要用Python创建一个NPC(非玩家角色),你需要以下几个步骤:定义角色属性、实现角色行为、创建角色交互。 其中,角色行为的实现尤其重要,因为它直接决定了NPC的智能与互动能力。通过编写合适的算法和逻辑,你可以让NPC在游戏中表现得更加自然和真实。
一、定义角色属性
NPC的属性通常包括名称、生命值、力量、位置等基本信息。这些属性可以通过Python的类来实现。
class NPC:
def __init__(self, name, health, strength, position):
self.name = name
self.health = health
self.strength = strength
self.position = position
npc = NPC("Guard", 100, 10, (5, 5))
print(npc.name, npc.health, npc.strength, npc.position)
在这个例子中,我们创建了一个基本的NPC类,并实例化了一个名为“Guard”的NPC。我们定义了NPC的名称、生命值、力量和位置。
二、实现角色行为
NPC的行为是通过方法来定义的,这些方法可以包括移动、攻击、防御等。以下是一些基本行为的示例:
1、移动
class NPC:
def __init__(self, name, health, strength, position):
self.name = name
self.health = health
self.strength = strength
self.position = position
def move(self, new_position):
self.position = new_position
print(f"{self.name} moved to {self.position}")
npc = NPC("Guard", 100, 10, (5, 5))
npc.move((10, 10))
在这个例子中,我们添加了一个move
方法来更新NPC的位置。
2、攻击
class NPC:
def __init__(self, name, health, strength, position):
self.name = name
self.health = health
self.strength = strength
self.position = position
def move(self, new_position):
self.position = new_position
print(f"{self.name} moved to {self.position}")
def attack(self, target):
target.health -= self.strength
print(f"{self.name} attacked {target.name} for {self.strength} damage")
class Player:
def __init__(self, name, health, position):
self.name = name
self.health = health
self.position = position
player = Player("Hero", 100, (0, 0))
npc = NPC("Guard", 100, 10, (5, 5))
npc.attack(player)
print(player.name, player.health)
在这个示例中,我们创建了一个玩家类,并为NPC添加了一个攻击方法。NPC能够攻击玩家并减少玩家的生命值。
三、创建角色交互
为了使NPC更智能和互动性更强,我们需要编写一些高级行为逻辑。例如,NPC可能会在特定条件下做出不同的反应。
1、巡逻行为
class NPC:
def __init__(self, name, health, strength, position, patrol_points):
self.name = name
self.health = health
self.strength = strength
self.position = position
self.patrol_points = patrol_points
self.current_patrol_index = 0
def patrol(self):
self.position = self.patrol_points[self.current_patrol_index]
self.current_patrol_index = (self.current_patrol_index + 1) % len(self.patrol_points)
print(f"{self.name} patrolled to {self.position}")
patrol_points = [(1, 1), (2, 2), (3, 3)]
npc = NPC("Guard", 100, 10, (0, 0), patrol_points)
npc.patrol()
npc.patrol()
npc.patrol()
在这个例子中,我们为NPC添加了一个巡逻行为。NPC会按顺序移动到预定义的巡逻点。
2、追逐行为
class NPC:
def __init__(self, name, health, strength, position):
self.name = name
self.health = health
self.strength = strength
self.position = position
def chase(self, target):
self.position = target.position
print(f"{self.name} chased {target.name} to {self.position}")
class Player:
def __init__(self, name, health, position):
self.name = name
self.health = health
self.position = position
player = Player("Hero", 100, (10, 10))
npc = NPC("Guard", 100, 10, (5, 5))
npc.chase(player)
在这个示例中,我们为NPC添加了一个追逐行为。NPC会追逐目标玩家并移动到玩家的位置。
四、添加更多复杂行为
为了使NPC更加智能和复杂,可以添加更多的行为和决策逻辑。例如,NPC可以根据玩家的距离决定是否进行攻击或逃跑。
1、决策树
class NPC:
def __init__(self, name, health, strength, position):
self.name = name
self.health = health
self.strength = strength
self.position = position
def distance_to(self, target):
return ((self.position[0] - target.position[0]) <strong> 2 + (self.position[1] - target.position[1]) </strong> 2) 0.5
def decide_action(self, target):
distance = self.distance_to(target)
if distance < 5:
self.attack(target)
else:
self.chase(target)
def attack(self, target):
target.health -= self.strength
print(f"{self.name} attacked {target.name} for {self.strength} damage")
def chase(self, target):
self.position = target.position
