Python采集数据功能如何处理:使用requests库发送HTTP请求、使用BeautifulSoup或lxml解析HTML、使用pandas处理数据、将数据存储到本地或数据库。例如,可以通过requests库发送HTTP请求,从网页获取内容,然后使用BeautifulSoup或lxml库解析HTML内容,提取所需的数据。之后,可以使用pandas库对数据进行处理,并将处理后的数据存储到本地文件或数据库中。以下是详细描述如何使用requests库发送HTTP请求并获取网页内容的方法。
在Python中,requests库是一个强大且易于使用的库,用于发送HTTP请求并处理响应。使用requests库,我们可以轻松地发送GET、POST等请求,并获取响应内容。以下是一个简单的示例,展示如何使用requests库发送GET请求并获取网页内容:
import requests
url = 'https://example.com'
response = requests.get(url)
if response.status_code == 200:
content = response.text
print(content)
else:
print(f'Failed to retrieve content. Status code: {response.status_code}')
在这个示例中,我们首先导入requests库,然后定义要访问的URL。接着,我们使用requests.get()
方法发送GET请求,并将响应存储在response
变量中。通过检查响应的状态码,我们可以确定请求是否成功。如果状态码为200,表示请求成功,我们可以访问response.text
属性获取网页内容。
一、使用requests库发送HTTP请求
requests库是Python中用于发送HTTP请求的首选库,它具有简单易用的API,适用于各种请求类型(如GET、POST、PUT、DELETE等)。requests库还支持会话、重试、代理等高级功能,使其成为采集数据的理想选择。
1、发送GET请求
GET请求是最常见的HTTP请求类型,用于从服务器获取数据。使用requests库发送GET请求非常简单,只需调用requests.get()
方法并传递URL即可。
import requests
url = 'https://example.com'
response = requests.get(url)
if response.status_code == 200:
content = response.text
print(content)
else:
print(f'Failed to retrieve content. Status code: {response.status_code}')
在上面的示例中,我们首先导入requests库,然后定义要访问的URL。接着,我们使用requests.get()
方法发送GET请求,并将响应存储在response
变量中。通过检查响应的状态码,我们可以确定请求是否成功。如果状态码为200,表示请求成功,我们可以访问response.text
属性获取网页内容。
2、发送POST请求
POST请求通常用于向服务器提交数据。使用requests库发送POST请求,只需调用requests.post()
方法并传递URL和数据。
import requests
url = 'https://example.com/login'
data = {'username': 'user', 'password': 'pass'}
response = requests.post(url, data=data)
if response.status_code == 200:
content = response.text
print(content)
else:
print(f'Failed to retrieve content. Status code: {response.status_code}')
在上面的示例中,我们定义了要访问的URL和提交的数据字典。然后,我们使用requests.post()
方法发送POST请求,并将响应存储在response
变量中。通过检查响应的状态码,我们可以确定请求是否成功。
二、使用BeautifulSoup解析HTML
BeautifulSoup是一个用于解析HTML和XML文档的Python库。它提供了一些简单易用的方法,可以轻松地从网页中提取数据。以下是如何使用BeautifulSoup解析HTML的示例。
1、安装BeautifulSoup
首先,我们需要安装BeautifulSoup库及其依赖的解析器库lxml。
pip install beautifulsoup4 lxml
2、解析HTML内容
使用requests库获取网页内容后,我们可以使用BeautifulSoup解析HTML并提取所需的数据。
import requests
from bs4 import BeautifulSoup
url = 'https://example.com'
response = requests.get(url)
if response.status_code == 200:
soup = BeautifulSoup(response.text, 'lxml')
title = soup.title.string
print(f'Title: {title}')
else:
print(f'Failed to retrieve content. Status code: {response.status_code}')
在上面的示例中,我们首先使用requests库获取网页内容。然后,我们使用BeautifulSoup解析HTML,并通过soup.title.string
属性获取网页的标题。
3、提取数据
BeautifulSoup提供了多种方法来查找和提取HTML元素。常用的方法包括find()
、find_all()
、select()
等。
import requests
from bs4 import BeautifulSoup
url = 'https://example.com'
response = requests.get(url)
if response.status_code == 200:
soup = BeautifulSoup(response.text, 'lxml')
articles = soup.find_all('article')
for article in articles:
