在Python中设置循环输入密码的方法主要有几种:使用while循环、使用for循环。以下是详细描述:
一、使用while循环
使用while循环可以让用户反复输入密码,直到输入正确为止。这种方式非常灵活,可以在任何地方中断循环。首先,我们设定一个正确的密码,然后开始一个while循环,直到用户输入正确的密码才终止循环。举个例子:
correct_password = "password123"
while True:
password = input("Enter your password: ")
if password == correct_password:
print("Password accepted.")
break
else:
print("Incorrect password. Try again.")
在这个例子中,用户会一直被要求输入密码,直到他们输入的密码与预设的密码匹配为止。
二、限制输入次数
有时候,我们可能不想让用户无限次地尝试输入密码。我们可以使用一个计数器来限制输入次数,例如限制用户最多输入3次密码。我们可以使用for循环来实现这一点:
correct_password = "password123"
max_attempts = 3
for attempt in range(max_attempts):
password = input("Enter your password: ")
if password == correct_password:
print("Password accepted.")
break
else:
print(f"Incorrect password. You have {max_attempts - attempt - 1} attempts left.")
else:
print("Maximum attempts reached. Access denied.")
在这个例子中,用户最多可以尝试3次。如果他们在3次尝试内没有输入正确的密码,程序会终止并显示“Maximum attempts reached. Access denied.”的消息。
三、隐藏输入的密码
在实际应用中,通常不希望用户输入的密码显示在屏幕上。我们可以使用getpass模块来隐藏输入的密码。getpass模块提供了一个getpass()函数,可以在用户输入密码时隐藏输入的字符。以下是示例代码:
import getpass
correct_password = "password123"
max_attempts = 3
for attempt in range(max_attempts):
password = getpass.getpass("Enter your password: ")
if password == correct_password:
print("Password accepted.")
break
else:
print(f"Incorrect password. You have {max_attempts - attempt - 1} attempts left.")
else:
print("Maximum attempts reached. Access denied.")
在这个例子中,用户输入的密码将不会显示在屏幕上,从而提高了安全性。
四、使用函数进行密码验证
将密码验证逻辑封装在一个函数中,可以使代码更加模块化和易于维护。以下是示例代码:
import getpass
def verify_password(correct_password, max_attempts=3):
for attempt in range(max_attempts):
password = getpass.getpass("Enter your password: ")
if password == correct_password:
print("Password accepted.")
return True
else:
print(f"Incorrect password. You have {max_attempts - attempt - 1} attempts left.")
print("Maximum attempts reached. Access denied.")
return False
使用示例
correct_password = "password123"
verify_password(correct_password)
通过将密码验证逻辑封装在verify_password函数中,我们可以在需要进行密码验证的任何地方调用这个函数。
五、增加安全性
为了进一步提高密码验证的安全性,可以考虑以下几点:
-
使用哈希函数存储密码
直接存储明文密码是不安全的。可以使用哈希函数(例如SHA-256)将密码哈希后存储。这样,即使数据库被泄露,攻击者也无法直接获取明文密码。 -
增加盐值
为了防止彩虹表攻击,可以在哈希密码之前增加一个随机的盐值。盐值是一个随机生成的字符串,它会与密码一起使用来生成哈希值。 -
使用多因素认证
除了输入密码,还可以要求用户提供其他验证信息,例如短信验证码、电子邮件验证码等。
以下是一个示例代码,展示了如何使用哈希函数和盐值来存储和验证密码:
import hashlib
import os
def hash_password(password, salt):
return hashlib.sha256(salt + password.encode()).hexdigest()
def verify_password(stored_password, salt, input_password):
return stored_password == hash_password(input_password, salt)
示例
password = "password123"
salt = os.urandom(16)
hashed_password = hash_password(password, salt)
max_attempts = 3
for attempt in range(max_attempts):
input_password = input("Enter your password: ")
if verify_password(hashed_password, salt, input_password):
print("Password accepted.")
break
else:
print(f"Incorrect password. You have {max_attempts - attempt - 1} attempts left.")
else:
print("Maximum attempts reached. Access denied.")
在这个例子中,我们使用了SHA-256哈希函数,并为每个密码生成一个随机的盐值。这样,即使两个用户的密码相同,它们的哈希值也会不同,从而提高了安全性。
总结:在Python中设置循环输入密码的方法有多种,包括使用while循环、限制输入次数、隐藏输入的密码、使用函数进行密码验证等。为了提高安全性,还可以使用哈希函数存储密码、增加盐值以及使用多因素认证。通过合理的设计和实现,可以确保用户的密码输入过程既方便又安全。
相关问答FAQs:
如何在Python中实现密码的循环输入?
在Python中,可以使用while
循环来实现密码的循环输入。你可以设置一个条件,只有当用户输入正确的密码时,循环才会结束。示例代码如下:
correct_password = "your_password"
while True:
user_input = input("请输入密码: ")
if user_input == correct_password:
print("密码正确!")
break
else:
print("密码错误,请重试。")
如何限制用户输入密码的尝试次数?
可以通过引入一个计数器来限制用户输入密码的尝试次数。例如,用户只能尝试三次,代码示例如下:
correct_password = "your_password"
attempts = 0
max_attempts = 3
while attempts < max_attempts:
user_input = input("请输入密码: ")
if user_input == correct_password:
print("密码正确!")
break
else:
attempts += 1
print(f"密码错误,请重试。剩余尝试次数: {max_attempts - attempts}")
if attempts == max_attempts:
print("达到最大尝试次数,程序结束。")
如何在密码输入时隐藏用户输入的字符?
在Python中,可以使用getpass
模块来隐藏用户输入的密码字符。这样可以提高安全性,代码示例如下:
import getpass
correct_password = "your_password"
while True:
user_input = getpass.getpass("请输入密码: ")
if user_input == correct_password:
print("密码正确!")
break
else:
print("密码错误,请重试。")
通过这些示例,可以灵活实现循环输入密码的功能,增强程序的交互性和安全性。
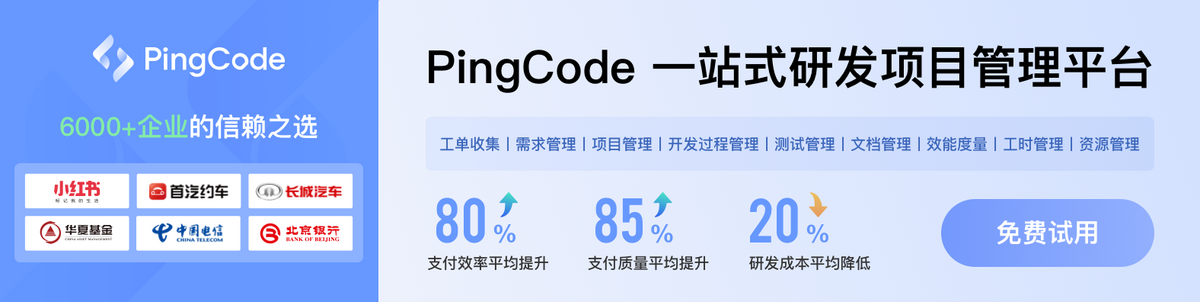