Python根据POST请求获取数据类型,可以通过解析请求头信息或请求体内容来实现。常见的做法包括:解析Content-Type头信息、使用request库解析请求体、根据请求体内容推断数据类型。下面将详细介绍这些方法,并提供具体的代码示例。
一、解析Content-Type头信息
在处理HTTP POST请求时,Content-Type
头信息指明了请求体的媒体类型。通过解析Content-Type
头信息,可以确定请求体的数据类型。以下是一些常见的Content-Type
值:
application/json
:表示请求体是JSON格式的数据。application/x-www-form-urlencoded
:表示请求体是URL编码的表单数据。multipart/form-data
:表示请求体是多部分表单数据,通常用于文件上传。
示例代码
from flask import Flask, request
app = Flask(__name__)
@app.route('/post', methods=['POST'])
def handle_post():
content_type = request.headers.get('Content-Type')
if content_type == 'application/json':
data_type = 'JSON'
elif content_type == 'application/x-www-form-urlencoded':
data_type = 'URL-encoded form data'
elif content_type == 'multipart/form-data':
data_type = 'Multipart form data'
else:
data_type = 'Unknown'
return f'Data type: {data_type}', 200
if __name__ == '__main__':
app.run(debug=True)
二、使用request库解析请求体
在处理POST请求时,Python的request
库(如Flask中的request
对象)可以自动解析请求体的内容。通过检查解析结果,可以进一步确认数据类型。
解析JSON数据
如果Content-Type
是application/json
,可以使用request.get_json()
方法解析请求体的JSON内容:
@app.route('/post', methods=['POST'])
def handle_post():
content_type = request.headers.get('Content-Type')
if content_type == 'application/json':
data = request.get_json()
return f'Received JSON data: {data}', 200
return 'Unsupported Content-Type', 400
解析表单数据
如果Content-Type
是application/x-www-form-urlencoded
或multipart/form-data
,可以使用request.form
解析表单数据:
@app.route('/post', methods=['POST'])
def handle_post():
content_type = request.headers.get('Content-Type')
if content_type in ['application/x-www-form-urlencoded', 'multipart/form-data']:
data = request.form
return f'Received form data: {data}', 200
return 'Unsupported Content-Type', 400
三、根据请求体内容推断数据类型
有时,Content-Type
头信息可能不准确或缺失。在这种情况下,可以根据请求体的内容推断数据类型。例如,通过尝试解析请求体的JSON内容来判断是否为JSON格式。
示例代码
import json
@app.route('/post', methods=['POST'])
def handle_post():
content_type = request.headers.get('Content-Type')
if content_type:
if content_type == 'application/json':
data = request.get_json()
return f'Received JSON data: {data}', 200
elif content_type in ['application/x-www-form-urlencoded', 'multipart/form-data']:
data = request.form
return f'Received form data: {data}', 200
else:
return 'Unsupported Content-Type', 400
else:
# 尝试解析JSON数据
try:
data = json.loads(request.data)
return f'Received JSON data: {data}', 200
except json.JSONDecodeError:
pass
# 尝试解析表单数据
data = request.form
if data:
return f'Received form data: {data}', 200
return 'Unable to determine data type', 400
四、综合处理方法
在实际应用中,可能需要结合多种方法来处理不同类型的数据。以下是一个综合处理POST请求的示例代码:
from flask import Flask, request
import json
app = Flask(__name__)
@app.route('/post', methods=['POST'])
def handle_post():
content_type = request.headers.get('Content-Type')
if content_type:
if content_type == 'application/json':
data = request.get_json()
data_type = 'JSON'
elif content_type in ['application/x-www-form-urlencoded', 'multipart/form-data']:
data = request.form
data_type = 'Form data'
else:
return 'Unsupported Content-Type', 400
else:
# 尝试解析JSON数据
try:
data = json.loads(request.data)
data_type = 'JSON'
except json.JSONDecodeError:
# 尝试解析表单数据
data = request.form
if data:
data_type = 'Form data'
else:
return 'Unable to determine data type', 400
return f'Received {data_type}: {data}', 200
if __name__ == '__main__':
app.run(debug=True)
结论
通过解析Content-Type
头信息、使用request
库解析请求体、以及根据请求体内容推断数据类型,可以在Python中有效地处理POST请求并确定数据类型。这些方法可以帮助开发者根据POST请求获取数据类型,并根据数据类型进行相应的处理。
相关问答FAQs:
如何在Python中通过POST请求获取数据类型?
在Python中,可以使用Flask或Django等框架来处理POST请求,获取数据类型。通常情况下,使用request
对象能够轻松获取请求体中的数据。例如,在Flask中,您可以使用request.json
获取JSON数据,使用request.form
获取表单数据。这些方法可以帮助您判断传入数据的类型。
在Python中,如何区分不同类型的POST数据?
在处理POST请求时,您可以通过检查Content-Type
头来区分数据类型。例如,如果Content-Type
是application/json
,您可以使用request.get_json()
来解析JSON数据。如果是application/x-www-form-urlencoded
,则可以使用request.form
获取表单数据。这样的判断可以帮助您正确处理不同格式的数据。
如何处理POST请求中可能出现的数据错误?
在处理POST请求时,数据错误是常见的问题。您可以使用try-except块捕获解析JSON或表单数据时可能出现的异常。例如,使用request.get_json()
时,如果数据格式不正确,会抛出werkzeug.exceptions.BadRequest
异常。通过合适的错误处理,您可以向用户返回有用的错误信息,帮助他们纠正数据格式。
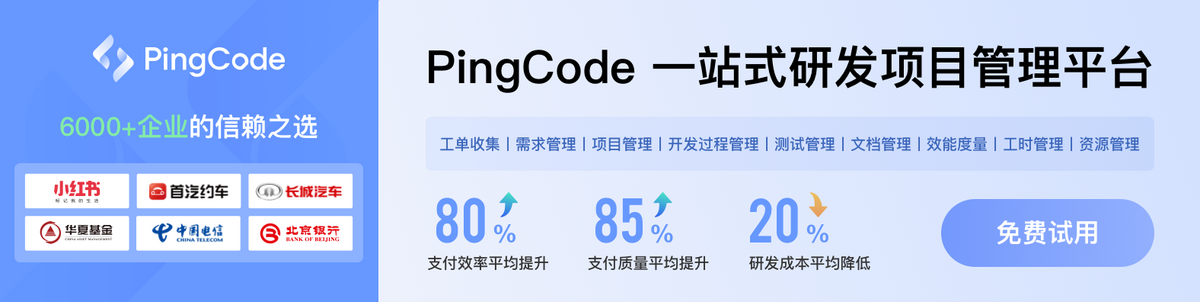