title = article.find('h2').string
content = article.find('p').text
print(f'Title: {title}')
print(f'Content: {content}')
else:
print(f'Failed to retrieve content. Status code: {response.status_code}')
在上面的示例中,我们使用find_all()
方法查找所有article
元素。然后,我们遍历这些元素,并使用find()
方法查找每个文章的标题和内容。
三、使用pandas处理数据
pandas是一个强大的数据处理和分析库,广泛用于数据科学和机器学习领域。使用pandas,我们可以轻松地对采集到的数据进行处理和分析。
1、安装pandas
首先,我们需要安装pandas库。
pip install pandas
2、将数据转换为DataFrame
在采集到数据后,我们可以使用pandas将数据转换为DataFrame,以便于进一步处理和分析。
import requests
from bs4 import BeautifulSoup
import pandas as pd
url = 'https://example.com'
response = requests.get(url)
if response.status_code == 200:
soup = BeautifulSoup(response.text, 'lxml')
articles = soup.find_all('article')
data = []
for article in articles:
title = article.find('h2').string
content = article.find('p').text
data.append({'Title': title, 'Content': content})
df = pd.DataFrame(data)
print(df)
else:
print(f'Failed to retrieve content. Status code: {response.status_code}')
在上面的示例中,我们将采集到的数据存储在列表中。然后,我们使用pd.DataFrame()
方法将数据转换为DataFrame。
3、数据清洗和处理
pandas提供了丰富的数据处理功能,可以轻松地对数据进行清洗和处理。例如,我们可以删除缺失值、过滤数据、进行数据转换等。
import requests
from bs4 import BeautifulSoup
import pandas as pd
url = 'https://example.com'
response = requests.get(url)
if response.status_code == 200:
soup = BeautifulSoup(response.text, 'lxml')
articles = soup.find_all('article')
data = []
for article in articles:
title = article.find('h2').string
content = article.find('p').text
data.append({'Title': title, 'Content': content})
df = pd.DataFrame(data)
# 数据清洗
df.dropna(inplace=True)
df['Content'] = df['Content'].str.strip()
print(df)
else:
print(f'Failed to retrieve content. Status code: {response.status_code}')
在上面的示例中,我们使用dropna()
方法删除包含缺失值的行,并使用str.strip()
方法去除内容中的空白字符。
四、将数据存储到本地或数据库
采集到的数据处理完毕后,我们可以将其存储到本地文件或数据库中,以便后续使用。
1、将数据存储到本地文件
pandas提供了多种方法来将DataFrame存储到本地文件,如CSV、Excel等。
import requests
from bs4 import BeautifulSoup
import pandas as pd
url = 'https://example.com'
response = requests.get(url)
if response.status_code == 200:
soup = BeautifulSoup(response.text, 'lxml')
articles = soup.find_all('article')
data = []
for article in articles:
title = article.find('h2').string
content = article.find('p').text
data.append({'Title': title, 'Content': content})
df = pd.DataFrame(data)
# 数据清洗
df.dropna(inplace=True)
df['Content'] = df['Content'].str.strip()
# 存储到本地文件
df.to_csv('articles.csv', index=False)
print('Data saved to articles.csv')
else:
print(f'Failed to retrieve content. Status code: {response.status_code}')
在上面的示例中,我们使用to_csv()
方法将DataFrame存储到CSV文件中。
2、将数据存储到数据库
我们还可以使用pandas和SQLAlchemy将数据存储到数据库中。
import requests
from bs4 import BeautifulSoup
import pandas as pd
from sqlalchemy import create_engine
url = 'https://example.com'
response = requests.get(url)
if response.status_code == 200:
soup = BeautifulSoup(response.text, 'lxml')
articles = soup.find_all('article')
