在Python中,放大柱状图的方法包括调整图形大小、修改坐标轴范围、增加数据间距、调整条形宽度等。 其中,调整图形大小是最常用的方式,通过改变图形的尺寸,可以更清晰地展示数据。本文将详细讲解这些方法及其实现方式。
一、调整图形大小
调整图形大小是放大柱状图最直接的方法。使用 matplotlib
库中的 figure
函数可以轻松实现这一点。
import matplotlib.pyplot as plt
Example data
labels = ['A', 'B', 'C', 'D']
values = [10, 20, 15, 25]
Create a figure with a specified size
plt.figure(figsize=(10, 6))
Plot the bar chart
plt.bar(labels, values)
Show the plot
plt.show()
在上述代码中,figsize
参数用于设置图形的宽度和高度,单位为英寸。通过调整 figsize
的值,可以将柱状图放大到所需的尺寸。
二、修改坐标轴范围
调整坐标轴范围也是一种放大柱状图的方法。通过改变坐标轴的范围,可以更清晰地展示特定区域的数据。
import matplotlib.pyplot as plt
Example data
labels = ['A', 'B', 'C', 'D']
values = [10, 20, 15, 25]
Create a figure
plt.figure(figsize=(10, 6))
Plot the bar chart
plt.bar(labels, values)
Set x and y axis limits
plt.xlim(-1, 4)
plt.ylim(0, 30)
Show the plot
plt.show()
在上述代码中,xlim
和 ylim
函数用于设置 x 轴和 y 轴的范围。通过调整这些范围,可以放大柱状图的特定区域。
三、增加数据间距
增加数据间距可以使柱状图更易于阅读,尤其是在数据点较多的情况下。
import matplotlib.pyplot as plt
import numpy as np
Example data
labels = ['A', 'B', 'C', 'D']
values = [10, 20, 15, 25]
Create a figure
plt.figure(figsize=(10, 6))
Create bar positions with increased spacing
positions = np.arange(len(labels)) * 1.5
Plot the bar chart
plt.bar(positions, values, tick_label=labels)
Show the plot
plt.show()
在上述代码中,np.arange
函数用于创建数据点的位置,* 1.5
用于增加数据点之间的间距。通过调整数据点的位置,可以使柱状图更加清晰。
四、调整条形宽度
调整条形宽度可以改变柱状图的视觉效果。通过增加条形宽度,可以使柱状图显得更加紧凑。
import matplotlib.pyplot as plt
Example data
labels = ['A', 'B', 'C', 'D']
values = [10, 20, 15, 25]
Create a figure
plt.figure(figsize=(10, 6))
Plot the bar chart with adjusted width
plt.bar(labels, values, width=0.5)
Show the plot
plt.show()
在上述代码中,width
参数用于设置条形的宽度。通过调整 width
的值,可以改变柱状图的视觉效果。
五、使用Seaborn库
Seaborn
是基于 matplotlib
的高级数据可视化库,它提供了更简洁的接口来创建漂亮的图表。使用 Seaborn
可以更容易地放大柱状图。
import seaborn as sns
import matplotlib.pyplot as plt
Example data
data = {'Labels': ['A', 'B', 'C', 'D'], 'Values': [10, 20, 15, 25]}
Create a figure
plt.figure(figsize=(10, 6))
Plot the bar chart using Seaborn
sns.barplot(x='Labels', y='Values', data=data)
Show the plot
plt.show()
在上述代码中,sns.barplot
函数用于创建柱状图,并且可以通过设置 figsize
参数来放大图形。
六、添加标题和标签
为柱状图添加标题和标签可以使图表更加专业和易于理解。
import matplotlib.pyplot as plt
Example data
labels = ['A', 'B', 'C', 'D']
values = [10, 20, 15, 25]
Create a figure
plt.figure(figsize=(10, 6))
Plot the bar chart
plt.bar(labels, values)
Add title and labels
plt.title('Sample Bar Chart')
