Python3 如何判断网页是否存在
使用 Python3 判断网页是否存在,可以通过发送 HTTP 请求并检查响应状态码、使用 try-except 处理异常、检查响应内容。 其中,通过发送 HTTP 请求并检查响应状态码是最常用的方法之一。接下来,我们将详细描述如何使用这些方法来判断网页是否存在。
一、通过 HTTP 请求检查网页状态
1、使用 requests 库
Python 的 requests
库使得发送 HTTP 请求变得非常简单。我们可以通过发送一个 GET 请求来检查网页是否存在。如果响应状态码为 200,则表示网页存在。常见的状态码如下:
- 200: 请求成功,网页存在。
- 404: 未找到网页,网页不存在。
- 500: 服务器内部错误,可能存在问题,但不能确定网页是否存在。
import requests
def check_website_exists(url):
try:
response = requests.get(url)
# 通过状态码判断网页是否存在
if response.status_code == 200:
return True
else:
return False
except requests.exceptions.RequestException as e:
# 处理请求异常
print(f"An error occurred: {e}")
return False
示例
url = "https://www.example.com"
if check_website_exists(url):
print("Website exists.")
else:
print("Website does not exist.")
2、使用 urllib 库
urllib
是 Python 内置的库,也可以用来发送 HTTP 请求并检查网页是否存在。与 requests
库类似,我们可以通过捕获异常来处理请求失败的情况。
from urllib.request import urlopen
from urllib.error import URLError, HTTPError
def check_website_exists(url):
try:
response = urlopen(url)
if response.status == 200:
return True
else:
return False
except HTTPError as e:
print(f"HTTP error occurred: {e.code}")
return False
except URLError as e:
print(f"URL error occurred: {e.reason}")
return False
示例
url = "https://www.example.com"
if check_website_exists(url):
print("Website exists.")
else:
print("Website does not exist.")
二、处理异常
1、捕获请求异常
在发送 HTTP 请求时,可能会遇到各种异常情况,例如网络连接失败、超时等。因此,捕获异常并进行相应处理是非常重要的。
import requests
def check_website_exists(url):
try:
response = requests.get(url, timeout=10)
if response.status_code == 200:
return True
else:
return False
except requests.exceptions.Timeout:
print("The request timed out")
return False
except requests.exceptions.TooManyRedirects:
print("Too many redirects")
return False
except requests.exceptions.RequestException as e:
print(f"An error occurred: {e}")
return False
示例
url = "https://www.example.com"
if check_website_exists(url):
print("Website exists.")
else:
print("Website does not exist.")
2、处理 HTTP 错误
在捕获 HTTP 错误时,可以根据不同的状态码进行不同的处理。例如,对于 404 错误,可以明确告知用户网页不存在;对于 500 错误,可以提示用户服务器内部错误。
from urllib.request import urlopen
from urllib.error import URLError, HTTPError
def check_website_exists(url):
try:
response = urlopen(url)
if response.status == 200:
return True
else:
return False
except HTTPError as e:
if e.code == 404:
print("Error 404: Not Found")
elif e.code == 500:
print("Error 500: Internal Server Error")
else:
print(f"HTTP error occurred: {e.code}")
return False
except URLError as e:
print(f"URL error occurred: {e.reason}")
return False
示例
url = "https://www.example.com"
if check_website_exists(url):
print("Website exists.")
else:
print("Website does not exist.")
三、检查响应内容
1、检查特定关键字
有时网页可能返回状态码 200,但内容却是一个错误页面。这种情况下,可以通过检查响应内容中的特定关键字来进一步确认网页是否存在。例如,可以检查网页标题是否包含 "404" 或 "Not Found" 等关键词。
import requests
def check_website_exists(url):
try:
response = requests.get(url, timeout=10)
if response.status_code == 200:
# 检查响应内容中的特定关键字
if "404" in response.text or "Not Found" in response.text:
return False
return True
else:
return False
except requests.exceptions.RequestException as e:
print(f"An error occurred: {e}")
return False
示例
url = "https://www.example.com"
if check_website_exists(url):
print("Website exists.")
else:
print("Website does not exist.")
2、检查网页标题
另一种方法是检查网页标题是否匹配预期的内容。可以通过解析 HTML 内容并检查 <title>
标签中的文本来实现这一点。
import requests
from bs4 import BeautifulSoup
def check_website_exists(url):
try:
response = requests.get(url, timeout=10)
if response.status_code == 200:
# 解析 HTML 内容并检查标题
soup = BeautifulSoup(response.text, 'html.parser')
title = soup.find('title').text
if "404" in title or "Not Found" in title:
return False
return True
else:
return False
except requests.exceptions.RequestException as e:
print(f"An error occurred: {e}")
return False
示例
url = "https://www.example.com"
if check_website_exists(url):
print("Website exists.")
else:
print("Website does not exist.")
四、使用头部请求
1、发送 HEAD 请求
发送 HEAD 请求只会获取响应头部,而不会下载整个网页内容。这种方法可以提高效率,特别是在检查大量网页时。
import requests
def check_website_exists(url):
try:
response = requests.head(url, timeout=10)
if response.status_code == 200:
return True
else:
return False
except requests.exceptions.RequestException as e:
print(f"An error occurred: {e}")
return False
示例
url = "https://www.example.com"
if check_website_exists(url):
print("Website exists.")
else:
print("Website does not exist.")