data = []
for article in articles:
title = article.find('h2').string
content = article.find('p').text
data.append({'Title': title, 'Content': content})
df = pd.DataFrame(data)
# 数据清洗
df.dropna(inplace=True)
df['Content'] = df['Content'].str.strip()
# 存储到数据库
engine = create_engine('sqlite:///articles.db')
df.to_sql('articles', con=engine, index=False, if_exists='replace')
print('Data saved to articles.db')
else:
print(f'Failed to retrieve content. Status code: {response.status_code}')
在上面的示例中,我们使用SQLAlchemy创建数据库连接,并使用to_sql()
方法将DataFrame存储到SQLite数据库中。
五、使用Selenium处理动态网页
有时,网页内容是通过JavaScript动态加载的,使用requests库无法直接获取这些内容。在这种情况下,我们可以使用Selenium模拟浏览器操作,以获取动态加载的内容。
1、安装Selenium
首先,我们需要安装Selenium库及其依赖的浏览器驱动程序(如ChromeDriver)。
pip install selenium
下载ChromeDriver并将其添加到系统路径。
2、使用Selenium获取动态内容
以下是一个使用Selenium获取动态网页内容的示例。
from selenium import webdriver
from selenium.webdriver.common.by import By
from selenium.webdriver.chrome.service import Service
from selenium.webdriver.chrome.options import Options
from webdriver_manager.chrome import ChromeDriverManager
import pandas as pd
配置Chrome选项
chrome_options = Options()
chrome_options.add_argument('--headless') # 无头模式
chrome_options.add_argument('--disable-gpu')
chrome_options.add_argument('--no-sandbox')
初始化Chrome浏览器
driver = webdriver.Chrome(service=Service(ChromeDriverManager().install()), options=chrome_options)
url = 'https://example.com'
driver.get(url)
等待页面加载完成
driver.implicitly_wait(10)
获取动态加载的内容
articles = driver.find_elements(By.TAG_NAME, 'article')
data = []
for article in articles:
title = article.find_element(By.TAG_NAME, 'h2').text
content = article.find_element(By.TAG_NAME, 'p').text
data.append({'Title': title, 'Content': content})
关闭浏览器
driver.quit()
转换为DataFrame并存储到本地文件
df = pd.DataFrame(data)
df.to_csv('articles_dynamic.csv', index=False)
print('Data saved to articles_dynamic.csv')
在上面的示例中,我们使用Selenium模拟浏览器操作,访问动态网页并获取内容。然后,我们将数据转换为DataFrame并存储到CSV文件中。
六、处理反爬虫机制
在采集数据时,可能会遇到网站的反爬虫机制,如IP封禁、验证码等。为了绕过这些机制,我们可以采取以下措施。
1、使用代理
使用代理可以隐藏我们的真实IP地址,从而避免被封禁。我们可以通过requests库的proxies参数配置代理。
import requests
url = 'https://example.com'
proxies = {
'http': 'http://proxy.example.com:8080',
'https': 'https://proxy.example.com:8080',
}
response = requests.get(url, proxies=proxies)
if response.status_code == 200:
content = response.text
print(content)
else:
print(f'Failed to retrieve content. Status code: {response.status_code}')
在上面的示例中,我们通过proxies参数配置HTTP和HTTPS代理。
2、设置请求头
通过设置请求头,我们可以模拟正常的浏览器请求,避免被反爬虫机制识别。
import requests
url = 'https://example.com'
headers = {
'User-Agent': 'Mozilla/5.0 (Windows NT 10.0; Win64; x64) AppleWebKit/537.36 (KHTML, like Gecko) Chrome/91.0.4472.124 Safari/537.36',
'Referer': 'https://www.google.com',
}
response = requests.get(url, headers=headers)
if response.status_code == 200:
content = response.text
print(content)
else:
