Python通过图片链接打开图片可以通过多种方式实现,常见的方法包括使用PIL(Python Imaging Library)、OpenCV、以及直接用requests库下载后再打开。其中,使用PIL库结合requests库是比较简单且常用的方法。下面将详细描述如何通过这几种方法实现打开图片。
使用PIL和requests库
PIL是Python Imaging Library的缩写,这是一个强大的图像处理库。虽然PIL已经停止更新,但是Pillow库是它的一个分支,并且得到了积极的维护和更新。requests库则是一个简单易用的HTTP库,用于发送HTTP请求。
- 安装Pillow和requests库:
pip install pillow requests
- 使用Pillow和requests库打开图片:
from PIL import Image
import requests
from io import BytesIO
def open_image_from_url(url):
response = requests.get(url)
img = Image.open(BytesIO(response.content))
img.show()
示例
image_url = "https://example.com/path/to/image.jpg"
open_image_from_url(image_url)
在上面的代码中,我们首先使用requests库发送HTTP GET请求获取图片的内容,然后使用BytesIO将图片内容转换为一个字节流,最后使用Pillow库的Image.open方法打开图片并显示。
使用OpenCV库
OpenCV(Open Source Computer Vision Library)是一个开源的计算机视觉和图像处理库。OpenCV提供了丰富的图像处理功能,并且可以通过cv2模块在Python中使用。
- 安装OpenCV和requests库:
pip install opencv-python requests
- 使用OpenCV和requests库打开图片:
import cv2
import requests
import numpy as np
def open_image_from_url(url):
response = requests.get(url)
img_array = np.array(bytearray(response.content), dtype=np.uint8)
img = cv2.imdecode(img_array, -1)
cv2.imshow('Image', img)
cv2.waitKey(0)
cv2.destroyAllWindows()
示例
image_url = "https://example.com/path/to/image.jpg"
open_image_from_url(image_url)
在上面的代码中,我们使用requests库获取图片的内容,并将其转换为一个字节数组,接着使用numpy库将字节数组转换为一个Numpy数组,然后使用OpenCV的imdecode方法将Numpy数组解码为图像,并显示图像。
直接下载图片后再打开
有时候,我们可能需要先下载图片到本地,然后再打开。这种方法也可以通过requests库实现。
- 安装requests库:
pip install requests
- 下载图片并打开:
import requests
from PIL import Image
import os
def download_image(url, filename):
response = requests.get(url)
with open(filename, 'wb') as f:
f.write(response.content)
def open_image(filename):
img = Image.open(filename)
img.show()
示例
image_url = "https://example.com/path/to/image.jpg"
image_filename = "downloaded_image.jpg"
download_image(image_url, image_filename)
open_image(image_filename)
删除临时下载的图片
os.remove(image_filename)
在上面的代码中,我们首先使用requests库下载图片并保存到本地文件,然后使用Pillow库打开并显示图片,最后删除临时下载的图片文件。
总结
通过以上几种方法,我们可以轻松地在Python中通过图片链接打开图片。使用PIL(Pillow)和requests库、OpenCV库、以及直接下载图片后再打开,都是有效的方法。根据具体需求选择合适的方法可以提高代码的可维护性和效率。在实际应用中,可以根据项目的具体需求选择最适合的方法。
相关问答FAQs:
如何在Python中通过图片链接下载并打开图片?
在Python中,可以使用requests
库来下载图片,然后使用PIL
库(Pillow)打开图片。以下是一个简单的示例代码:
import requests
from PIL import Image
from io import BytesIO
url = '图片链接'
response = requests.get(url)
img = Image.open(BytesIO(response.content))
img.show()
确保在使用之前安装了requests
和Pillow
库,可以通过pip install requests Pillow
进行安装。
是否可以使用其他库来实现相同功能?
除了requests
和PIL
,你还可以使用opencv
库来打开图片。opencv
提供了强大的图像处理功能。示例代码如下:
import cv2
import numpy as np
import requests
url = '图片链接'
response = requests.get(url)
img_array = np.asarray(bytearray(response.content), dtype=np.uint8)
img = cv2.imdecode(img_array, cv2.IMREAD_COLOR)
cv2.imshow('Image', img)
cv2.waitKey(0)
cv2.destroyAllWindows()
使用opencv
时,请确保安装了相关库,命令为pip install opencv-python
。
在使用图片链接时需要注意什么?
在使用图片链接时,请确保链接是有效的并且指向的文件确实是图片。某些网站可能会限制直接访问图片,导致下载失败。此外,处理大图像文件时要考虑内存使用情况,适当进行优化和处理。确保遵循相关网站的使用条款,避免未经授权的下载。
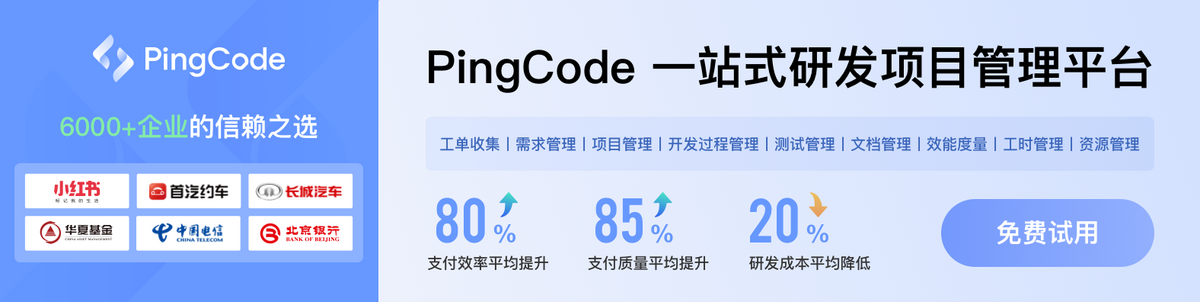