Python操作数据文件的基本方法包括:打开文件、读取文件、写入文件、关闭文件、使用上下文管理器、处理CSV文件、处理Excel文件、使用Pandas库进行数据操作。在本文中,我们将详细介绍这些方法,并提供示例代码以帮助读者更好地理解和应用这些技巧。
一、打开文件、读取文件、写入文件、关闭文件
使用Python操作文件的基本步骤包括打开文件、读取或写入文件以及关闭文件。Python的内置open()
函数可以用于打开文件,并返回一个文件对象。可以使用该文件对象的方法进行读写操作,最后使用close()
方法关闭文件。
1.1、打开文件
要打开文件,使用open()
函数,该函数接受文件路径和模式作为参数。常见的模式包括:
'r'
:读取模式(默认)'w'
:写入模式(会覆盖文件内容)'a'
:追加模式(在文件末尾添加内容)'b'
:二进制模式(可与其他模式结合使用)
file = open('example.txt', 'r')
1.2、读取文件
读取文件内容可以使用以下几种方法:
read()
:读取整个文件内容readline()
:按行读取文件readlines()
:读取文件的所有行,并返回一个列表
content = file.read()
print(content)
line = file.readline()
print(line)
lines = file.readlines()
print(lines)
1.3、写入文件
写入文件使用write()
方法,该方法接受一个字符串作为参数,并将其写入文件。
file = open('example.txt', 'w')
file.write('Hello, World!')
file.close()
1.4、关闭文件
使用完文件后,必须关闭文件以释放资源。
file.close()
二、使用上下文管理器
使用上下文管理器(with语句)可以简化文件操作,并确保文件在使用完毕后自动关闭。
2.1、读取文件
with open('example.txt', 'r') as file:
content = file.read()
print(content)
2.2、写入文件
with open('example.txt', 'w') as file:
file.write('Hello, World!')
三、处理CSV文件
CSV(Comma Separated Values)文件是一种常见的数据存储格式,Python的csv
模块提供了读取和写入CSV文件的功能。
3.1、读取CSV文件
使用csv.reader()
函数可以读取CSV文件,并返回一个可迭代对象。
import csv
with open('example.csv', 'r') as file:
reader = csv.reader(file)
for row in reader:
print(row)
3.2、写入CSV文件
使用csv.writer()
函数可以写入CSV文件。
import csv
with open('example.csv', 'w', newline='') as file:
writer = csv.writer(file)
writer.writerow(['Name', 'Age', 'City'])
writer.writerow(['Alice', '30', 'New York'])
writer.writerow(['Bob', '25', 'San Francisco'])
四、处理Excel文件
Excel文件是另一种常见的数据存储格式,Python的openpyxl
和pandas
库提供了处理Excel文件的功能。
4.1、使用openpyxl库
openpyxl
库可以用于读取和写入Excel文件。
读取Excel文件
import openpyxl
workbook = openpyxl.load_workbook('example.xlsx')
sheet = workbook.active
for row in sheet.iter_rows(values_only=True):
print(row)
写入Excel文件
import openpyxl
workbook = openpyxl.Workbook()
sheet = workbook.active
sheet.append(['Name', 'Age', 'City'])
sheet.append(['Alice', '30', 'New York'])
sheet.append(['Bob', '25', 'San Francisco'])
workbook.save('example.xlsx')
4.2、使用Pandas库
Pandas库提供了更高级的功能来处理Excel文件。
读取Excel文件
import pandas as pd
df = pd.read_excel('example.xlsx')
print(df)
写入Excel文件
import pandas as pd
data = {
'Name': ['Alice', 'Bob'],
'Age': [30, 25],
