在Python中调整字体大小,可以通过使用各种图形库,如Matplotlib、Tkinter、Pygame等来实现,每个库有其特定的方法和属性来设置字体大小。本文将详细介绍如何使用这些库来调整字体大小。
Matplotlib、Tkinter、Pygame是一些在Python中常用的图形库,能够方便地实现字体大小的调整。接下来,我们将详细介绍如何在每个库中实现这一功能。
一、MATPLOTLIB
Matplotlib 是一个广泛用于绘制图形的库,特别是在科学计算和数据可视化领域。调整字体大小在Matplotlib中非常简单,可以通过设置字体大小参数来实现。
1、使用默认字体大小
在Matplotlib中,字体大小的默认设置可以通过rcParams
字典来调整。例如:
import matplotlib.pyplot as plt
plt.rcParams['font.size'] = 12 # 设置默认字体大小为12
plt.plot([1, 2, 3], [4, 5, 6])
plt.title('Title') # 标题字体大小将使用默认设置
plt.xlabel('X-axis')
plt.ylabel('Y-axis')
plt.show()
2、为特定文本设置字体大小
在创建图形时,可以为特定的文本元素设置字体大小:
import matplotlib.pyplot as plt
plt.plot([1, 2, 3], [4, 5, 6])
plt.title('Title', fontsize=20) # 设置标题字体大小为20
plt.xlabel('X-axis', fontsize=15)
plt.ylabel('Y-axis', fontsize=15)
plt.show()
3、调整图例和标签字体大小
如果需要调整图例和标签的字体大小,可以使用以下方法:
import matplotlib.pyplot as plt
plt.plot([1, 2, 3], [4, 5, 6], label='Line 1')
plt.plot([1, 2, 3], [6, 5, 4], label='Line 2')
plt.title('Title', fontsize=20)
plt.xlabel('X-axis', fontsize=15)
plt.ylabel('Y-axis', fontsize=15)
plt.legend(fontsize=12) # 设置图例字体大小为12
plt.show()
二、TKINTER
Tkinter 是Python的标准GUI库,广泛用于创建桌面应用程序。调整字体大小在Tkinter中可以通过font
模块来实现。
1、设置按钮和标签的字体大小
可以使用tkinter.font
模块来设置按钮和标签的字体大小:
import tkinter as tk
from tkinter import font
root = tk.Tk()
myFont = font.Font(family='Helvetica', size=20) # 创建字体对象
button = tk.Button(root, text="Click Me", font=myFont)
button.pack()
label = tk.Label(root, text="Hello, World!", font=myFont)
label.pack()
root.mainloop()
2、动态调整字体大小
如果需要动态调整字体大小,可以使用config
方法:
import tkinter as tk
from tkinter import font
def increase_font_size():
current_size = myFont['size']
myFont.config(size=current_size + 2)
root = tk.Tk()
myFont = font.Font(family='Helvetica', size=20)
button = tk.Button(root, text="Click Me", font=myFont, command=increase_font_size)
button.pack()
label = tk.Label(root, text="Hello, World!", font=myFont)
label.pack()
root.mainloop()
三、PYGAME
Pygame 是一个用于开发游戏的库,提供了绘制图形、处理输入和播放声音的功能。在Pygame中调整字体大小需要使用pygame.font
模块。
1、设置文本的字体大小
可以通过pygame.font.Font
类来设置文本的字体大小:
import pygame
pygame.init()
screen = pygame.display.set_mode((640, 480))
pygame.display.set_caption('Font Size Example')
font = pygame.font.Font(None, 36) # 设置字体大小为36
text = font.render('Hello, World!', True, (255, 255, 255))
text_rect = text.get_rect(center=(320, 240))
running = True
while running:
for event in pygame.event.get():
if event.type == pygame.QUIT:
running = False
screen.fill((0, 0, 0))
screen.blit(text, text_rect)
pygame.display.flip()
pygame.quit()
2、动态调整字体大小
可以通过创建不同大小的字体对象来动态调整字体大小:
import pygame
pygame.init()
screen = pygame.display.set_mode((640, 480))
pygame.display.set_caption('Font Size Example')
size = 36
font = pygame.font.Font(None, size)
text = font.render('Hello, World!', True, (255, 255, 255))
text_rect = text.get_rect(center=(320, 240))
running = True
while running:
for event in pygame.event.get():
if event.type == pygame.QUIT:
running = False
elif event.type == pygame.KEYDOWN:
if event.key == pygame.K_UP:
size += 2
elif event.key == pygame.K_DOWN:
size -= 2
font = pygame.font.Font(None, size)
text = font.render('Hello, World!', True, (255, 255, 255))
text_rect = text.get_rect(center=(320, 240))
screen.fill((0, 0, 0))
screen.blit(text, text_rect)
pygame.display.flip()
pygame.quit()
四、使用其他库调整字体大小
除了上述常用的库外,还有许多其他Python库可以用来调整字体大小,如ReportLab、PIL/Pillow等。
1、ReportLab
ReportLab 是一个用于生成PDF的库,可以通过setFont
方法来设置字体大小:
from reportlab.lib.pagesizes import letter
from reportlab.pdfgen import canvas
c = canvas.Canvas("example.pdf", pagesize=letter)
c.setFont("Helvetica", 12) # 设置字体大小为12
c.drawString(100, 750, "Hello, World!")
