Python进行接口测试用例的方法包括:使用requests库进行HTTP请求、使用unittest框架进行测试管理、使用mock模拟接口响应。
其中,使用requests库进行HTTP请求,可以方便地模拟客户端向服务器发起请求,并接收响应数据。requests库具有易用、功能强大、支持多种HTTP方法等特点,使得编写接口测试用例变得更加简单高效。
下面我们将详细介绍如何在Python中进行接口测试用例。
一、使用requests库进行HTTP请求
requests库是一个非常流行的Python库,用于发送HTTP请求。以下是使用requests库进行接口测试的基本步骤:
安装requests库
首先,确保你已经安装了requests库。如果没有安装,可以使用以下命令进行安装:
pip install requests
发送HTTP请求
requests库支持多种HTTP方法,例如GET、POST、PUT、DELETE等。以下是一些常用的请求示例:
发送GET请求
import requests
response = requests.get('https://api.example.com/resource')
print(response.status_code)
print(response.json())
发送POST请求
import requests
data = {'key1': 'value1', 'key2': 'value2'}
response = requests.post('https://api.example.com/resource', json=data)
print(response.status_code)
print(response.json())
发送带有Headers的请求
import requests
headers = {'Authorization': 'Bearer YOUR_ACCESS_TOKEN'}
response = requests.get('https://api.example.com/resource', headers=headers)
print(response.status_code)
print(response.json())
二、使用unittest框架进行测试管理
unittest是Python内置的测试框架,可以用来编写和运行测试用例。以下是使用unittest编写接口测试用例的基本步骤:
编写测试用例
首先,创建一个测试类并继承unittest.TestCase。然后,定义测试方法,并在方法中使用requests库发送HTTP请求并进行断言。
import unittest
import requests
class TestAPI(unittest.TestCase):
def test_get_resource(self):
response = requests.get('https://api.example.com/resource')
self.assertEqual(response.status_code, 200)
self.assertIn('key1', response.json())
def test_post_resource(self):
data = {'key1': 'value1', 'key2': 'value2'}
response = requests.post('https://api.example.com/resource', json=data)
self.assertEqual(response.status_code, 201)
self.assertEqual(response.json()['key1'], 'value1')
if __name__ == '__main__':
unittest.main()
运行测试用例
运行测试用例可以直接在命令行中执行脚本:
python test_api.py
三、使用mock模拟接口响应
在某些情况下,接口可能不可用或不方便进行真实请求。这时可以使用unittest.mock库来模拟接口响应。
模拟GET请求响应
import unittest
from unittest.mock import patch
import requests
class TestAPI(unittest.TestCase):
@patch('requests.get')
def test_get_resource(self, mock_get):
mock_get.return_value.status_code = 200
mock_get.return_value.json.return_value = {'key1': 'value1'}
response = requests.get('https://api.example.com/resource')
self.assertEqual(response.status_code, 200)
self.assertIn('key1', response.json())
if __name__ == '__main__':
unittest.main()
模拟POST请求响应
import unittest
from unittest.mock import patch
import requests
class TestAPI(unittest.TestCase):
@patch('requests.post')
def test_post_resource(self, mock_post):
mock_post.return_value.status_code = 201
mock_post.return_value.json.return_value = {'key1': 'value1'}
data = {'key1': 'value1', 'key2': 'value2'}
response = requests.post('https://api.example.com/resource', json=data)
self.assertEqual(response.status_code, 201)
self.assertEqual(response.json()['key1'], 'value1')
if __name__ == '__main__':
unittest.main()
通过以上方法,可以使用Python进行接口测试用例的编写和管理。下面将进一步介绍一些高级用法和技巧。
四、使用参数化测试
在编写接口测试用例时,通常需要针对不同的输入数据进行测试。unittest框架可以使用参数化测试来简化这类测试的编写。
使用ddt库进行参数化测试
ddt(Data-Driven Tests)是一个用于参数化测试的Python库。可以使用以下命令安装ddt:
pip install ddt
以下是使用ddt编写参数化测试用例的示例:
import unittest
