Python 3 查询可调用函数的方法
在Python 3中,查询可调用函数的方法有多种,如使用callable()
函数、通过dir()
函数查看对象的方法、使用反射模块inspect
等。下面将详细介绍这些方法,并以实际示例来演示它们的应用。
一、使用callable()
函数
callable()
函数是Python内置的一个函数,它用于检查一个对象是否是可调用的。如果对象是可调用的,callable()
函数返回True
,否则返回False
。可调用的对象包括函数、方法、类等。
示例代码
def my_function():
print("Hello, World!")
print(callable(my_function)) # 输出: True
my_variable = 42
print(callable(my_variable)) # 输出: False
在这个示例中,my_function
是一个函数对象,因此callable(my_function)
返回True
。而my_variable
是一个整数对象,不是可调用的,因此callable(my_variable)
返回False
。
二、通过dir()
函数查看对象的方法
dir()
函数是Python内置的另一个函数,它返回一个列表,包含一个对象的所有属性和方法。通过查看这个列表,我们可以知道该对象有哪些方法是可调用的。
示例代码
class MyClass:
def method1(self):
print("Method1")
def method2(self):
print("Method2")
obj = MyClass()
methods = [attr for attr in dir(obj) if callable(getattr(obj, attr))]
print(methods) # 输出: ['method1', 'method2']
在这个示例中,我们定义了一个类MyClass
,它包含两个方法method1
和method2
。我们创建了一个MyClass
的实例obj
,并使用dir(obj)
获取obj
的所有属性和方法。然后,我们使用列表推导式过滤出所有可调用的方法。
三、使用反射模块inspect
inspect
模块提供了几个有用的函数来获取对象的信息,其中包括getmembers()
和ismethod()
函数。inspect.getmembers()
返回对象的所有成员,而inspect.ismethod()
检查一个成员是否是方法。
示例代码
import inspect
class MyClass:
def method1(self):
print("Method1")
def method2(self):
print("Method2")
obj = MyClass()
methods = [name for name, member in inspect.getmembers(obj, inspect.ismethod)]
print(methods) # 输出: ['method1', 'method2']
在这个示例中,我们使用inspect.getmembers()
获取obj
的所有成员,并使用inspect.ismethod()
过滤出所有方法。
四、综合示例
下面是一个综合示例,演示如何使用上述方法查询一个类的所有可调用函数:
import inspect
class MyClass:
def __init__(self):
pass
def method1(self):
print("Method1")
def method2(self):
print("Method2")
@staticmethod
def static_method():
print("Static Method")
@classmethod
def class_method(cls):
print("Class Method")
使用 callable() 函数
obj = MyClass()
print(callable(obj.method1)) # 输出: True
print(callable(obj.static_method)) # 输出: True
使用 dir() 函数
methods = [attr for attr in dir(obj) if callable(getattr(obj, attr))]
print(methods) # 输出: ['class_method', 'method1', 'method2', 'static_method']
使用 inspect 模块
methods = [name for name, member in inspect.getmembers(obj, inspect.ismethod)]
print(methods) # 输出: ['class_method', 'method1', 'method2']
获取静态方法和类方法
static_methods = [name for name, member in inspect.getmembers(MyClass, inspect.isfunction) if not name.startswith('__')]
print(static_methods) # 输出: ['class_method', 'static_method']
在这个综合示例中,我们定义了一个类MyClass
,它包含普通方法、静态方法和类方法。我们演示了如何使用callable()
、dir()
和inspect
模块查询该类的所有可调用函数。
五、总结
在Python 3中,查询可调用函数的方法有多种,包括使用callable()
函数、通过dir()
函数查看对象的方法、使用反射模块inspect
等。每种方法都有其独特的优点和应用场景。通过结合使用这些方法,我们可以全面地了解一个对象的可调用属性和方法,并在实际编程中灵活应用它们。
相关问答FAQs:
如何在Python 3中列出当前模块中的所有可调用函数?
在Python 3中,可以使用内置的dir()
函数结合inspect
模块来列出当前模块中的所有可调用函数。首先,导入inspect
模块,然后通过inspect.getmembers()
函数过滤出可调用对象。示例代码如下:
import inspect
def sample_function():
pass
class SampleClass:
def method(self):
pass
callable_functions = [func for func in inspect.getmembers(__import__(__name__), predicate=inspect.isfunction)]
print(callable_functions)
Python 3中如何判断一个对象是否为可调用函数?
可以使用内置的callable()
函数来判断一个对象是否为可调用函数。这个函数会返回布尔值,指示对象是否可以被调用。示例代码如下:
def my_function():
return "Hello, World!"
print(callable(my_function)) # 输出: True
print(callable(5)) # 输出: False
在Python 3中如何通过模块名称查询可调用函数?
可以使用importlib
模块动态导入模块,并结合inspect
模块查询其可调用函数。首先,使用importlib.import_module()
导入指定的模块,然后使用inspect.getmembers()
获取可调用函数。示例代码如下:
import importlib
import inspect
module_name = 'math' # 假设要查询math模块
module = importlib.import_module(module_name)
callable_functions = [func for func in inspect.getmembers(module, predicate=inspect.isfunction)]
print(callable_functions)
这些方法可以帮助你在Python 3中有效地查询和识别可调用函数。
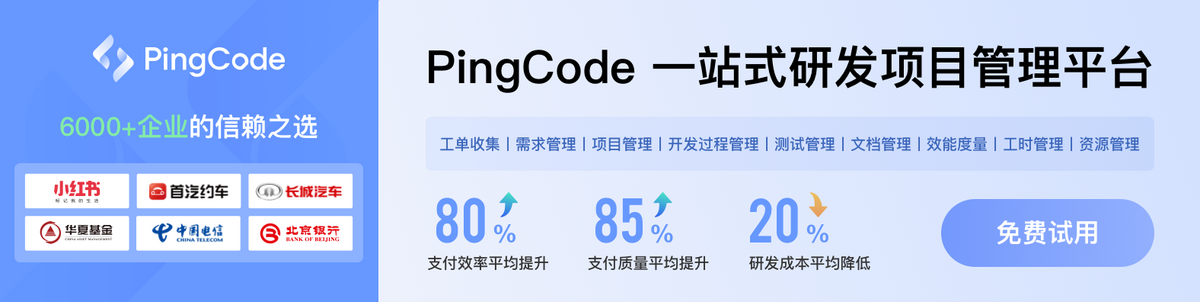