如何在 Python 中将列表中的数字处理
在 Python 中将列表中的数字进行处理的方法有很多,包括:使用列表推导式、使用 map() 函数、使用 for 循环等。 其中,列表推导式是一种简洁且优雅的方法,尤其适用于简单的操作。我们以将列表中的每个数字平方为例,来详细说明使用列表推导式的方法。
列表推导式是一种生成列表的简洁语法,可以通过一个表达式和一个循环来生成列表。假设我们有一个包含数字的列表 numbers
,我们可以通过以下代码将每个数字平方:
numbers = [1, 2, 3, 4, 5]
squared_numbers = [x2 for x in numbers]
print(squared_numbers) # 输出: [1, 4, 9, 16, 25]
下面我们将详细探讨在 Python 中处理列表中数字的不同方法。
一、使用列表推导式
列表推导式是一种简洁的语法,可以用来生成新的列表。它不仅简化了代码,还提高了代码的可读性。
示例:将列表中的每个数字平方
假设我们有一个包含数字的列表 numbers
,我们想要生成一个新的列表,包含每个数字的平方。可以使用列表推导式来实现:
numbers = [1, 2, 3, 4, 5]
squared_numbers = [x2 for x in numbers]
print(squared_numbers) # 输出: [1, 4, 9, 16, 25]
示例:将列表中的每个数字加 1
我们也可以使用列表推导式来生成一个包含每个数字加 1 的新列表:
numbers = [1, 2, 3, 4, 5]
incremented_numbers = [x + 1 for x in numbers]
print(incremented_numbers) # 输出: [2, 3, 4, 5, 6]
二、使用 map() 函数
map()
函数是 Python 内置的高阶函数,可以将一个函数应用到一个或多个序列(如列表)中的每个元素,并返回一个迭代器。我们可以使用 map()
函数来对列表中的每个数字进行操作。
示例:将列表中的每个数字平方
numbers = [1, 2, 3, 4, 5]
squared_numbers = list(map(lambda x: x2, numbers))
print(squared_numbers) # 输出: [1, 4, 9, 16, 25]
示例:将列表中的每个数字加 1
numbers = [1, 2, 3, 4, 5]
incremented_numbers = list(map(lambda x: x + 1, numbers))
print(incremented_numbers) # 输出: [2, 3, 4, 5, 6]
三、使用 for 循环
对于一些更复杂的操作,或者当你不习惯使用列表推导式和 map()
函数时,可以使用传统的 for
循环来处理列表中的数字。
示例:将列表中的每个数字平方
numbers = [1, 2, 3, 4, 5]
squared_numbers = []
for x in numbers:
squared_numbers.append(x2)
print(squared_numbers) # 输出: [1, 4, 9, 16, 25]
示例:将列表中的每个数字加 1
numbers = [1, 2, 3, 4, 5]
incremented_numbers = []
for x in numbers:
incremented_numbers.append(x + 1)
print(incremented_numbers) # 输出: [2, 3, 4, 5, 6]
四、使用 NumPy 库
如果你处理的是大量的数字,或者需要进行复杂的数学运算,可以考虑使用 NumPy 库。NumPy 提供了高效的多维数组操作,并且支持大量的数学函数。
安装 NumPy
首先,需要安装 NumPy 库:
pip install numpy
示例:将列表中的每个数字平方
import numpy as np
numbers = np.array([1, 2, 3, 4, 5])
squared_numbers = numbers2
print(squared_numbers) # 输出: [ 1 4 9 16 25]
示例:将列表中的每个数字加 1
import numpy as np
numbers = np.array([1, 2, 3, 4, 5])
incremented_numbers = numbers + 1
print(incremented_numbers) # 输出: [2 3 4 5 6]
五、使用 pandas 库
对于数据分析任务,pandas 库非常有用。pandas 提供了强大的数据结构和数据分析工具。
安装 pandas
首先,需要安装 pandas 库:
pip install pandas
示例:将列表中的每个数字平方
import pandas as pd
numbers = pd.Series([1, 2, 3, 4, 5])
squared_numbers = numbers2
print(squared_numbers) # 输出: 0 1
# 1 4
# 2 9
# 3 16
# 4 25
# dtype: int64
示例:将列表中的每个数字加 1
import pandas as pd
numbers = pd.Series([1, 2, 3, 4, 5])
incremented_numbers = numbers + 1
print(incremented_numbers) # 输出: 0 2
# 1 3
# 2 4
# 3 5
# 4 6
# dtype: int64
六、总结
在 Python 中处理列表中的数字有很多种方法,包括使用列表推导式、map() 函数、for 循环、NumPy 库和 pandas 库。选择哪种方法取决于具体的需求和个人的编程风格。列表推导式和 map()
函数适合于简单的操作,而 for
循环则适合于更复杂的操作。对于处理大量数据或进行复杂数学运算,NumPy 和 pandas 是非常有用的工具。希望通过这篇文章,你能够更加灵活地处理 Python 列表中的数字。
相关问答FAQs:
如何在Python中对列表中的数字进行排序?
在Python中,可以使用内置的sort()
方法或sorted()
函数对列表中的数字进行排序。sort()
方法会直接修改原始列表,而sorted()
函数则返回一个新的排序列表。示例代码如下:
numbers = [5, 3, 8, 1, 2]
numbers.sort() # 对原列表进行排序
print(numbers) # 输出: [1, 2, 3, 5, 8]
# 或者使用sorted函数
sorted_numbers = sorted(numbers)
print(sorted_numbers) # 输出: [1, 2, 3, 5, 8]
如何在Python中计算列表中数字的总和?
可以使用内置的sum()
函数来计算列表中所有数字的总和。此函数会遍历列表并返回所有元素的和。例如:
numbers = [5, 3, 8, 1, 2]
total = sum(numbers)
print(total) # 输出: 19
如何在Python中筛选出列表中的偶数?
为了从列表中筛选出偶数,可以使用列表推导式或filter()
函数。以下是两种常见的方法:
使用列表推导式:
numbers = [5, 3, 8, 1, 2]
even_numbers = [num for num in numbers if num % 2 == 0]
print(even_numbers) # 输出: [8, 2]
使用filter()
函数:
numbers = [5, 3, 8, 1, 2]
even_numbers = list(filter(lambda x: x % 2 == 0, numbers))
print(even_numbers) # 输出: [8, 2]
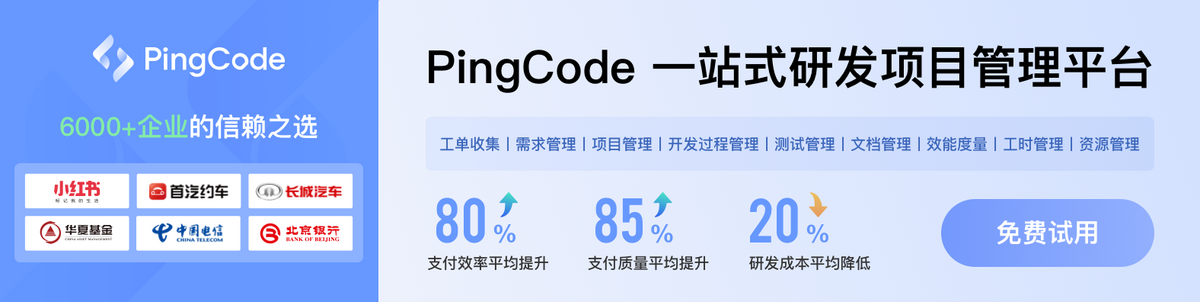