Python如何输入一个空间坐标
在Python中输入一个空间坐标可以通过多种方式实现,最常见的方法包括使用内置的input函数、通过文件输入、使用图形界面库如Tkinter、或者通过外部库如Pandas来读取数据。这些方法各有优缺点,选择合适的方法取决于具体的应用场景和需求。下面我们将详细介绍其中的一种方法——使用内置的input函数,并扩展到其他方法的使用。
一、使用内置的input函数
Python的内置input函数是最简单和直接的方法之一。通过input函数,用户可以直接在控制台输入坐标。以下是一个简单的示例代码:
def get_coordinates():
x = float(input("Enter the X coordinate: "))
y = float(input("Enter the Y coordinate: "))
z = float(input("Enter the Z coordinate: "))
return (x, y, z)
coordinates = get_coordinates()
print("The coordinates you entered are:", coordinates)
在这个示例中,程序首先提示用户输入X、Y和Z坐标,然后将这些值存储在一个元组中并输出。
二、通过文件输入
在实际应用中,坐标数据可能存储在文件中,通过读取文件来获取坐标也是常见的方法之一。可以使用Python的内置open函数来读取文件,或使用更高级的库如Pandas进行数据处理。
1、使用内置的open函数
下面是一个使用open函数读取坐标数据的示例:
def read_coordinates_from_file(file_path):
with open(file_path, 'r') as file:
lines = file.readlines()
coordinates = []
for line in lines:
x, y, z = map(float, line.strip().split(','))
coordinates.append((x, y, z))
return coordinates
file_path = 'coordinates.txt'
coordinates = read_coordinates_from_file(file_path)
print("The coordinates from the file are:", coordinates)
在这个示例中,程序读取一个名为coordinates.txt的文件,假设文件中的每一行包含一个坐标,坐标之间用逗号分隔,程序将这些坐标存储在一个列表中并输出。
2、使用Pandas库
Pandas是一个强大的数据处理库,特别适合处理结构化数据。使用Pandas读取坐标文件更为简便。以下是一个示例:
import pandas as pd
def read_coordinates_with_pandas(file_path):
df = pd.read_csv(file_path)
coordinates = df.to_records(index=False)
return list(coordinates)
file_path = 'coordinates.csv'
coordinates = read_coordinates_with_pandas(file_path)
print("The coordinates from the CSV file are:", coordinates)
在这个示例中,程序使用Pandas读取一个CSV文件,假设文件的每一行是一个坐标,程序将这些坐标转换为一个列表并输出。
三、使用图形界面库如Tkinter
对于需要用户友好界面的应用,可以使用Tkinter创建一个简单的图形界面来输入坐标。以下是一个示例:
import tkinter as tk
from tkinter import simpledialog
def get_coordinates_with_tkinter():
root = tk.Tk()
root.withdraw() # Hide the root window
x = float(simpledialog.askstring("Input", "Enter the X coordinate:"))
y = float(simpledialog.askstring("Input", "Enter the Y coordinate:"))
z = float(simpledialog.askstring("Input", "Enter the Z coordinate:"))
return (x, y, z)
coordinates = get_coordinates_with_tkinter()
print("The coordinates you entered are:", coordinates)
在这个示例中,程序使用Tkinter库创建一个简单的对话框,提示用户输入X、Y和Z坐标,然后将这些值存储在一个元组中并输出。
四、通过外部库读取数据
在处理复杂数据时,可能需要使用外部库来读取和处理数据。Pandas库是一个非常流行的数据处理库,非常适合处理表格数据。以下是一个使用Pandas读取CSV文件的示例:
import pandas as pd
def read_coordinates_with_pandas(file_path):
df = pd.read_csv(file_path)
coordinates = df.to_records(index=False)
