去除字符串空格的几种方法包括使用strip()、lstrip()、rstrip()、replace()、split()和join()。
其中最常用的方法是使用strip()函数,它可以去除字符串两端的空格。下面将对strip()函数的使用进行详细描述。
strip()函数:这个函数用于去除字符串两端的空格。它不会影响字符串中间的空格。例如:
my_string = " Hello World! "
cleaned_string = my_string.strip()
print(cleaned_string) # 输出 "Hello World!"
一、使用strip()去除两端空格
strip()函数是Python内置的字符串方法,用于去除字符串两端的空格。这个方法非常方便,特别适用于清理用户输入的数据,确保数据格式的一致性。
example_str = " Python is awesome! "
cleaned_str = example_str.strip()
print(cleaned_str) # 输出 "Python is awesome!"
strip()不仅可以去除空格,还可以去除其他指定字符,只需在括号内指定这些字符即可。例如:
example_str = "###Python is awesome!###"
cleaned_str = example_str.strip("#")
print(cleaned_str) # 输出 "Python is awesome!"
二、使用lstrip()去除左侧空格
lstrip()函数用于去除字符串左侧的空格或指定字符。这在处理需要保留右侧格式但去除左侧多余空格的场景中非常有用。
example_str = " Python is awesome! "
cleaned_str = example_str.lstrip()
print(cleaned_str) # 输出 "Python is awesome! "
同样,lstrip()也可以去除其他指定字符:
example_str = "###Python is awesome!###"
cleaned_str = example_str.lstrip("#")
print(cleaned_str) # 输出 "Python is awesome!###"
三、使用rstrip()去除右侧空格
与lstrip()相对应,rstrip()函数用于去除字符串右侧的空格或指定字符。
example_str = " Python is awesome! "
cleaned_str = example_str.rstrip()
print(cleaned_str) # 输出 " Python is awesome!"
同样,rstrip()也可以去除其他指定字符:
example_str = "###Python is awesome!###"
cleaned_str = example_str.rstrip("#")
print(cleaned_str) # 输出 "###Python is awesome!"
四、使用replace()去除所有空格
replace()函数可以将字符串中的所有指定字符替换为另一个字符。通过将空格替换为空字符串,可以去除字符串中的所有空格。
example_str = " Python is awesome! "
cleaned_str = example_str.replace(" ", "")
print(cleaned_str) # 输出 "Pythonisawesome!"
replace()非常灵活,可以替换任意字符或字符串:
example_str = "Python is awesome!"
cleaned_str = example_str.replace("awesome", "great")
print(cleaned_str) # 输出 "Python is great!"
五、使用split()和join()去除所有空格
split()和join()函数的组合可以有效地去除字符串中的所有空格。先使用split()将字符串按空格分割成多个子字符串,再使用join()将这些子字符串重新组合成一个字符串。
example_str = " Python is awesome! "
cleaned_str = "".join(example_str.split())
print(cleaned_str) # 输出 "Pythonisawesome!"
split()函数不仅可以按空格分割字符串,还可以按其他指定字符进行分割:
example_str = "Python#is#awesome!"
cleaned_str = "".join(example_str.split("#"))
print(cleaned_str) # 输出 "Pythonisawesome!"
六、使用正则表达式去除空格
正则表达式提供了更强大的字符串处理能力,可以匹配和替换复杂的字符串模式。通过使用re模块中的sub()函数,可以去除字符串中的所有空格。
import re
example_str = " Python is awesome! "
cleaned_str = re.sub(r"\s+", "", example_str)
print(cleaned_str) # 输出 "Pythonisawesome!"
正则表达式还可以用于匹配和替换其他复杂的字符串模式:
example_str = "Python is awesome!"
cleaned_str = re.sub(r"\s+", "-", example_str)
print(cleaned_str) # 输出 "Python-is-awesome!"
七、使用列表解析去除空格
列表解析提供了一种简洁的方法来处理字符串中的空格。通过遍历字符串中的每个字符,并仅保留非空格字符,可以去除字符串中的所有空格。
example_str = " Python is awesome! "
cleaned_str = "".join([char for char in example_str if char != " "])
print(cleaned_str) # 输出 "Pythonisawesome!"