'City': ['New York', 'San Francisco']
}
df = pd.DataFrame(data)
df.to_excel('example.xlsx', index=False)
五、使用Pandas库进行数据操作
Pandas库是Python中强大的数据分析工具,提供了大量函数和方法来操作数据文件。
5.1、读取CSV文件
使用read_csv()
函数可以读取CSV文件,并返回一个DataFrame对象。
import pandas as pd
df = pd.read_csv('example.csv')
print(df)
5.2、读取Excel文件
使用read_excel()
函数可以读取Excel文件,并返回一个DataFrame对象。
import pandas as pd
df = pd.read_excel('example.xlsx')
print(df)
5.3、数据清洗和转换
Pandas提供了许多函数和方法来清洗和转换数据。
删除缺失值
df = df.dropna()
填充缺失值
df = df.fillna(value=0)
数据分组和聚合
grouped = df.groupby('City').mean()
print(grouped)
5.4、数据可视化
Pandas与Matplotlib库结合,可以方便地进行数据可视化。
import pandas as pd
import matplotlib.pyplot as plt
df = pd.read_csv('example.csv')
df.plot(kind='bar', x='Name', y='Age')
plt.show()
六、处理JSON文件
JSON(JavaScript Object Notation)是一种常见的数据交换格式,Python的json
模块提供了读取和写入JSON文件的功能。
6.1、读取JSON文件
使用json.load()
函数可以读取JSON文件,并将其解析为Python对象。
import json
with open('example.json', 'r') as file:
data = json.load(file)
print(data)
6.2、写入JSON文件
使用json.dump()
函数可以将Python对象写入JSON文件。
import json
data = {
'name': 'Alice',
'age': 30,
'city': 'New York'
}
with open('example.json', 'w') as file:
json.dump(data, file, indent=4)
七、处理XML文件
XML(eXtensible Markup Language)是一种常见的数据存储和交换格式,Python的xml.etree.ElementTree
模块提供了读取和写入XML文件的功能。
7.1、读取XML文件
使用ElementTree
模块可以读取XML文件,并解析为树结构。
import xml.etree.ElementTree as ET
tree = ET.parse('example.xml')
root = tree.getroot()
for child in root:
print(child.tag, child.attrib)
7.2、写入XML文件
使用ElementTree
模块可以创建XML文件,并将其写入磁盘。
import xml.etree.ElementTree as ET
root = ET.Element('root')
child = ET.SubElement(root, 'child')
child.set('name', 'Alice')
tree = ET.ElementTree(root)
tree.write('example.xml')
八、处理SQLite数据库
SQLite是一种轻量级的关系型数据库,Python的sqlite3
模块提供了与SQLite数据库交互的功能。
8.1、连接数据库
使用sqlite3.connect()
函数可以连接到SQLite数据库,并返回一个连接对象。
import sqlite3
conn = sqlite3.connect('example.db')
8.2、创建表
使用连接对象的execute()
方法可以执行SQL语句。
conn.execute('''CREATE TABLE IF NOT EXISTS users
(id INTEGER PRIMARY KEY,
name TEXT,
age INTEGER,
city TEXT)''')
conn.commit()
8.3、插入数据
使用连接对象的execute()
方法可以插入数据。
conn.execute("INSERT INTO users (name, age, city) VALUES ('Alice', 30, 'New York')")
conn.commit()
8.4、查询数据
使用连接对象的execute()
方法可以查询数据,并使用fetchall()
方法获取结果。