return list(coordinates)
file_path = 'coordinates.csv'
coordinates = read_coordinates_with_pandas(file_path)
print("The coordinates from the CSV file are:", coordinates)
在这个示例中,程序使用Pandas读取一个CSV文件,假设文件的每一行是一个坐标,程序将这些坐标转换为一个列表并输出。
五、使用三维坐标系统
在一些高级应用中,如科学计算或图形处理,可能需要使用三维坐标系统。Python提供了许多库来处理三维坐标系统,如NumPy和matplotlib。以下是一个使用NumPy处理三维坐标的示例:
import numpy as np
def create_3d_coordinate_array():
coordinates = np.array([
[1.0, 2.0, 3.0],
[4.0, 5.0, 6.0],
[7.0, 8.0, 9.0]
])
return coordinates
coordinates = create_3d_coordinate_array()
print("The 3D coordinates array is:")
print(coordinates)
在这个示例中,程序使用NumPy创建一个三维坐标数组,并输出数组的内容。
六、应用实例:三维图形绘制
在实际应用中,三维坐标可以用于绘制三维图形。以下是一个使用matplotlib绘制三维散点图的示例:
import matplotlib.pyplot as plt
from mpl_toolkits.mplot3d import Axes3D
def plot_3d_coordinates(coordinates):
fig = plt.figure()
ax = fig.add_subplot(111, projection='3d')
xs, ys, zs = zip(*coordinates)
ax.scatter(xs, ys, zs)
plt.show()
coordinates = [
(1.0, 2.0, 3.0),
(4.0, 5.0, 6.0),
(7.0, 8.0, 9.0)
]
plot_3d_coordinates(coordinates)
在这个示例中,程序使用matplotlib库绘制一个三维散点图,展示了一组三维坐标。
七、总结
输入空间坐标在Python中有多种实现方法,包括使用内置的input函数、通过文件输入、使用图形界面库如Tkinter、以及通过外部库如Pandas读取数据。选择合适的方法取决于具体的应用场景和需求。无论是简单的控制台输入,还是复杂的数据处理和图形绘制,Python都提供了强大的工具和库来满足不同的需求。
相关问答FAQs:
如何在Python中输入三维空间坐标?
在Python中,可以使用内置的input()
函数来接收用户输入的三维空间坐标。用户可以按照特定格式输入坐标,例如“x,y,z”,然后使用split()
方法将其分割并转换为浮点数。例如:
coordinates = input("请输入三维空间坐标 (格式: x,y,z): ")
x, y, z = map(float, coordinates.split(','))
这样就能将输入的字符串转换为三个浮点数,分别代表x、y和z坐标。
如何验证用户输入的空间坐标格式?
为了确保用户输入的坐标格式正确,可以使用异常处理和正则表达式进行验证。通过捕获ValueError
,可以判断用户输入的格式是否符合要求。同时,正则表达式可以帮助验证输入是否为数字。例如:
import re
def is_valid_coordinate(coordinate):
pattern = r"^-?\d+(\.\d+)?,-?\d+(\.\d+)?,-?\d+(\.\d+)?$"
return re.match(pattern, coordinate) is not None
coordinates = input("请输入三维空间坐标 (格式: x,y,z): ")
if is_valid_coordinate(coordinates):
x, y, z = map(float, coordinates.split(','))
else:
print("输入格式不正确,请按照 x,y,z 的格式输入坐标。")
通过这种方式,可以有效地检查输入的有效性。
在Python中如何将空间坐标存储为对象?
创建一个类来表示空间坐标是一种很好的方法,可以使用类属性来存储x、y、z值。这样可以方便地进行坐标的管理和操作。例如:
class Coordinate:
def __init__(self, x, y, z):
self.x = x
self.y = y
self.z = z
def __repr__(self):
return f"Coordinate({self.x}, {self.y}, {self.z})"
# 输入坐标并创建对象
coordinates = input("请输入三维空间坐标 (格式: x,y,z): ")
x, y, z = map(float, coordinates.split(','))
point = Coordinate(x, y, z)
print(point)
通过这种方式,可以将空间坐标封装在一个对象中,便于后续的扩展和功能实现。
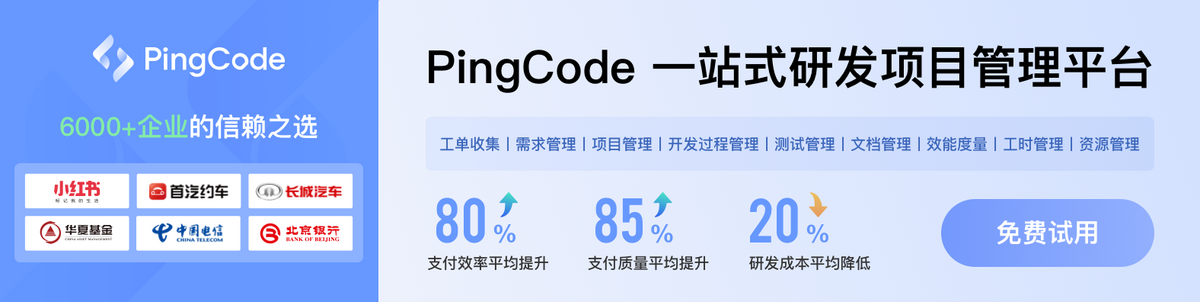