列表解析不仅可以用于去除空格,还可以用于处理其他字符或字符串模式:
example_str = "Python is awesome!"
cleaned_str = "".join([char for char in example_str if char.isalpha()])
print(cleaned_str) # 输出 "Pythonisawesome"
八、对字符串进行预处理
在实际应用中,经常需要对字符串进行预处理,以确保数据的一致性和准确性。通过结合上述方法,可以对字符串进行多层次的预处理,去除空格和其他不需要的字符。
example_str = " Python is awesome! "
cleaned_str = example_str.strip().replace(" ", "").lower()
print(cleaned_str) # 输出 "pythonisawesome!"
这种多层次的预处理方法可以确保字符串格式的一致性,特别是在处理用户输入的数据时非常有用。
九、总结与最佳实践
在处理字符串时,选择合适的方法非常重要。不同的方法有不同的应用场景,了解每种方法的优缺点可以帮助你更好地处理字符串数据。
总结:
- strip()、lstrip()、rstrip():适用于去除字符串两端或一侧的空格或指定字符。
- replace():适用于替换字符串中的所有指定字符。
- split()和join():适用于按指定字符分割并重新组合字符串。
- 正则表达式:适用于匹配和替换复杂的字符串模式。
- 列表解析:适用于按条件过滤字符串中的字符。
在实际应用中,结合多种方法进行预处理,可以确保字符串数据的准确性和一致性。
总之,掌握这些方法可以帮助你在Python编程中更高效地处理字符串数据,提升代码的可读性和维护性。无论是处理用户输入、文本分析还是数据清理,这些方法都将是你不可或缺的工具。
相关问答FAQs:
如何在Python中去掉字符串开头和结尾的空格?
在Python中,可以使用strip()
方法来去掉字符串开头和结尾的空格。这个方法会返回一个新的字符串,原字符串保持不变。例如:
text = " Hello, World! "
cleaned_text = text.strip()
print(cleaned_text) # 输出: "Hello, World!"
如何去掉字符串中间的空格?
如果需要去掉字符串中间的所有空格,可以使用replace()
方法。这个方法可以将指定的字符替换为空字符串。示例代码如下:
text = "Hello, World!"
no_spaces_text = text.replace(" ", "")
print(no_spaces_text) # 输出: "Hello,World!"
在Python中是否可以使用正则表达式去除空格?
是的,使用re
模块可以通过正则表达式来去除字符串中的空格。可以使用re.sub()
函数来替换掉所有空格。以下是一个示例:
import re
text = " Hello, World! "
cleaned_text = re.sub(r'\s+', ' ', text).strip()
print(cleaned_text) # 输出: "Hello, World!"
这种方法适用于需要处理多种空白字符的情况,如制表符和换行符。
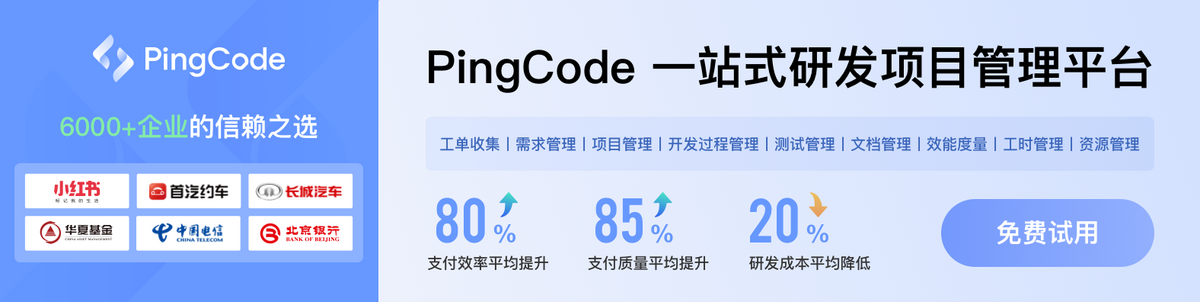