print(f"{self.name} chased {target.name} to {self.position}")
class Player:
def __init__(self, name, health, position):
self.name = name
self.health = health
self.position = position
player = Player("Hero", 100, (10, 10))
npc = NPC("Guard", 100, 10, (5, 5))
npc.decide_action(player)
print(player.name, player.health)
在这个示例中,我们为NPC添加了一个决策树。NPC会根据与玩家的距离决定是攻击还是追逐玩家。
2、状态机
class NPC:
def __init__(self, name, health, strength, position):
self.name = name
self.health = health
self.strength = strength
self.position = position
self.state = "idle"
def distance_to(self, target):
return ((self.position[0] - target.position[0]) <strong> 2 + (self.position[1] - target.position[1]) </strong> 2) 0.5
def update(self, target):
distance = self.distance_to(target)
if self.state == "idle" and distance < 10:
self.state = "chase"
elif self.state == "chase" and distance < 5:
self.state = "attack"
if self.state == "chase":
self.chase(target)
elif self.state == "attack":
self.attack(target)
def attack(self, target):
target.health -= self.strength
print(f"{self.name} attacked {target.name} for {self.strength} damage")
def chase(self, target):
self.position = target.position
print(f"{self.name} chased {target.name} to {self.position}")
class Player:
def __init__(self, name, health, position):
self.name = name
self.health = health
self.position = position
player = Player("Hero", 100, (10, 10))
npc = NPC("Guard", 100, 10, (5, 5))
npc.update(player)
print(player.name, player.health)
在这个例子中,我们为NPC添加了一个状态机。NPC会根据状态和玩家的距离更新自己的行为。
五、环境交互
NPC不仅需要与玩家交互,还需要与环境进行交互。这可以通过添加感知和反应机制来实现。例如,NPC可以感知周围的障碍物并选择绕过它们。
1、感知机制
class NPC:
def __init__(self, name, health, strength, position, vision_range):
self.name = name
self.health = health
self.strength = strength
self.position = position
self.vision_range = vision_range
def can_see(self, target):
distance = ((self.position[0] - target.position[0]) <strong> 2 + (self.position[1] - target.position[1]) </strong> 2) 0.5
return distance <= self.vision_range
class Player:
def __init__(self, name, health, position):
self.name = name
self.health = health
self.position = position
player = Player("Hero", 100, (10, 10))
npc = NPC("Guard", 100, 10, (5, 5), 15)
if npc.can_see(player):
print(f"{npc.name} can see {player.name}")
else:
print(f"{npc.name} cannot see {player.name}")
在这个示例中,我们为NPC添加了一个视野范围。NPC可以检测目标是否在视野范围内。
2、路径规划
class NPC:
def __init__(self, name, health, strength, position):
self.name = name
self.health = health
self.strength = strength
self.position = position
def move_to(self, destination):
self.position = destination
print(f"{self.name} moved to {self.position}")
def find_path(self, start, end):
# 简单的路径规划算法
path = [start]
current_position = start
while current_position != end:
if current_position[0] < end[0]:
current_position = (current_position[0] + 1, current_position[1])
elif current_position[0] > end[0]:
current_position = (current_position[0] - 1, current_position[1])
if current_position[1] < end[1]:
current_position = (current_position[0], current_position[1] + 1)
elif current_position[1] > end[1]:
current_position = (current_position[0], current_position[1] - 1)
path.append(current_position)
return path
npc = NPC("Guard", 100, 10, (0, 0))
path = npc.find_path((0, 0), (5, 5))
for step in path:
npc.move_to(step)
在这个示例中,我们为NPC添加了一个简单的路径规划算法。NPC能够找到从起点到终点的路径并逐步移动。
六、总结
通过上述步骤,你可以使用Python创建一个功能丰富且智能的NPC。关键在于定义角色属性、实现角色行为、创建角色交互以及添加更多复杂行为和环境交互。随着需求的增加,你可以进一步扩展NPC的功能,例如添加更多的感知机制、高级的路径规划算法和复杂的决策树或状态机。这样,你的NPC将更加智能和互动性更强,为游戏或应用提供更好的用户体验。
相关问答FAQs:
如何用Python创建一个简单的NPC(非玩家角色)?
