Python获取圆上的所有点的方法有:使用数学公式计算、使用NumPy库生成点、使用matplotlib绘制圆形
在Python中,可以通过多种方法来获取圆上的所有点。首先可以使用数学公式来计算圆上的点坐标,其次可以使用NumPy库来生成点,最后也可以使用matplotlib库来绘制圆形。下面将详细描述如何使用这些方法获取圆上的点。
一、使用数学公式计算
计算圆上点的基本数学公式为:
- 圆心(h, k)
- 半径 r
- 点的极坐标 θ
点的直角坐标公式为:
[ x = h + r \cdot \cos(\theta) ]
[ y = k + r \cdot \sin(\theta) ]
通过改变 θ 的值(从0到2π),可以获取圆上的所有点。
示例代码:
import math
def get_circle_points(h, k, r, num_points):
points = []
for i in range(num_points):
theta = 2 * math.pi * i / num_points
x = h + r * math.cos(theta)
y = k + r * math.sin(theta)
points.append((x, y))
return points
参数设置
h = 0
k = 0
r = 5
num_points = 100
获取圆上的点
circle_points = get_circle_points(h, k, r, num_points)
print(circle_points)
在上面的代码中,通过迭代 num_points 次数来计算θ的不同值,从而获取圆上的所有点。这样可以确保点在圆上均匀分布。
二、使用NumPy库生成点
NumPy库是一个强大的数值计算库,它提供了许多用于科学计算的函数。我们可以使用NumPy库中的 linspace
和 cos
, sin
函数来生成圆上的点。
示例代码:
import numpy as np
def get_circle_points_np(h, k, r, num_points):
theta = np.linspace(0, 2 * np.pi, num_points)
x = h + r * np.cos(theta)
y = k + r * np.sin(theta)
points = np.vstack((x, y)).T
return points
参数设置
h = 0
k = 0
r = 5
num_points = 100
获取圆上的点
circle_points_np = get_circle_points_np(h, k, r, num_points)
print(circle_points_np)
在上面的代码中,使用 linspace
函数生成从0到2π的等差数列,通过向量化操作计算圆上的所有点,这使得代码更加简洁和高效。
三、使用matplotlib绘制圆形
matplotlib 是一个数据可视化库,常用于绘制图表。我们可以使用matplotlib库来绘制圆,并获取圆上的点。
示例代码:
import matplotlib.pyplot as plt
def plot_circle(h, k, r, num_points):
theta = np.linspace(0, 2 * np.pi, num_points)
x = h + r * np.cos(theta)
y = k + r * np.sin(theta)
plt.figure(figsize=(6, 6))
plt.plot(x, y)
plt.scatter(h, k, color='red') # 圆心
plt.title('Circle with center ({}, {}) and radius {}'.format(h, k, r))
plt.xlabel('X')
plt.ylabel('Y')
plt.axis('equal')
plt.grid(True)
plt.show()
参数设置
h = 0
k = 0
r = 5
num_points = 100
绘制圆形
plot_circle(h, k, r, num_points)
在上面的代码中,使用 plot
函数绘制圆形,使用 scatter
函数绘制圆心。通过可视化的方式,可以直观地看到圆上的点。
四、结合使用NumPy和matplotlib
结合使用NumPy和matplotlib,可以获取圆上的点并进行可视化展示。
示例代码:
import numpy as np
import matplotlib.pyplot as plt
def get_and_plot_circle_points(h, k, r, num_points):
theta = np.linspace(0, 2 * np.pi, num_points)
x = h + r * np.cos(theta)
y = k + r * np.sin(theta)
points = np.vstack((x, y)).T
plt.figure(figsize=(6, 6))
plt.plot(x, y)
plt.scatter(h, k, color='red') # 圆心
plt.title('Circle with center ({}, {}) and radius {}'.format(h, k, r))
plt.xlabel('X')
plt.ylabel('Y')
plt.axis('equal')
plt.grid(True)
plt.show()
return points
参数设置
h = 0
k = 0
r = 5
num_points = 100
获取并绘制圆上的点
circle_points = get_and_plot_circle_points(h, k, r, num_points)
print(circle_points)
在上面的代码中,首先使用NumPy生成圆上的点,然后使用matplotlib进行可视化展示,这样既能够获取点的坐标,又能直观展示圆的形状。
五、总结
通过上述方法,可以使用Python获取圆上的所有点。使用数学公式计算的方法简单直接,适用于需要理解基本原理的情况;使用NumPy库生成点的方法更加高效,适用于需要处理大量数据的情况;使用matplotlib绘制圆形的方法便于可视化,适用于需要展示结果的情况。结合使用NumPy和matplotlib,可以兼顾高效计算和可视化展示。选择哪种方法取决于具体的需求和使用场景。
相关问答FAQs:
如何使用Python计算圆的周长和面积?
在Python中,可以使用公式计算圆的周长和面积。周长公式为C = 2 * π * r,面积公式为A = π * r²,其中r是圆的半径。可以使用math库中的π值来进行计算。示例代码如下:
import math
def circle_properties(radius):
circumference = 2 * math.pi * radius
area = math.pi * radius ** 2
return circumference, area
radius = 5
circumference, area = circle_properties(radius)
print(f"周长: {circumference}, 面积: {area}")
如何在Python中绘制圆?
要在Python中绘制圆,可以使用matplotlib库。该库提供了简单的方式来绘制各种形状,包括圆。以下是一个基本的示例:
import matplotlib.pyplot as plt
def draw_circle(radius):
circle = plt.Circle((0, 0), radius, fill=False)
fig, ax = plt.subplots()
ax.add_artist(circle)
ax.set_xlim(-radius-1, radius+1)
ax.set_ylim(-radius-1, radius+1)
ax.set_aspect('equal', 'box')
plt.grid()
plt.show()
draw_circle(5)
如何生成圆周上的点以进行进一步计算或绘图?
可以通过使用极坐标系统生成圆周上的点。给定半径r和圆心坐标,可以使用以下公式计算每个点的坐标:
- x = r * cos(θ)
- y = r * sin(θ)
其中θ是从0到2π的角度。示例代码如下:
import numpy as np
def points_on_circle(radius, num_points):
angles = np.linspace(0, 2 * np.pi, num_points)
points = [(radius * np.cos(angle), radius * np.sin(angle)) for angle in angles]
return points
circle_points = points_on_circle(5, 100)
print(circle_points)
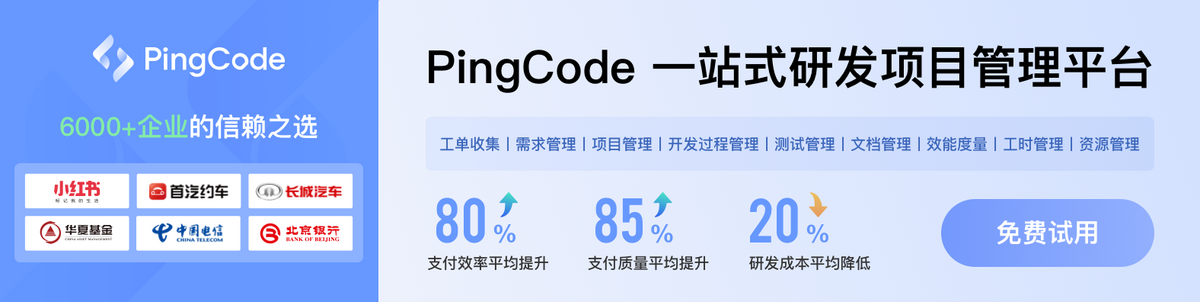