print(f'Failed to retrieve content. Status code: {response.status_code}')
在上面的示例中,我们通过headers参数设置User-Agent和Referer等请求头。
3、处理验证码
对于包含验证码的网页,我们可以使用Selenium手动输入验证码,或者使用OCR技术自动识别验证码。
from selenium import webdriver
from selenium.webdriver.common.by import By
from selenium.webdriver.chrome.service import Service
from selenium.webdriver.chrome.options import Options
from webdriver_manager.chrome import ChromeDriverManager
配置Chrome选项
chrome_options = Options()
chrome_options.add_argument('--headless') # 无头模式
chrome_options.add_argument('--disable-gpu')
chrome_options.add_argument('--no-sandbox')
初始化Chrome浏览器
driver = webdriver.Chrome(service=Service(ChromeDriverManager().install()), options=chrome_options)
url = 'https://example.com'
driver.get(url)
等待页面加载完成
driver.implicitly_wait(10)
手动输入验证码
captcha_input = driver.find_element(By.ID, 'captcha')
captcha_input.send_keys('manual_captcha_solution')
提交表单
submit_button = driver.find_element(By.ID, 'submit')
submit_button.click()
获取表单提交后的内容
content = driver.page_source
关闭浏览器
driver.quit()
print(content)
在上面的示例中,我们使用Selenium手动输入验证码并提交表单,以获取表单提交后的内容。
七、定时采集和增量更新
在实际应用中,我们可能需要定时采集数据,并进行增量更新。可以使用Python的定时任务库sched或APScheduler来实现定时任务。
1、使用sched库实现定时任务
import sched
import time
import requests
创建调度器
scheduler = sched.scheduler(time.time, time.sleep)
def fetch_data():
url = 'https://example.com'
response = requests.get(url)
if response.status_code == 200:
content = response.text
print(content)
else:
print(f'Failed to retrieve content. Status code: {response.status_code}')
# 重新调度任务
scheduler.enter(3600, 1, fetch_data)
调度第一次任务
scheduler.enter(0, 1, fetch_data)
运行调度器
scheduler.run()
在上面的示例中,我们使用sched库创建调度器,并调度一个每小时运行一次的任务。
2、使用APScheduler实现定时任务
from apscheduler.schedulers.blocking import BlockingScheduler
import requests
def fetch_data():
url = 'https://example.com'
response = requests.get(url)
if response.status_code == 200:
content = response.text
print(content)
else:
print(f'Failed to retrieve content. Status code: {response.status_code}')
创建调度器
scheduler = BlockingScheduler()
调度任务
scheduler.add_job(fetch_data, 'interval', hours=1)
运行调度器
scheduler.start()
在上面的示例中,我们使用APScheduler创建调度器,并调度一个每小时运行一次的任务。
3、实现增量更新
为了实现增量更新,我们可以在采集数据时记录上次采集的时间戳,并在下次采集时只获取新的数据。
import requests
import pandas as pd
from datetime import datetime
记录上次采集时间
last_fetch_time = None
相关问答FAQs:
如何使用Python进行数据采集?
Python提供了多种库和工具来进行数据采集,如Requests、Beautiful Soup、Scrapy等。使用Requests库可以方便地发送HTTP请求并获取网页内容,而Beautiful Soup则可以帮助解析HTML或XML文档,从中提取所需的数据。对于需要处理大量数据的网站,Scrapy框架是一个非常强大且高效的选择,支持异步处理和数据存储。
在Python中如何处理采集到的数据?
采集到的数据通常需要经过清洗和整理才能使用。可以使用Pandas库来处理数据,这个库提供了强大的数据结构和数据分析工具。通过Pandas,可以轻松地对数据进行去重、填充缺失值、转换数据类型等操作。此外,数据还可以导出为CSV、Excel等格式,方便后续分析和使用。
如何避免数据采集中的法律和伦理问题?
在进行数据采集时,遵守法律法规和网站的使用条款是至关重要的。确保在采集前检查网站的robots.txt文件,了解哪些内容是被允许的,哪些是不被允许的。在进行采集操作时,可以设置合理的请求频率,避免对目标网站造成负担,并尊重用户隐私,不采集敏感信息。
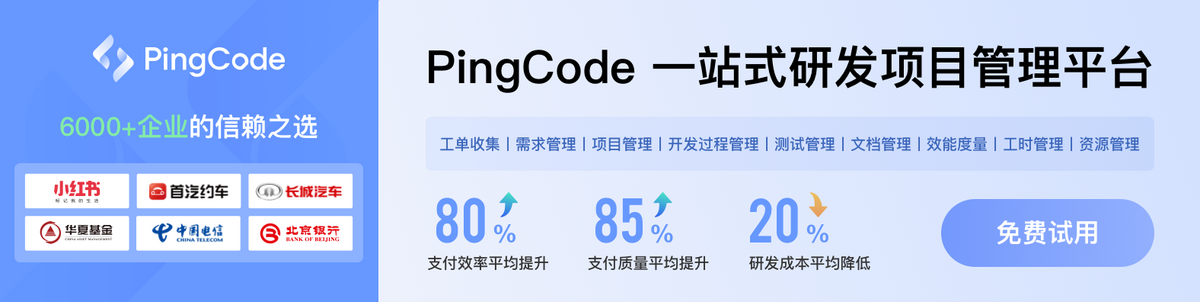