要创建一个NPC,您可以使用Python的面向对象编程特性。首先,定义一个NPC类,包含属性如名字、生命值和行为方法。例如:
class NPC:
def __init__(self, name, health):
self.name = name
self.health = health
def speak(self):
print(f"{self.name} says: Hello, traveler!")
def take_damage(self, damage):
self.health -= damage
print(f"{self.name} takes {damage} damage and has {self.health} health left.")
通过这种方式,您可以创建多个具有不同属性和行为的NPC实例。
在Python中如何使NPC与玩家进行交互?
为了使NPC与玩家进行互动,您可以使用输入函数来获取玩家的选择。例如,您可以让玩家选择与NPC对话或战斗:
npc = NPC("Gandalf", 100)
action = input("Do you want to talk to or fight Gandalf? (talk/fight): ").strip().lower()
if action == "talk":
npc.speak()
elif action == "fight":
npc.take_damage(20)
else:
print("Invalid action.")
通过这种方式,您可以创建互动体验,让玩家在游戏中做出选择。
如何在Python中为NPC添加更多功能和复杂性?
为了增加NPC的复杂性,可以为其添加更多的行为和状态。例如,可以引入状态机来管理NPC的行为,或者使用状态属性来决定NPC在不同情况下的反应。此外,可以考虑增加对话系统,允许玩家与NPC进行更深入的交流。
class NPC:
def __init__(self, name, health):
self.name = name
self.health = health
self.is_hostile = False
def engage(self):
if self.is_hostile:
print(f"{self.name} is attacking!")
else:
print(f"{self.name} is friendly.")
def toggle_hostility(self):
self.is_hostile = not self.is_hostile
state = "hostile" if self.is_hostile else "friendly"
print(f"{self.name} is now {state}.")
通过这种方式,您可以根据游戏的进展和玩家的选择调整NPC的行为,使游戏体验更加丰富。
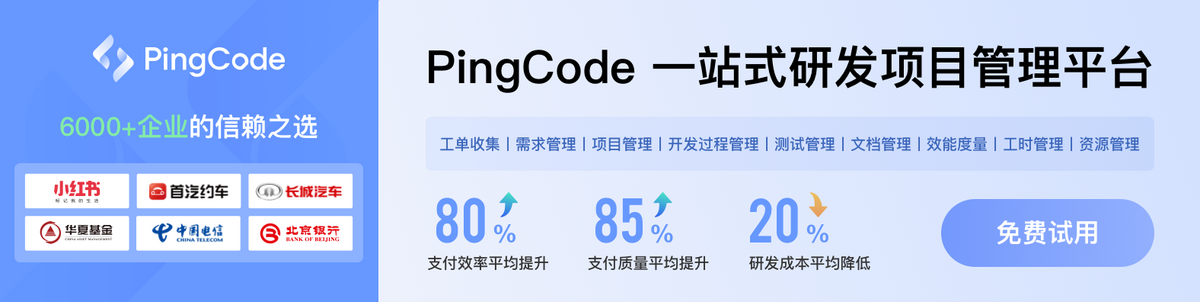