plt.xlabel('Categories')
plt.ylabel('Values')
Show the plot
plt.show()
在上述代码中,title
、xlabel
和 ylabel
函数用于添加标题和标签。通过添加这些元素,可以使柱状图更具信息性。
七、调整字体大小
调整字体大小可以提高图表的可读性,尤其是在放大图形时。
import matplotlib.pyplot as plt
Example data
labels = ['A', 'B', 'C', 'D']
values = [10, 20, 15, 25]
Create a figure
plt.figure(figsize=(10, 6))
Plot the bar chart
plt.bar(labels, values)
Add title and labels with adjusted font size
plt.title('Sample Bar Chart', fontsize=20)
plt.xlabel('Categories', fontsize=15)
plt.ylabel('Values', fontsize=15)
Show the plot
plt.show()
在上述代码中,fontsize
参数用于设置标题和标签的字体大小。通过调整 fontsize
的值,可以提高图表的可读性。
八、使用网格线
添加网格线可以帮助读者更容易地理解数据。
import matplotlib.pyplot as plt
Example data
labels = ['A', 'B', 'C', 'D']
values = [10, 20, 15, 25]
Create a figure
plt.figure(figsize=(10, 6))
Plot the bar chart
plt.bar(labels, values)
Add grid lines
plt.grid(True)
Show the plot
plt.show()
在上述代码中,grid
函数用于添加网格线。通过添加网格线,可以使数据更加易于阅读。
九、使用不同颜色和样式
使用不同的颜色和样式可以使柱状图更具吸引力和辨识度。
import matplotlib.pyplot as plt
Example data
labels = ['A', 'B', 'C', 'D']
values = [10, 20, 15, 25]
Create a figure
plt.figure(figsize=(10, 6))
Plot the bar chart with different colors
plt.bar(labels, values, color=['red', 'blue', 'green', 'orange'])
Show the plot
plt.show()
在上述代码中,color
参数用于设置条形的颜色。通过使用不同的颜色,可以使柱状图更具视觉吸引力。
十、使用堆叠柱状图
堆叠柱状图可以在同一图表中显示多组数据,提供更全面的信息。
import matplotlib.pyplot as plt
Example data
labels = ['A', 'B', 'C', 'D']
values1 = [10, 20, 15, 25]
values2 = [5, 10, 7, 12]
Create a figure
plt.figure(figsize=(10, 6))
Plot the bar chart
plt.bar(labels, values1, label='Group 1')
plt.bar(labels, values2, bottom=values1, label='Group 2')
Add legend
plt.legend()
Show the plot
plt.show()
在上述代码中,bottom
参数用于设置堆叠条形的底部位置。通过创建堆叠柱状图,可以在同一图表中显示多组数据。
总结
放大柱状图的方法有很多,包括调整图形大小、修改坐标轴范围、增加数据间距、调整条形宽度、使用 Seaborn
库、添加标题和标签、调整字体大小、使用网格线、使用不同颜色和样式以及创建堆叠柱状图等。通过结合这些方法,可以创建更加清晰、专业和易于理解的柱状图。
相关问答FAQs:
如何在Python中调整柱状图的大小?
在Python中,可以使用Matplotlib库来绘制柱状图并调整其大小。可以通过figsize
参数在创建图形时指定图的宽度和高度,例如:
import matplotlib.pyplot as plt
plt.figure(figsize=(10, 6)) # 设置图形大小为10x6英寸
plt.bar(x, y)
plt.show()
这将使柱状图的尺寸更符合您的需求。
可以使用哪些库来放大柱状图?
除了Matplotlib,Seaborn和Plotly等库也提供了绘制柱状图的功能。Seaborn构建于Matplotlib之上,提供了更高层次的接口,而Plotly则可以创建交互式图形。通过设置相应的参数,您可以在这些库中调整柱状图的大小。
放大柱状图时需要注意哪些细节?
在放大柱状图时,确保数据的可读性是关键。调整图形尺寸后,可能需要重新设置坐标轴标签、标题和图例的位置,以保持图表的整洁。同时,考虑图形的分辨率,确保在不同设备上查看时,图形的清晰度和可读性不会受到影响。
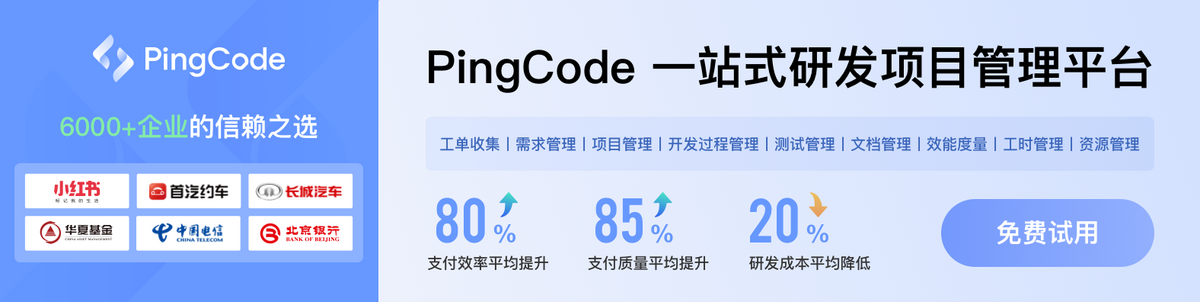