import requests
from ddt import ddt, data, unpack
@ddt
class TestAPI(unittest.TestCase):
@data(
('https://api.example.com/resource1', 200),
('https://api.example.com/resource2', 404)
)
@unpack
def test_get_resource(self, url, expected_status):
response = requests.get(url)
self.assertEqual(response.status_code, expected_status)
if __name__ == '__main__':
unittest.main()
五、使用pytest进行测试
除了unittest外,pytest也是一个非常流行的测试框架,具有更简洁的语法和更强大的功能。以下是使用pytest编写接口测试用例的基本步骤:
安装pytest
使用以下命令安装pytest:
pip install pytest
编写测试用例
以下是使用pytest编写的接口测试用例示例:
import requests
def test_get_resource():
response = requests.get('https://api.example.com/resource')
assert response.status_code == 200
assert 'key1' in response.json()
def test_post_resource():
data = {'key1': 'value1', 'key2': 'value2'}
response = requests.post('https://api.example.com/resource', json=data)
assert response.status_code == 201
assert response.json()['key1'] == 'value1'
运行测试用例
在命令行中运行以下命令来执行测试用例:
pytest test_api.py
六、生成测试报告
生成测试报告可以帮助更好地了解测试执行结果和覆盖率。pytest支持多种报告插件,例如pytest-html和pytest-cov。
安装pytest-html
使用以下命令安装pytest-html:
pip install pytest-html
生成HTML报告
在命令行中运行以下命令来生成HTML报告:
pytest --html=report.html
安装pytest-cov
使用以下命令安装pytest-cov:
pip install pytest-cov
生成覆盖率报告
在命令行中运行以下命令来生成覆盖率报告:
pytest --cov=your_module test_api.py
七、集成到CI/CD流水线
将接口测试集成到CI/CD流水线中,可以确保每次代码变更后自动运行测试,及时发现并修复问题。以下是一些常见的CI/CD工具和集成方法:
使用GitHub Actions
GitHub Actions是GitHub提供的CI/CD服务,可以使用以下配置文件集成接口测试:
name: API Tests
on: [push, pull_request]
jobs:
test:
runs-on: ubuntu-latest
steps:
- name: Checkout code
uses: actions/checkout@v2
- name: Set up Python
uses: actions/setup-python@v2
with:
python-version: 3.8
- name: Install dependencies
run: |
python -m pip install --upgrade pip
pip install requests pytest
- name: Run tests
run: pytest --html=report.html
使用Jenkins
Jenkins是一个开源的CI/CD工具,可以通过创建Pipeline脚本集成接口测试:
pipeline {
agent any
stages {
stage('Checkout') {
steps {
checkout scm
}
}
stage('Install dependencies') {
steps {
sh 'pip install requests pytest'
}
}
stage('Run tests') {
steps {
sh 'pytest --html=report.html'
}
}
}
post {
always {
archiveArtifacts artifacts: 'report.html', allowEmptyArchive: true
}
}
}
通过以上步骤,可以使用Python编写、管理、运行和报告接口测试用例,并集成到CI/CD流水线中,提高测试的自动化程度和效率。
相关问答FAQs:
接口测试用例需要哪些准备工作?
在进行Python接口测试之前,需要确保有一套清晰的接口文档,包含每个接口的请求方式、参数、返回值等信息。此外,准备好相关的测试环境和工具,比如Postman、Requests库等。确保你的Python环境已经安装了pytest、unittest等测试框架,这样可以有效地组织和运行测试用例。
使用Python进行接口测试有哪些常用库?
Python中有多个库可以用来进行接口测试,最常用的包括Requests库,用于发送HTTP请求;pytest和unittest用于测试框架;以及Swagger,用于接口文档的生成和测试。通过结合这些工具,可以实现高效的接口测试。
如何编写一个简单的Python接口测试用例?
编写接口测试用例时,可以使用Requests库发送请求并获取响应。示例代码如下:
import requests
def test_api_endpoint():
url = "https://api.example.com/endpoint"
response = requests.get(url)
assert response.status_code == 200
assert "expected_key" in response.json()
此代码示例展示了如何发送GET请求并验证响应状态码及返回数据的有效性。通过断言可以确保接口的功能符合预期。
如何处理接口测试中的错误和异常情况?
在接口测试中,处理错误和异常至关重要。可以通过捕获异常来记录错误信息,并进行相应的断言。例如,使用try-except块来捕获请求异常,并在断言中验证响应的状态码和内容。这样可以提高测试的稳定性和准确性,确保在出现问题时能够及时反馈。
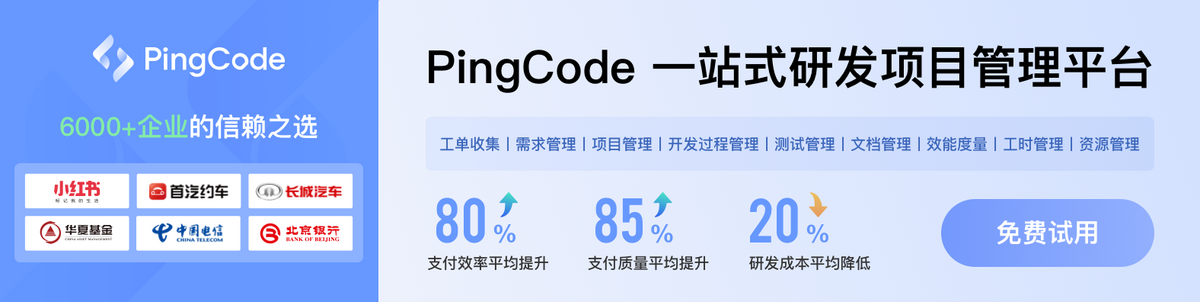