在Python类中多线程调用函数的方法包括:使用Thread类、使用ThreadPoolExecutor、使用多线程库等。 其中,使用Thread
类是最基础的方法,能够让我们更好地理解多线程的工作原理。使用ThreadPoolExecutor则更简洁高效,适合管理大量线程。下面,我将详细描述这两种方法,并提供相应的代码示例。
一、使用Thread类
Thread
类是Python标准库threading
模块中的核心类,用于创建和管理线程。通过继承Thread
类或者直接创建Thread
实例,可以实现多线程功能。
1.1、直接创建Thread实例
在这种方法中,我们直接创建Thread
实例,并将类中的方法作为目标函数传递给Thread
对象。
import threading
class MyClass:
def my_method(self, param1, param2):
print(f"Running with {param1} and {param2}")
def start_threads(self):
threads = []
for i in range(5):
t = threading.Thread(target=self.my_method, args=(i, i*2))
threads.append(t)
t.start()
for t in threads:
t.join()
if __name__ == "__main__":
obj = MyClass()
obj.start_threads()
在上面的代码中,我们创建了一个MyClass
类,并定义了一个my_method
方法。在start_threads
方法中,我们创建多个线程,每个线程运行my_method
方法,并传递不同的参数。
1.2、继承Thread类
另一种方法是通过继承Thread
类,将类中的方法作为线程的主函数。
import threading
class MyThread(threading.Thread):
def __init__(self, param1, param2):
threading.Thread.__init__(self)
self.param1 = param1
self.param2 = param2
def run(self):
print(f"Running with {self.param1} and {self.param2}")
if __name__ == "__main__":
threads = []
for i in range(5):
t = MyThread(i, i*2)
threads.append(t)
t.start()
for t in threads:
t.join()
在这个例子中,我们创建了一个MyThread
类,继承自threading.Thread
,并重写了run
方法。在run
方法中,我们实现了线程的具体功能。我们可以创建多个MyThread
实例,并启动它们。
二、使用ThreadPoolExecutor
ThreadPoolExecutor
是Python标准库concurrent.futures
模块中的一个类,用于管理线程池,简化多线程编程。
from concurrent.futures import ThreadPoolExecutor
class MyClass:
def my_method(self, param1, param2):
print(f"Running with {param1} and {param2}")
def start_threads(self):
with ThreadPoolExecutor(max_workers=5) as executor:
futures = [executor.submit(self.my_method, i, i*2) for i in range(5)]
for future in futures:
future.result()
if __name__ == "__main__":
obj = MyClass()
obj.start_threads()
在这个例子中,我们使用ThreadPoolExecutor
创建了一个线程池,并提交了多个任务给线程池执行。executor.submit
方法返回一个Future
对象,我们可以通过调用future.result
方法获取任务的执行结果。
三、使用多线程库
除了threading
和concurrent.futures
模块,Python还有其他一些多线程库,例如multiprocessing
模块和gevent
库。
3.1、使用multiprocessing模块
multiprocessing
模块支持进程间通信和共享数据,适用于CPU密集型任务。
from multiprocessing import Process
class MyClass:
def my_method(self, param1, param2):
print(f"Running with {param1} and {param2}")
def start_threads(self):
processes = []
for i in range(5):
p = Process(target=self.my_method, args=(i, i*2))
processes.append(p)
p.start()
for p in processes:
p.join()
if __name__ == "__main__":
obj = MyClass()
obj.start_threads()
在这个例子中,我们使用multiprocessing
模块创建了多个进程,每个进程运行my_method
方法。与线程不同,进程之间是独立的,适合在多核CPU上并行执行。
3.2、使用gevent库
gevent
是一个支持协程的并发库,适用于IO密集型任务。
import gevent
from gevent import monkey
monkey.patch_all()
class MyClass:
def my_method(self, param1, param2):
print(f"Running with {param1} and {param2}")
def start_threads(self):
greenlets = [gevent.spawn(self.my_method, i, i*2) for i in range(5)]
