plt.xlabel("X-axis")
plt.ylabel("Y-axis")
plt.grid(True)
plt.axis("equal")
plt.show()
调用函数绘制正方形
draw_square(0, 0, 5)
draw_square(2, 3, 7, color='green', linestyle='--', linewidth=2)
这段代码定义了一个 draw_square
函数,可以传入顶点坐标、边长、颜色、线条样式和线宽等参数,方便绘制不同的正方形。
八、在不同的坐标系中绘制正方形
在一些情况下,我们可能需要在不同的坐标系中绘制正方形。matplotlib库提供了多种坐标系选项,例如极坐标系、对数坐标系等。下面将介绍如何在这些坐标系中绘制正方形。
在极坐标系中绘制正方形
极坐标系使用极径和极角来描述点的位置。要在极坐标系中绘制正方形,可以使用 polar
参数创建极坐标轴,并转换顶点坐标。
import matplotlib.pyplot as plt
import numpy as np
定义正方形的顶点坐标
x = 1
y = 1
L = 2
vertices = [(x, y), (x + L, y), (x + L, y + L), (x, y + L), (x, y)]
提取顶点的x和y坐标
x_coords, y_coords = zip(*vertices)
转换为极坐标
r = np.sqrt(np.array(x_coords)<strong>2 + np.array(y_coords)</strong>2)
theta = np.arctan2(y_coords, x_coords)
使用plot函数绘制正方形
plt.polar(theta, r, marker='o')
显示绘制结果
plt.title("Square in Polar Coordinates")
plt.show()
这段代码首先计算每个顶点的极径和极角,然后使用 polar
函数绘制正方形。
在对数坐标系中绘制正方形
对数坐标系使用对数刻度,可以更好地展示数据的变化。要在对数坐标系中绘制正方形,可以使用 set_xscale
和 set_yscale
函数设置对数刻度。
import matplotlib.pyplot as plt
定义正方形的顶点坐标
x = 1
y = 1
L = 2
vertices = [(x, y), (x + L, y), (x + L, y + L), (x, y + L), (x, y)]
提取顶点的x和y坐标
x_coords, y_coords = zip(*vertices)
使用plot函数绘制正方形
plt.plot(x_coords, y_coords, marker='o')
设置对数坐标系
plt.xscale('log')
plt.yscale('log')
显示绘制结果
plt.title("Square in Logarithmic Coordinates")
plt.xlabel("X-axis")
plt.ylabel("Y-axis")
plt.grid(True)
plt.show()
这段代码使用 xscale
和 yscale
函数将坐标轴设置为对数刻度,并绘制正方形。
九、总结
在本文中,我们详细介绍了如何使用Python的matplotlib库绘制正方形。具体步骤包括安装和导入matplotlib库、定义正方形的顶点坐标、使用plot函数绘制正方形、显示绘制结果。此外,还介绍了如何绘制任意位置和大小的正方形、使用函数封装绘制正方形、在不同的坐标系中绘制正方形等高级用法。
通过学习这些内容,相信你已经掌握了如何在Python中使用坐标绘制正方形的技巧,并能够根据实际需求进行调整和扩展。希望本文对你有所帮助,祝你在Python绘图中取得更大的进步。
相关问答FAQs:
如何在Python中使用坐标系统绘制正方形?
在Python中,可以使用多种库来绘制正方形,例如Matplotlib和Turtle。使用Matplotlib时,可以通过定义正方形的四个顶点的坐标,然后利用plt.plot()函数将这些点连接起来。Turtle库则提供了更直观的绘图方式,可以通过设置画笔的方向和移动距离来绘制正方形。
在绘制正方形时,如何确定坐标的起始点?
起始点的选择通常取决于你希望正方形在绘图区域中的位置。一般可以选择坐标系的原点(0, 0)作为起点,之后根据正方形的边长来确定其他三个顶点的坐标。例如,如果正方形的边长为5,起始点为(0, 0),那么其他顶点分别为(5, 0)、(5, 5)和(0, 5)。
使用Turtle库绘制正方形的代码示例是什么?
使用Turtle库绘制正方形非常简单。可以通过以下代码实现:
import turtle
t = turtle.Turtle()
for _ in range(4):
t.forward(100) # 设置边长为100
t.right(90) # 每次右转90度
turtle.done()
此代码段将创建一个边长为100的正方形,您可以根据需要调整边长的值。
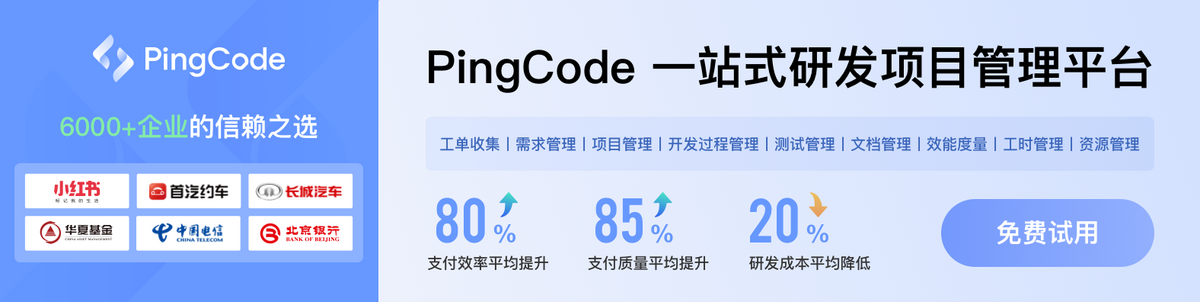