c.setFont("Helvetica", 20) # 设置字体大小为20
c.drawString(100, 700, "Hello, World!")
c.save()
2、PIL/Pillow
PIL (Python Imaging Library) 或其分支Pillow是用于图像处理的库,可以通过ImageFont
模块来设置字体大小:
from PIL import Image, ImageDraw, ImageFont
image = Image.new('RGB', (800, 600), (255, 255, 255))
draw = ImageDraw.Draw(image)
font = ImageFont.truetype("arial.ttf", 36) # 设置字体大小为36
draw.text((100, 100), "Hello, World!", fill=(0, 0, 0), font=font)
font = ImageFont.truetype("arial.ttf", 72) # 设置字体大小为72
draw.text((100, 200), "Hello, World!", fill=(0, 0, 0), font=font)
image.show()
五、结论
通过本文的介绍,我们可以看到,在Python中调整字体大小的方法有很多,具体实现方式取决于所使用的库。无论是用于绘制图形的Matplotlib、创建桌面应用的Tkinter、开发游戏的Pygame,还是生成PDF的ReportLab和处理图像的PIL/Pillow,都提供了相应的方法来设置和调整字体大小。选择合适的库和方法,可以帮助我们在不同的应用场景中灵活地控制字体的显示效果。
相关问答FAQs:
如何在Python中调整图形界面的字体大小?
在Python中,使用Tkinter库创建图形用户界面时,可以通过设置标签或按钮的字体属性来调整字体大小。具体来说,可以使用font
模块中的Font
类来指定字体名称、大小等。例如:
import tkinter as tk
from tkinter import font
root = tk.Tk()
custom_font = font.Font(family="Helvetica", size=12) # 设置字体大小为12
label = tk.Label(root, text="Hello, World!", font=custom_font)
label.pack()
root.mainloop()
上述代码展示了如何创建一个包含自定义字体大小的标签。
在Matplotlib中如何改变图表的字体大小?
Matplotlib是一个用于绘制图表的强大库,用户可以通过fontsize
参数轻松调整图表中各个元素的字体大小。可以在绘制图表时传递该参数,或全局设置。例如:
import matplotlib.pyplot as plt
plt.plot([1, 2, 3], [4, 5, 6])
plt.title('Title', fontsize=16) # 设置标题字体大小
plt.xlabel('X-axis', fontsize=12) # 设置X轴标签字体大小
plt.ylabel('Y-axis', fontsize=12) # 设置Y轴标签字体大小
plt.show()
这种方法可以确保图表的可读性和专业性。
如何在Pandas数据框中调整字体大小?
在使用Pandas库展示数据时,用户可能希望调整表格的字体大小以提高可读性。通过使用style
属性,可以方便地设置字体大小。例如:
import pandas as pd
data = {'Column1': [1, 2, 3], 'Column2': [4, 5, 6]}
df = pd.DataFrame(data)
styled_df = df.style.set_properties(**{'font-size': '12pt'}) # 设置字体大小为12pt
styled_df
这种方法适用于在Jupyter Notebook中展示数据框,能够提供更好的视觉效果。
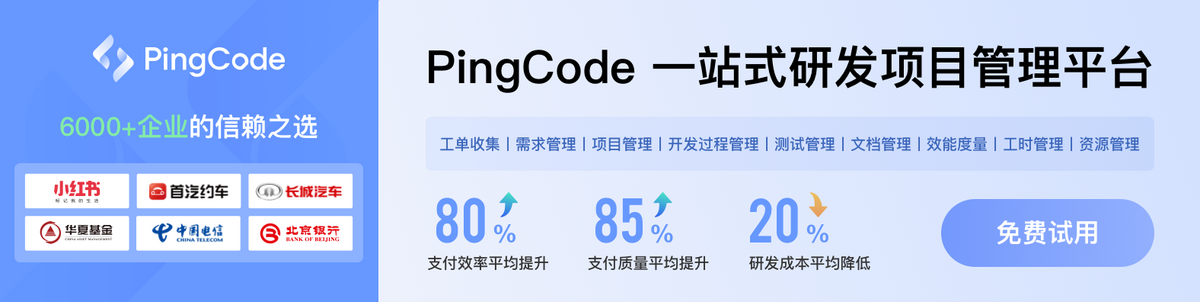