gevent.joinall(greenlets)
if __name__ == "__main__":
obj = MyClass()
obj.start_threads()
在这个例子中,我们使用gevent
库创建了多个协程,每个协程运行my_method
方法。gevent.spawn
方法返回一个Greenlet
对象,我们可以通过调用gevent.joinall
方法等待所有协程完成。
四、多线程编程的注意事项
4.1、线程安全
多线程编程中,线程安全是一个重要问题。多个线程同时访问共享资源时,可能会导致数据不一致。为了解决这个问题,我们可以使用锁(Lock)机制。
import threading
class MyClass:
def __init__(self):
self.lock = threading.Lock()
self.shared_resource = 0
def my_method(self, param1, param2):
with self.lock:
self.shared_resource += 1
print(f"Running with {param1} and {param2}, shared_resource: {self.shared_resource}")
def start_threads(self):
threads = []
for i in range(5):
t = threading.Thread(target=self.my_method, args=(i, i*2))
threads.append(t)
t.start()
for t in threads:
t.join()
if __name__ == "__main__":
obj = MyClass()
obj.start_threads()
在这个例子中,我们使用threading.Lock
创建了一个锁对象,并在访问共享资源时使用with self.lock
语句加锁,确保线程安全。
4.2、线程池的合理配置
在线程池中,线程数量的合理配置对性能有很大影响。线程数量过少可能导致资源未充分利用,线程数量过多则可能导致线程切换开销过大。一般来说,线程池大小可以根据任务的类型和系统资源进行调整。
4.3、避免死锁
在多线程编程中,死锁是一个常见问题。当多个线程互相等待对方释放资源时,就会发生死锁。为避免死锁,可以遵循以下原则:
- 尽量减少锁的使用范围。
- 按照固定顺序获取锁。
- 使用超时机制。
import threading
class MyClass:
def __init__(self):
self.lock1 = threading.Lock()
self.lock2 = threading.Lock()
def my_method1(self):
with self.lock1:
print("Acquired lock1 in my_method1")
with self.lock2:
print("Acquired lock2 in my_method1")
def my_method2(self):
with self.lock2:
print("Acquired lock2 in my_method2")
with self.lock1:
print("Acquired lock1 in my_method2")
def start_threads(self):
t1 = threading.Thread(target=self.my_method1)
t2 = threading.Thread(target=self.my_method2)
t1.start()
t2.start()
t1.join()
t2.join()
if __name__ == "__main__":
obj = MyClass()
obj.start_threads()
在这个例子中,我们模拟了一个可能导致死锁的场景。为避免死锁,我们可以重新设计代码,确保锁的获取顺序一致,或者使用超时机制。
总结
在Python类中多线程调用函数的方法有很多,包括使用Thread
类、ThreadPoolExecutor
、multiprocessing
模块和gevent
库等。每种方法有其优缺点和适用场景,选择适合的方法可以提高程序的性能和可靠性。在多线程编程中,线程安全、合理配置线程池和避免死锁是需要特别注意的问题。通过合理设计和使用多线程技术,可以有效提升程序的并发性能。
相关问答FAQs:
在Python类中如何实现多线程调用函数?
在Python中,可以使用threading
模块来实现多线程。您可以在类中定义一个方法,然后通过创建线程来调用该方法。通常,您需要创建一个线程对象,并将目标函数设为类中的方法。例如:
import threading
class MyClass:
def my_function(self):
print("Function is running in thread:", threading.current_thread().name)
# 创建类的实例
my_instance = MyClass()
# 创建线程
thread = threading.Thread(target=my_instance.my_function)
# 启动线程
thread.start()
这样,my_function
将在新线程中执行。
多线程对Python性能的影响是什么?
多线程可以提高程序的响应性,特别是对于I/O密集型任务,例如网络请求或文件操作。然而,由于Python的全局解释器锁(GIL),在CPU密集型任务中,线程可能不会提高性能。对于这类任务,使用多进程(例如multiprocessing
模块)可能更合适。
如何在类中管理多个线程的生命周期?
管理多个线程的生命周期可以通过维护线程的列表来实现。您可以启动多个线程,并在完成所有线程的工作后使用join()
方法等待它们结束。例如:
threads = []
for i in range(5):
thread = threading.Thread(target=my_instance.my_function)
threads.append(thread)
thread.start()
for thread in threads:
thread.join()
这样可以确保所有线程完成执行后,主程序再继续进行后续操作。
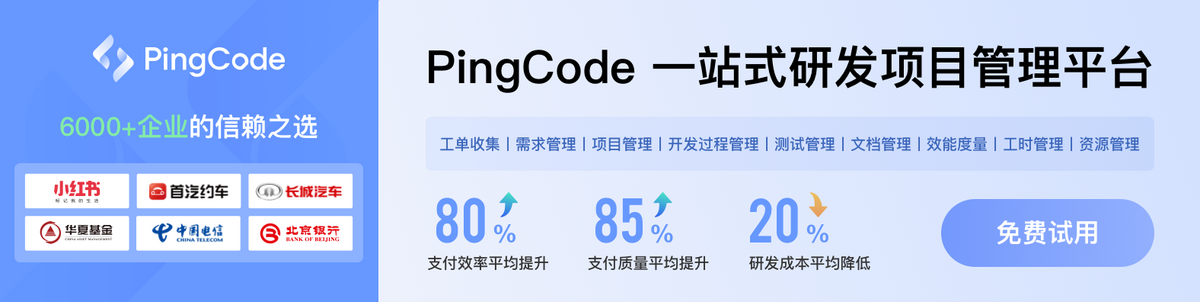