要在Python中制作超级玛丽,可以使用Pygame库、Sprite类、事件处理、碰撞检测。其中,使用Pygame库是最重要的一步,因为它提供了创建游戏所需的所有工具和功能。
制作超级玛丽的详细步骤如下:
一、安装Pygame库
在开始制作游戏之前,您需要先安装Pygame库。您可以使用以下命令来安装Pygame:
pip install pygame
Pygame是一个开源的Python库,专门用于创建视频游戏。它为开发者提供了图像、声音、事件处理和碰撞检测等功能。
二、设置游戏窗口和基本元素
- 初始化Pygame:
import pygame
import sys
pygame.init()
screen = pygame.display.set_mode((800, 600))
pygame.display.set_caption("超级玛丽")
clock = pygame.time.Clock()
- 创建游戏主循环:
running = True
while running:
for event in pygame.event.get():
if event.type == pygame.QUIT:
running = False
screen.fill((0, 0, 0)) # 清屏
pygame.display.flip()
clock.tick(60)
pygame.quit()
sys.exit()
三、加载和显示游戏角色
- 加载图像资源:
mario_image = pygame.image.load('mario.png').convert_alpha()
mario_rect = mario_image.get_rect()
mario_rect.topleft = (100, 100)
- 绘制角色到屏幕上:
screen.blit(mario_image, mario_rect)
四、处理用户输入
- 捕获键盘事件:
keys = pygame.key.get_pressed()
if keys[pygame.K_LEFT]:
mario_rect.x -= 5
if keys[pygame.K_RIGHT]:
mario_rect.x += 5
if keys[pygame.K_UP]:
mario_rect.y -= 5
if keys[pygame.K_DOWN]:
mario_rect.y += 5
- 在主循环中更新角色位置:
screen.blit(mario_image, mario_rect)
五、添加背景和平台
- 加载背景图像:
background_image = pygame.image.load('background.png').convert()
- 绘制背景:
screen.blit(background_image, (0, 0))
- 创建平台类:
class Platform(pygame.sprite.Sprite):
def __init__(self, x, y):
super().__init__()
self.image = pygame.image.load('platform.png').convert_alpha()
self.rect = self.image.get_rect()
self.rect.topleft = (x, y)
- 实例化平台并添加到组中:
platforms = pygame.sprite.Group()
platforms.add(Platform(200, 500))
- 绘制平台:
platforms.draw(screen)
六、碰撞检测与物理引擎
- 重力和跳跃:
gravity = 0.5
mario_speed_y = 0
on_ground = False
if not on_ground:
mario_speed_y += gravity
mario_rect.y += mario_speed_y
if mario_rect.y >= 500:
mario_rect.y = 500
on_ground = True
mario_speed_y = 0
else:
on_ground = False
- 碰撞检测:
hits = pygame.sprite.spritecollide(mario, platforms, False)
if hits:
mario_rect.y = hits[0].rect.top - mario_rect.height
mario_speed_y = 0
on_ground = True
else:
on_ground = False
七、添加敌人和障碍物
- 创建敌人类:
class Enemy(pygame.sprite.Sprite):
def __init__(self, x, y):
super().__init__()
self.image = pygame.image.load('enemy.png').convert_alpha()
self.rect = self.image.get_rect()
self.rect.topleft = (x, y)
self.speed_x = -2
def update(self):
self.rect.x += self.speed_x
- 实例化敌人并添加到组中:
enemies = pygame.sprite.Group()
enemies.add(Enemy(600, 500))
- 更新和绘制敌人:
enemies.update()
enemies.draw(screen)
八、添加游戏状态和得分系统
- 初始化得分和字体:
score = 0
font = pygame.font.Font(None, 36)
- 显示得分:
score_text = font.render(f"Score: {score}", True, (255, 255, 255))
screen.blit(score_text, (10, 10))
- 更新得分:
if mario_rect.colliderect(coin_rect):
score += 10
coin_rect.x = random.randint(0, 800)
九、添加声音效果和背景音乐
- 加载声音和音乐:
jump_sound = pygame.mixer.Sound('jump.wav')
pygame.mixer.music.load('background.mp3')
pygame.mixer.music.play(-1)
- 播放跳跃声音:
if keys[pygame.K_UP] and on_ground:
jump_sound.play()
十、优化和发布游戏
- 优化游戏性能:
- 减少图像大小和数量
- 使用更高效的数据结构
- 避免不必要的计算
- 打包游戏:
- 使用pyinstaller或cx_Freeze将Python脚本打包成可执行文件
pip install pyinstaller
pyinstaller --onefile mario_game.py
通过上述步骤,您可以在Python中创建一个简单的超级玛丽游戏。当然,这只是一个基础示例,实际游戏开发中还有很多细节需要处理,比如更多的关卡设计、更复杂的碰撞检测、动画效果等。希望本文能帮助您更好地理解如何在Python中制作超级玛丽,并激发您的创造力去开发更多有趣的游戏。
相关问答FAQs:
如何在Python中创建一个类似超级玛丽的游戏?
在Python中创建一个类似超级玛丽的游戏,首先需要选择一个合适的游戏开发库。Pygame是一个非常流行且易于使用的库,适合初学者。你需要学习如何使用Pygame来处理图形、声音和用户输入。接下来,设计游戏的基本框架,包括角色、敌人、关卡和碰撞检测等逻辑。通过逐步添加功能,能够逐渐完善你的游戏。
我该如何处理游戏中的角色动画?
角色动画可以通过使用帧动画来实现。在Pygame中,可以将角色的不同状态(如行走、跳跃等)分成不同的图像帧,并根据游戏中的条件切换这些帧。你需要编写逻辑来检测角色的状态,并相应地更新显示的图像。这种方式将使角色看起来更生动和真实。
在制作游戏时,如何设计游戏关卡和敌人?
设计游戏关卡时,考虑到难度逐渐增加是非常重要的。可以先从简单的障碍和敌人开始,然后逐渐引入更复杂的元素。在Pygame中,使用网格系统可以帮助你布局关卡。敌人的行为可以通过编写简单的AI来实现,比如沿着固定路径移动或对玩家的位置做出反应。测试每个关卡的可玩性也是关键,确保玩家在挑战的同时不会感到沮丧。
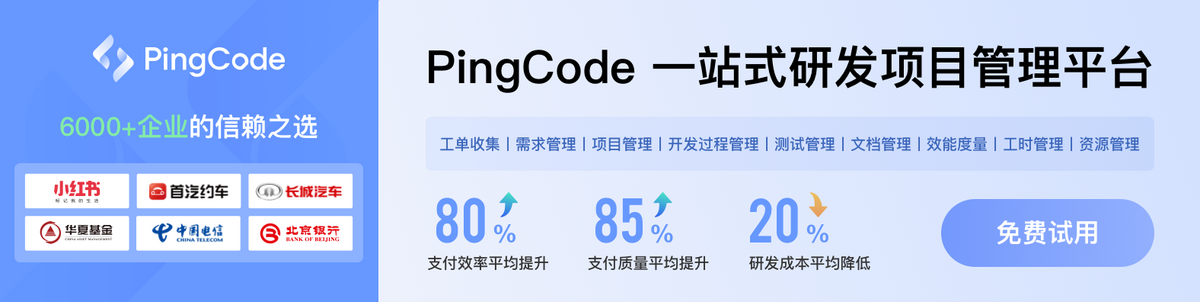