要判断读取的文件内容是否为空,可以通过以下几种方法:检查文件大小、检查读取的内容、检查文件行数。 其中,通过检查文件大小的方法是最为直接和高效的,因为它可以在不读取文件内容的情况下,直接判断文件是否为空。下面将详细描述如何实现这些方法。
一、检查文件大小
检查文件大小是判断文件是否为空的最简单和高效的方法。通过使用Python的os
模块,我们可以轻松获取文件的大小。如果文件大小为0字节,那么可以确定文件是空的。
import os
def is_file_empty(file_path):
return os.path.getsize(file_path) == 0
file_path = 'example.txt'
if is_file_empty(file_path):
print("The file is empty.")
else:
print("The file is not empty.")
在上面的代码中,os.path.getsize(file_path)
返回文件的大小(以字节为单位)。如果文件大小为0,则该文件为空。
二、检查读取的内容
另一种方法是读取文件的内容,并检查读取到的数据是否为空。这种方法可以用于处理较小的文件,因为它需要将文件内容读入内存。
1. 使用read()方法
def is_file_content_empty(file_path):
with open(file_path, 'r') as file:
content = file.read()
return content == ''
file_path = 'example.txt'
if is_file_content_empty(file_path):
print("The file content is empty.")
else:
print("The file content is not empty.")
在上面的代码中,file.read()
读取文件的全部内容,并将其存储在content
变量中。如果content
为空字符串,则文件内容为空。
2. 使用readlines()方法
def is_file_lines_empty(file_path):
with open(file_path, 'r') as file:
lines = file.readlines()
return len(lines) == 0
file_path = 'example.txt'
if is_file_lines_empty(file_path):
print("The file lines are empty.")
else:
print("The file lines are not empty.")
在上面的代码中,file.readlines()
读取文件的所有行,并将其存储在lines
列表中。如果lines
列表为空,则文件内容为空。
三、检查文件行数
通过逐行读取文件并检查是否存在至少一行内容,也可以判断文件是否为空。这种方法适用于需要处理非常大的文件,因为它逐行处理文件,而不是将整个文件读入内存。
def is_file_empty_by_lines(file_path):
with open(file_path, 'r') as file:
for line in file:
return False
return True
file_path = 'example.txt'
if is_file_empty_by_lines(file_path):
print("The file is empty by lines.")
else:
print("The file is not empty by lines.")
在上面的代码中,通过迭代文件的每一行,如果存在至少一行内容,则返回False
。如果遍历完所有行都没有内容,则返回True
。
四、结合多种方法
在实际应用中,结合多种方法可以提高判断的准确性和效率。例如,可以先检查文件大小,如果文件大小为0,则直接判断文件为空;否则,再进一步读取文件内容进行判断。
import os
def is_file_empty_combined(file_path):
if os.path.getsize(file_path) == 0:
return True
with open(file_path, 'r') as file:
content = file.read()
return content == ''
file_path = 'example.txt'
if is_file_empty_combined(file_path):
print("The file is empty (combined method).")
else:
print("The file is not empty (combined method).")
在上面的代码中,先检查文件大小,如果文件大小为0,则直接判断文件为空;否则,进一步读取文件内容进行判断。
五、处理二进制文件
上述方法主要适用于处理文本文件。如果需要处理二进制文件,可以使用类似的方法进行判断。
def is_binary_file_empty(file_path):
return os.path.getsize(file_path) == 0
file_path = 'example.bin'
if is_binary_file_empty(file_path):
print("The binary file is empty.")
else:
print("The binary file is not empty.")
在上面的代码中,通过检查二进制文件的大小来判断文件是否为空。
总结:通过检查文件大小、检查读取的内容、检查文件行数等方法可以判断读取的文件内容是否为空。检查文件大小是最简单和高效的方法,但在实际应用中,结合多种方法可以提高判断的准确性和效率。
相关问答FAQs:
如何在Python中有效判断文件是否为空?
在Python中,可以通过检查文件的大小来判断文件是否为空。使用os
模块中的stat
方法可以获取文件的状态信息,包括文件大小。以下是一个示例代码:
import os
file_path = 'your_file.txt' # 替换为你的文件路径
if os.stat(file_path).st_size == 0:
print("文件是空的")
else:
print("文件不是空的")
在读取文件内容后如何检查其内容是否为空?
在读取文件内容后,可以使用条件语句来检查读取的数据是否为空字符串。以下是相关示例:
with open('your_file.txt', 'r') as file:
content = file.read()
if not content:
print("文件内容为空")
else:
print("文件内容:", content)
为什么文件可能会被认为是空的?
文件可能被认为是空的有多种原因,包括文件创建后没有写入任何数据,或者文件内容被清空。也有可能是文件路径错误或文件损坏导致无法读取内容。在处理文件时,确保路径正确并验证文件的完整性也是很重要的。
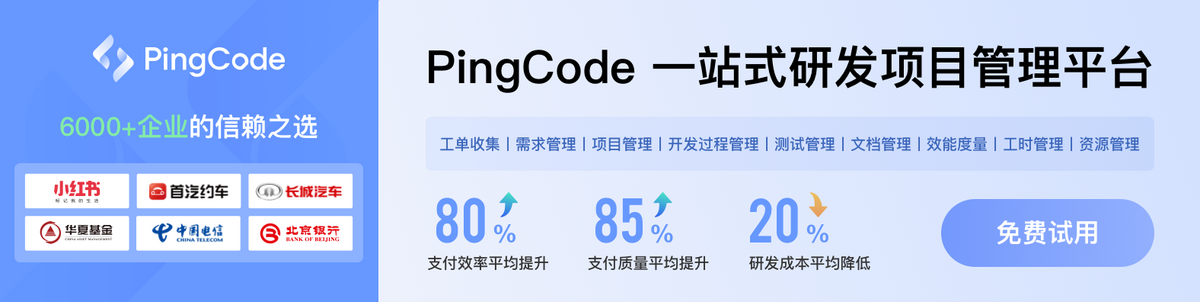