使用Python抓取店铺的关键字,可以通过以下几种方式:使用requests库发送HTTP请求、使用BeautifulSoup解析HTML、利用Scrapy框架进行数据爬取、结合Selenium进行动态页面处理。 其中,requests 和 BeautifulSoup 是最基础的方法,适用于静态页面;Scrapy 是一个功能强大的爬虫框架,适用于大规模爬取;Selenium 则适用于处理需要JavaScript渲染的动态页面。下面将详细介绍如何使用这些方法来抓取店铺的关键字。
一、请求与解析
要使用Python抓取店铺的关键字,首先需要发送HTTP请求获取网页内容,然后解析HTML以提取需要的信息。最常用的库是requests和BeautifulSoup。
1. 使用requests库发送HTTP请求
requests库用于发送HTTP请求,获取网页内容。首先,安装requests库:
pip install requests
使用示例:
import requests
url = 'https://example.com/shop'
response = requests.get(url)
html_content = response.text
在上述代码中,我们发送了一个GET请求并获取了网页的HTML内容。
2. 使用BeautifulSoup解析HTML
BeautifulSoup用于解析HTML文档并提取数据。首先,安装BeautifulSoup:
pip install beautifulsoup4
使用示例:
from bs4 import BeautifulSoup
soup = BeautifulSoup(html_content, 'html.parser')
keywords = soup.find_all('meta', attrs={'name': 'keywords'})
for keyword in keywords:
print(keyword['content'])
在上述代码中,我们使用BeautifulSoup解析HTML内容,并查找所有meta标签中name属性为keywords的内容。
二、使用Scrapy框架
Scrapy是一个强大的爬虫框架,适用于大规模数据爬取。首先,安装Scrapy:
pip install scrapy
1. 创建Scrapy项目
scrapy startproject shopkeywords
cd shopkeywords
2. 创建爬虫
scrapy genspider shop_spider example.com
3. 编写爬虫
在shop_spider.py
中:
import scrapy
class ShopSpider(scrapy.Spider):
name = 'shop_spider'
start_urls = ['https://example.com/shop']
def parse(self, response):
keywords = response.xpath('//meta[@name="keywords"]/@content').getall()
for keyword in keywords:
yield {'keyword': keyword}
4. 运行爬虫
scrapy crawl shop_spider -o keywords.json
在上述代码中,我们定义了一个Scrapy爬虫,发送请求并解析响应,提取关键字并保存到JSON文件中。
三、结合Selenium进行动态页面处理
Selenium用于处理需要JavaScript渲染的动态页面。首先,安装Selenium:
pip install selenium
1. 安装WebDriver
下载适用于你浏览器的WebDriver并将其路径添加到系统PATH中。例如,使用Chrome浏览器,下载ChromeDriver。
2. 使用Selenium抓取关键字
from selenium import webdriver
from bs4 import BeautifulSoup
driver = webdriver.Chrome()
driver.get('https://example.com/shop')
html_content = driver.page_source
driver.quit()
soup = BeautifulSoup(html_content, 'html.parser')
keywords = soup.find_all('meta', attrs={'name': 'keywords'})
for keyword in keywords:
print(keyword['content'])
在上述代码中,我们使用Selenium打开网页,获取渲染后的HTML内容,并使用BeautifulSoup解析HTML。
四、综合实例
结合上述方法,创建一个综合实例,通过命令行参数输入店铺URL,抓取并输出关键字。
import argparse
import requests
from bs4 import BeautifulSoup
from selenium import webdriver
def fetch_keywords(url, use_selenium=False):
if use_selenium:
driver = webdriver.Chrome()
driver.get(url)
html_content = driver.page_source
driver.quit()
else:
response = requests.get(url)
html_content = response.text
soup = BeautifulSoup(html_content, 'html.parser')
keywords = soup.find_all('meta', attrs={'name': 'keywords'})
return [keyword['content'] for keyword in keywords]
if __name__ == "__main__":
parser = argparse.ArgumentParser(description='Fetch shop keywords.')
parser.add_argument('url', type=str, help='The URL of the shop')
parser.add_argument('--selenium', action='store_true', help='Use Selenium for dynamic pages')
args = parser.parse_args()
keywords = fetch_keywords(args.url, args.selenium)
for keyword in keywords:
print(keyword)
运行示例:
python fetch_keywords.py https://example.com/shop --selenium
通过上述综合实例,我们可以方便地抓取指定店铺的关键字,无论页面是静态还是动态。
总结
抓取店铺的关键字是一个常见的数据爬取任务。使用Python,我们可以通过requests和BeautifulSoup来处理静态页面,通过Scrapy框架来进行大规模数据爬取,通过Selenium来处理动态页面。根据具体需求选择合适的方法,能够高效地完成抓取任务。
相关问答FAQs:
如何使用Python抓取店铺的关键字?
要抓取店铺的关键字,可以使用Python中的爬虫库,如Beautiful Soup和Requests。首先,您需要确定要抓取的网站,并分析其HTML结构,以便能够定位到关键字所在的元素。接着,使用Requests库获取网页内容,利用Beautiful Soup解析HTML,并提取所需的关键字信息。完成后,可以将结果存储到文件或数据库中,以便后续分析。
抓取店铺关键字时需要注意哪些法律和道德问题?
在进行网页抓取时,务必遵循网站的robots.txt文件中的规定,确保您抓取的内容不违反网站的使用条款。此外,尊重数据隐私和版权,避免抓取敏感或受保护的信息。合理使用抓取的内容,避免造成对网站的负面影响,比如频繁请求导致服务器过载。
有什么推荐的Python库可以帮助抓取店铺的关键字?
在Python中,有多个库可以帮助您进行网页抓取。常用的包括Requests(用于发送HTTP请求)、Beautiful Soup(用于解析HTML和XML文档)、Scrapy(一个强大的爬虫框架),以及Pandas(用于数据处理和分析)。选择合适的库可以大大简化抓取过程,并提高数据处理的效率。
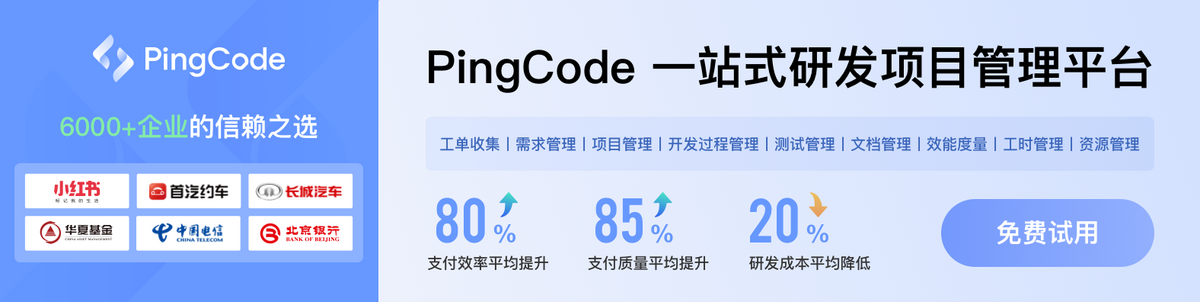