如何用Python爬百度文库
爬取百度文库内容,可以通过以下步骤:使用requests模块发送请求获取网页HTML内容、使用BeautifulSoup解析HTML文档、模拟登录获取权限、处理JavaScript渲染页面。下面我们将详细介绍这些步骤。
一、使用requests模块发送请求获取网页HTML内容
首先,我们需要使用requests模块发送请求来获取百度文库页面的HTML内容。requests模块是Python中一个简单易用的HTTP请求库,可以方便地发送HTTP请求并获取响应。
import requests
def get_html_content(url):
headers = {
'User-Agent': 'Mozilla/5.0 (Windows NT 10.0; Win64; x64) AppleWebKit/537.36 (KHTML, like Gecko) Chrome/58.0.3029.110 Safari/537.3'}
response = requests.get(url, headers=headers)
if response.status_code == 200:
return response.text
else:
return None
url = 'https://wenku.baidu.com/view/some_document.html'
html_content = get_html_content(url)
print(html_content)
使用requests模块发送请求获取网页HTML内容:我们需要指定请求头(headers)来模拟浏览器行为,以防止被百度文库检测到是爬虫请求。
二、使用BeautifulSoup解析HTML文档
获取到HTML内容后,我们需要使用BeautifulSoup模块来解析HTML文档。BeautifulSoup是一个用于解析HTML和XML文档的Python库,可以方便地提取网页中的数据。
from bs4 import BeautifulSoup
def parse_html_content(html_content):
soup = BeautifulSoup(html_content, 'html.parser')
# Extract specific content based on the structure of the HTML document
title = soup.find('title').get_text()
paragraphs = soup.find_all('p')
document_content = []
for paragraph in paragraphs:
document_content.append(paragraph.get_text())
return title, document_content
title, document_content = parse_html_content(html_content)
print(f"Title: {title}")
print("Content:")
for paragraph in document_content:
print(paragraph)
使用BeautifulSoup解析HTML文档:我们可以根据HTML文档的结构提取所需的内容,例如文档标题和段落内容。
三、模拟登录获取权限
有些百度文库文档需要登录才能查看全部内容。因此,我们需要模拟登录百度账号,以获取查看文档的权限。模拟登录通常需要使用会话(Session)对象来保持登录状态。
def login_baidu(username, password):
session = requests.Session()
login_url = 'https://passport.baidu.com/v2/?login'
payload = {
'username': username,
'password': password,
# Add other required login parameters
}
response = session.post(login_url, data=payload)
if response.status_code == 200 and '成功' in response.text:
return session
else:
return None
username = 'your_username'
password = 'your_password'
session = login_baidu(username, password)
if session:
url = 'https://wenku.baidu.com/view/some_document.html'
html_content = session.get(url).text
title, document_content = parse_html_content(html_content)
print(f"Title: {title}")
print("Content:")
for paragraph in document_content:
print(paragraph)
else:
print("Login failed")
模拟登录获取权限:我们需要使用会话对象来保持登录状态,并在发送请求时携带登录后的Cookie信息。
四、处理JavaScript渲染页面
有些百度文库页面是通过JavaScript渲染的,requests模块无法直接获取到完整的内容。这种情况下,我们需要使用Selenium等浏览器自动化工具来模拟浏览器行为,获取完整的页面内容。
from selenium import webdriver
from selenium.webdriver.common.by import By
from selenium.webdriver.chrome.service import Service
from selenium.webdriver.chrome.options import Options
def get_full_page_content(url):
chrome_options = Options()
chrome_options.add_argument('--headless')
chrome_options.add_argument('--disable-gpu')
service = Service('path_to_chromedriver')
driver = webdriver.Chrome(service=service, options=chrome_options)
driver.get(url)
# Wait for the page to fully load
driver.implicitly_wait(10)
full_page_content = driver.page_source
driver.quit()
return full_page_content
url = 'https://wenku.baidu.com/view/some_document.html'
full_page_content = get_full_page_content(url)
title, document_content = parse_html_content(full_page_content)
print(f"Title: {title}")
print("Content:")
for paragraph in document_content:
print(paragraph)
处理JavaScript渲染页面:通过使用Selenium等浏览器自动化工具,我们可以模拟浏览器行为,等待页面完全加载后获取完整的页面内容。
五、保存和处理爬取的数据
在成功爬取到百度文库的内容后,我们可能需要将这些数据保存到本地文件或数据库中,以便后续处理和分析。
import json
def save_to_file(title, document_content, filename):
with open(filename, 'w', encoding='utf-8') as f:
data = {
'title': title,
'content': document_content
}
json.dump(data, f, ensure_ascii=False, indent=4)
filename = 'document.json'
save_to_file(title, document_content, filename)
print(f"Document saved to {filename}")
保存和处理爬取的数据:我们可以将爬取到的文档内容保存为JSON文件,以便后续处理和分析。
总结
通过上述步骤,我们可以使用Python爬取百度文库的内容。首先,我们使用requests模块发送请求获取网页HTML内容,然后使用BeautifulSoup解析HTML文档。对于需要登录的文档,我们可以模拟登录获取权限,并使用会话对象保持登录状态。对于JavaScript渲染的页面,我们可以使用Selenium等浏览器自动化工具来获取完整的页面内容。最后,我们可以将爬取到的数据保存到本地文件或数据库中,以便后续处理和分析。
需要注意的是,爬取百度文库等网站的内容时,请遵守相关法律法规和网站的使用条款,避免对网站造成过大压力或影响他人正常使用。
相关问答FAQs:
如何使用Python获取百度文库中的文档内容?
要获取百度文库中的文档内容,您需要使用Python的网络请求库(如requests)来发送HTTP请求,并解析返回的HTML内容。可以使用Beautiful Soup等库来提取所需的信息。另外,您可能需要处理页面中的JavaScript内容或使用代理来避免被网站封锁。
在爬取百度文库时,如何处理反爬机制?
百度文库可能会采用多种反爬机制,如IP封锁、验证码等。为了应对这些机制,可以考虑使用代理IP轮换,设置请求间隔时间,模拟用户行为(如随机用户代理),并在必要时手动输入验证码。
是否可以使用Python库来简化百度文库的爬取过程?
确实可以借助一些现有的Python库来简化爬取过程。例如,使用Scrapy框架可以快速构建爬虫,而Pyppeteer或Selenium可以处理动态加载的页面,帮助提取需要的内容。这些库提供了丰富的功能,能够提高爬取效率和稳定性。
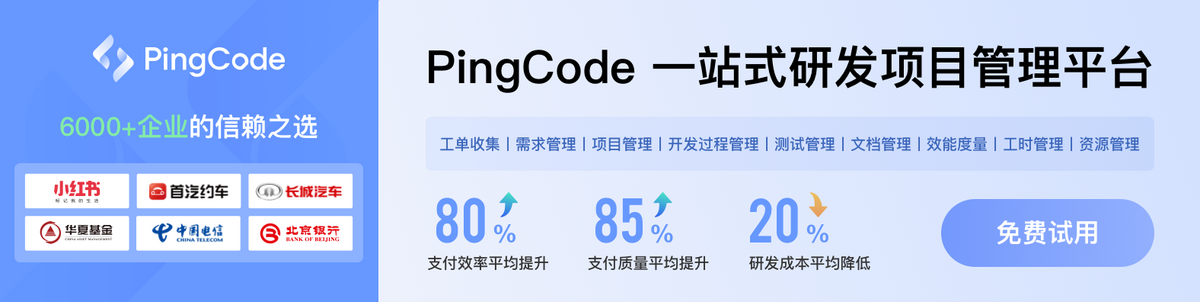