cursor = conn.execute("SELECT * FROM users")
for row in cursor:
print(row)
8.5、关闭连接
使用连接对象的close()
方法可以关闭数据库连接。
conn.close()
九、处理HDF5文件
HDF5是一种用于存储和管理大型数据集的文件格式,Python的h5py
库提供了读取和写入HDF5文件的功能。
9.1、读取HDF5文件
使用h5py.File
类可以读取HDF5文件,并访问其中的数据集。
import h5py
with h5py.File('example.h5', 'r') as file:
dataset = file['dataset']
print(dataset[:])
9.2、写入HDF5文件
使用h5py.File
类可以创建HDF5文件,并写入数据集。
import h5py
import numpy as np
data = np.arange(100).reshape(10, 10)
with h5py.File('example.h5', 'w') as file:
file.create_dataset('dataset', data=data)
十、处理Parquet文件
Parquet是一种用于高效存储和查询大数据集的列式存储格式,Python的pyarrow
和fastparquet
库提供了读取和写入Parquet文件的功能。
10.1、使用pyarrow库
读取Parquet文件
import pyarrow.parquet as pq
table = pq.read_table('example.parquet')
df = table.to_pandas()
print(df)
写入Parquet文件
import pyarrow.parquet as pq
import pandas as pd
data = {
'Name': ['Alice', 'Bob'],
'Age': [30, 25],
'City': ['New York', 'San Francisco']
}
df = pd.DataFrame(data)
table = pa.Table.from_pandas(df)
pq.write_table(table, 'example.parquet')
10.2、使用fastparquet库
读取Parquet文件
import fastparquet
df = fastparquet.ParquetFile('example.parquet').to_pandas()
print(df)
写入Parquet文件
import fastparquet
import pandas as pd
data = {
'Name': ['Alice', 'Bob'],
'Age': [30, 25],
'City': ['New York', 'San Francisco']
}
df = pd.DataFrame(data)
fastparquet.write('example.parquet', df)
总结:本文详细介绍了Python操作数据文件的多种方法和技巧,包括基本文件操作、上下文管理器、处理CSV文件、处理Excel文件、使用Pandas库进行数据操作、处理JSON文件、处理XML文件、处理SQLite数据库、处理HDF5文件和处理Parquet文件。通过这些方法,读者可以高效地读取、写入和处理各种格式的数据文件。希望本文能为您提供有用的参考和帮助。
相关问答FAQs:
如何使用Python读取数据文件?
要读取数据文件,可以使用内置的open()
函数。根据文件类型的不同,常用的读取方式包括使用CSV模块、Pandas库或者直接读取文本文件。例如,对于CSV文件,可以使用import csv
模块中的csv.reader()
方法来逐行读取数据。如果你想要更强大的数据处理功能,Pandas库提供了pd.read_csv()
,能够快速将CSV文件转化为DataFrame格式,便于后续分析和处理。
Python支持哪些数据文件格式?
Python支持多种数据文件格式,包括文本文件(如.txt)、CSV文件(.csv)、Excel文件(.xlsx)、JSON文件(.json)、XML文件(.xml)等。针对不同格式,有对应的库可以使用,比如openpyxl
用于处理Excel文件,json
模块用于JSON数据,xml.etree.ElementTree
可用于解析XML文件。选择合适的库可以提高处理效率。
如何使用Python写入数据文件?
使用Python写入数据文件同样简单。可以使用open()
函数以写入模式打开文件,使用write()
或writelines()
方法将数据写入文件。对于CSV文件,使用csv.writer()
可以方便地将列表或字典格式的数据写入。而对于Excel文件,可以使用Pandas库的to_excel()
方法,轻松将DataFrame写入Excel文件。确保在写入完成后关闭文件,或者使用with
语句自动管理文件打开和关闭。
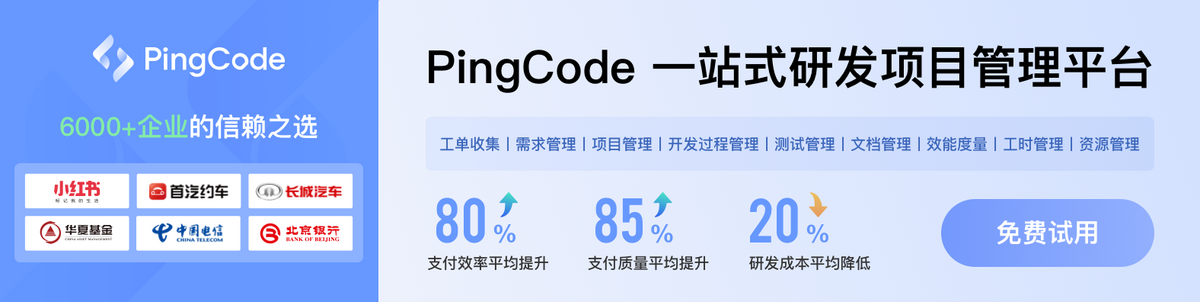