并发运行两个软件的常用方法包括使用多线程、多进程、以及异步编程。 其中,使用多线程和多进程是最常见的方法。在Python中,可以通过threading
模块和multiprocessing
模块来实现多线程和多进程编程。接下来我们将详细介绍这些方法。
一、使用多线程
多线程是一种轻量级的并行执行方式,适用于I/O密集型任务。在Python中,可以使用threading
模块来创建和管理线程。
1、创建线程
可以通过继承threading.Thread
类并重写run
方法来创建线程。下面是一个示例代码:
import threading
import time
def run_software1():
print("Software 1 is running")
time.sleep(5)
print("Software 1 has completed")
def run_software2():
print("Software 2 is running")
time.sleep(5)
print("Software 2 has completed")
创建线程
thread1 = threading.Thread(target=run_software1)
thread2 = threading.Thread(target=run_software2)
启动线程
thread1.start()
thread2.start()
等待线程完成
thread1.join()
thread2.join()
print("Both software have completed")
在这个示例中,我们定义了两个函数run_software1
和run_software2
,分别模拟两个软件的运行。然后,我们创建了两个线程thread1
和thread2
,并启动它们。最后,我们使用join
方法等待两个线程完成。
2、线程同步
当多个线程访问共享资源时,需要进行同步以避免竞争条件。可以使用threading
模块中的Lock
对象来实现线程同步。
import threading
import time
lock = threading.Lock()
def run_software1():
with lock:
print("Software 1 is running")
time.sleep(5)
print("Software 1 has completed")
def run_software2():
with lock:
print("Software 2 is running")
time.sleep(5)
print("Software 2 has completed")
创建线程
thread1 = threading.Thread(target=run_software1)
thread2 = threading.Thread(target=run_software2)
启动线程
thread1.start()
thread2.start()
等待线程完成
thread1.join()
thread2.join()
print("Both software have completed")
在这个示例中,我们使用了Lock
对象来同步线程。每个线程在访问共享资源时,首先获取锁,完成操作后释放锁,以确保多个线程不会同时访问共享资源。
二、使用多进程
多进程是一种重量级的并行执行方式,适用于CPU密集型任务。在Python中,可以使用multiprocessing
模块来创建和管理进程。
1、创建进程
可以通过继承multiprocessing.Process
类并重写run
方法来创建进程。下面是一个示例代码:
import multiprocessing
import time
def run_software1():
print("Software 1 is running")
time.sleep(5)
print("Software 1 has completed")
def run_software2():
print("Software 2 is running")
time.sleep(5)
print("Software 2 has completed")
创建进程
process1 = multiprocessing.Process(target=run_software1)
process2 = multiprocessing.Process(target=run_software2)
启动进程
process1.start()
process2.start()
等待进程完成
process1.join()
process2.join()
print("Both software have completed")
在这个示例中,我们定义了两个函数run_software1
和run_software2
,分别模拟两个软件的运行。然后,我们创建了两个进程process1
和process2
,并启动它们。最后,我们使用join
方法等待两个进程完成。
2、进程间通信
在多进程编程中,进程间通信(IPC)是一个重要的概念。可以使用multiprocessing
模块中的Queue
对象来实现进程间通信。
import multiprocessing
import time
def run_software1(queue):
print("Software 1 is running")
time.sleep(5)
print("Software 1 has completed")
queue.put("Software 1 result")
def run_software2(queue):
print("Software 2 is running")