2、处理重定向
在发送 HEAD 请求时,网页可能会进行重定向。在这种情况下,可以选择是否跟随重定向。
import requests
def check_website_exists(url, allow_redirects=True):
try:
response = requests.head(url, allow_redirects=allow_redirects, timeout=10)
if response.status_code == 200:
return True
else:
return False
except requests.exceptions.RequestException as e:
print(f"An error occurred: {e}")
return False
示例
url = "https://www.example.com"
if check_website_exists(url):
print("Website exists.")
else:
print("Website does not exist.")
五、使用第三方服务
1、使用 HTTP 状态码检查服务
有些第三方服务专门提供 HTTP 状态码检查,可以通过这些服务来判断网页是否存在。例如,使用 httpbin.org
提供的服务。
import requests
def check_website_exists(url):
try:
response = requests.get(f"https://httpbin.org/status/200?url={url}", timeout=10)
if response.status_code == 200:
return True
else:
return False
except requests.exceptions.RequestException as e:
print(f"An error occurred: {e}")
return False
示例
url = "https://www.example.com"
if check_website_exists(url):
print("Website exists.")
else:
print("Website does not exist.")
2、使用在线工具
一些在线工具和 API 可以帮助检查网页是否存在,例如 isitdownrightnow.com
或 downforeveryoneorjustme.com
。可以通过调用这些 API 来检查网页状态。
import requests
def check_website_exists(url):
try:
response = requests.get(f"https://isitdownrightnow.com/check.php?domain={url}", timeout=10)
if "is UP" in response.text:
return True
else:
return False
except requests.exceptions.RequestException as e:
print(f"An error occurred: {e}")
return False
示例
url = "https://www.example.com"
if check_website_exists(url):
print("Website exists.")
else:
print("Website does not exist.")
通过以上方法,我们可以使用 Python3 来判断网页是否存在。从发送 HTTP 请求检查状态码、处理异常、检查响应内容、使用头部请求到利用第三方服务,每种方法都有其适用的场景和优缺点。在实际应用中,可以根据具体需求选择合适的方法来判断网页是否存在。
相关问答FAQs:
如何使用Python3检查网页的可用性?
您可以使用Python的requests
库发送HTTP请求来检查网页是否存在。通过捕获HTTP响应状态码,可以判断网页是否可访问。例如,状态码200表示网页存在,而404表示网页不存在。以下是一个简单的代码示例:
import requests
def check_website(url):
try:
response = requests.get(url)
if response.status_code == 200:
return "网页存在"
else:
return f"网页不存在,状态码: {response.status_code}"
except requests.exceptions.RequestException as e:
return f"请求出错: {e}"
url = "https://www.example.com"
print(check_website(url))
使用Python3时,如何处理可能出现的异常?
在进行网页请求时,网络问题可能导致请求失败。因此,在编写代码时,务必要使用异常处理来捕获这些错误。通过try
和except
语句,您可以捕获requests.exceptions.RequestException
,并给出用户友好的错误信息。这可以确保您的程序在遇到问题时不会崩溃,而是优雅地处理错误并返回相应的信息。
可以使用哪些库来判断网页是否存在?
除了requests
库,Python中还有其他一些库可以用来检查网页的可用性,例如urllib
和http.client
。urllib
可以通过urlopen
方法获取网页响应,而http.client
则提供了更底层的HTTP客户端功能。选择合适的库取决于您的具体需求和使用习惯。
以下是使用urllib
的一个简单示例:
from urllib.request import urlopen
from urllib.error import URLError
def check_website(url):
try:
response = urlopen(url)
return "网页存在"
except URLError:
return "网页不存在"
url = "https://www.example.com"
print(check_website(url))
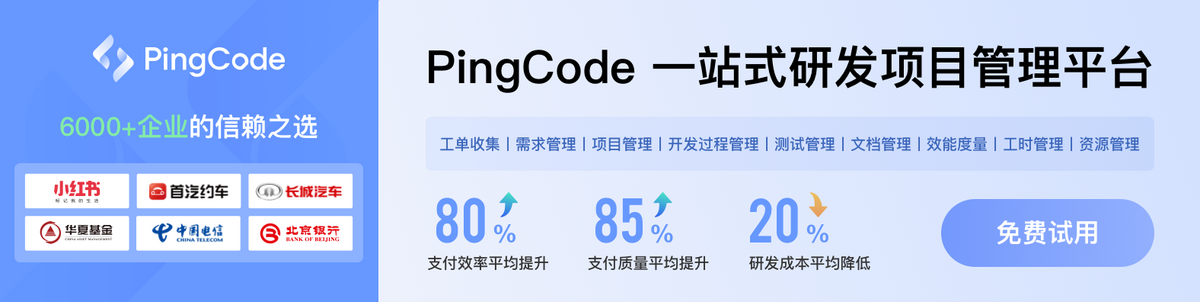