time.sleep(5)
print("Software 2 has completed")
queue.put("Software 2 result")
创建队列
queue = multiprocessing.Queue()
创建进程
process1 = multiprocessing.Process(target=run_software1, args=(queue,))
process2 = multiprocessing.Process(target=run_software2, args=(queue,))
启动进程
process1.start()
process2.start()
等待进程完成
process1.join()
process2.join()
获取结果
result1 = queue.get()
result2 = queue.get()
print("Both software have completed")
print(f"Results: {result1}, {result2}")
在这个示例中,我们使用Queue
对象来实现进程间通信。每个进程在完成任务后,将结果放入队列中。主进程等待两个进程完成后,从队列中获取结果并打印。
三、使用异步编程
异步编程是一种高效的并行执行方式,适用于I/O密集型任务。在Python中,可以使用asyncio
模块来实现异步编程。
1、定义异步函数
可以使用async def
关键字定义异步函数,并使用await
关键字等待异步操作完成。下面是一个示例代码:
import asyncio
async def run_software1():
print("Software 1 is running")
await asyncio.sleep(5)
print("Software 1 has completed")
async def run_software2():
print("Software 2 is running")
await asyncio.sleep(5)
print("Software 2 has completed")
async def main():
await asyncio.gather(run_software1(), run_software2())
运行异步任务
asyncio.run(main())
print("Both software have completed")
在这个示例中,我们定义了两个异步函数run_software1
和run_software2
,分别模拟两个软件的运行。然后,我们使用asyncio.gather
并发地运行这两个异步函数。最后,我们使用asyncio.run
运行主异步函数main
。
2、异步I/O操作
在异步编程中,I/O操作通常是异步的,可以使用await
关键字等待I/O操作完成。
import asyncio
async def run_software1():
print("Software 1 is running")
await asyncio.sleep(5)
print("Software 1 has completed")
async def run_software2():
print("Software 2 is running")
await asyncio.sleep(5)
print("Software 2 has completed")
async def main():
await asyncio.gather(run_software1(), run_software2())
运行异步任务
asyncio.run(main())
print("Both software have completed")
在这个示例中,我们使用了asyncio.sleep
函数来模拟异步I/O操作。await
关键字用于等待异步操作完成。
四、总结
通过以上介绍,我们可以看到,Python提供了多种并发运行两个软件的方法,包括多线程、多进程和异步编程。不同的方法适用于不同的场景:
- 多线程:适用于I/O密集型任务,可以通过
threading
模块创建和管理线程。 - 多进程:适用于CPU密集型任务,可以通过
multiprocessing
模块创建和管理进程。 - 异步编程:适用于I/O密集型任务,可以通过
asyncio
模块实现异步编程。
根据具体需求选择合适的方法,并合理管理线程、进程和异步任务,可以有效提高程序的并发执行效率。
相关问答FAQs:
如何使用Python实现并发运行多个软件?
要实现并发运行两个软件,可以使用Python的subprocess
模块配合threading
或multiprocessing
模块。使用subprocess
模块可以启动外部软件,而通过threading
或multiprocessing
模块可以实现真正的并发执行。具体步骤包括导入所需模块、定义启动函数以及创建线程或进程来执行软件。
我需要安装哪些库或模块来支持并发运行软件?
Python自带的标准库已经包含了subprocess
、threading
和multiprocessing
模块,因此不需要额外安装任何库。只需确保你的Python环境是最新版本,以便使用这些模块的最新特性和功能。
在并发运行软件时,我应该考虑哪些潜在的问题?
在并发运行软件时,可能会遇到资源竞争、死锁、内存管理等问题。确保每个进程或线程独立运行,避免共享全局变量,以减少冲突。此外,监控系统资源的使用情况,以防止因资源不足导致的性能下降。适当的异常处理机制也是必要的,以保证程序的稳定性。
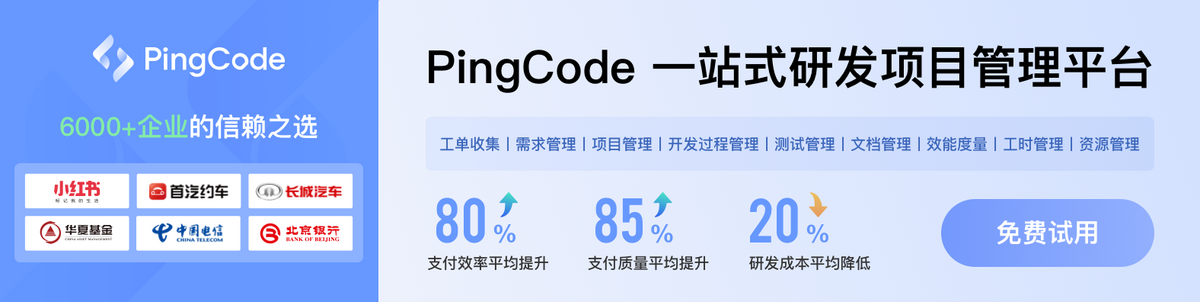