开发个人语音助手Python的关键是使用合适的语音识别库、自然语言处理库和文本转语音工具,常见的包括SpeechRecognition、pyttsx3、pyaudio等。确保语音助手能够准确识别用户的语音命令、进行合理的自然语言处理并做出合适的响应,是开发成功的关键。
详细描述:使用SpeechRecognition库进行语音识别。SpeechRecognition库是Python中最常用的语音识别库之一,它提供了对多种语音识别引擎的支持。该库可以将麦克风捕捉到的语音转换为文本,从而让程序能够识别用户的语音命令。这一步是语音助手中最基础也是最关键的一步。通过合理的训练和优化,SpeechRecognition库可以提高语音识别的准确性和响应速度。
一、准备工作
在开始开发个人语音助手之前,首先需要准备好开发环境和所需的库。下面列出了一些关键步骤:
1、安装Python和必要的库
确保你已经安装了Python(建议使用Python 3.x),并使用pip安装以下库:
pip install SpeechRecognition
pip install pyttsx3
pip install pyaudio
pip install wikipedia
pip install pywhatkit
pip install datetime
pip install requests
2、配置麦克风和扬声器
确保你的开发设备配备了麦克风和扬声器,并且能够正常工作。你可以通过操作系统的设置来测试和调整麦克风和扬声器的音量。
二、语音识别
语音识别是语音助手的核心功能之一。这里我们使用SpeechRecognition库来实现语音识别功能。
1、导入库并初始化语音识别器
首先,导入SpeechRecognition库并初始化语音识别器:
import speech_recognition as sr
初始化语音识别器
recognizer = sr.Recognizer()
2、捕捉和识别语音
接下来,通过麦克风捕捉用户的语音并进行识别:
def listen():
with sr.Microphone() as source:
print("Listening...")
# 调整噪音水平
recognizer.adjust_for_ambient_noise(source)
audio = recognizer.listen(source)
try:
# 识别语音
command = recognizer.recognize_google(audio)
print(f"User said: {command}")
except sr.UnknownValueError:
print("Sorry, I did not understand that.")
except sr.RequestError:
print("Sorry, my speech service is down.")
return command
测试语音识别功能
listen()
三、自然语言处理
自然语言处理(NLP)是语音助手理解用户命令并做出响应的关键。我们可以使用简单的if-else结构来处理命令,或者借助更高级的NLP库如spaCy。
1、基本命令处理
下面是一个处理基本命令的例子:
def handle_command(command):
if 'time' in command:
tell_time()
elif 'date' in command:
tell_date()
elif 'search' in command:
search_wikipedia(command)
elif 'play' in command:
play_youtube(command)
else:
print("Sorry, I didn't understand the command.")
def tell_time():
from datetime import datetime
current_time = datetime.now().strftime("%I:%M %p")
print(f"The time is {current_time}")
def tell_date():
from datetime import datetime
current_date = datetime.now().strftime("%B %d, %Y")
print(f"Today's date is {current_date}")
def search_wikipedia(command):
import wikipedia
query = command.replace("search", "").strip()
result = wikipedia.summary(query, sentences=2)
print(result)
def play_youtube(command):
import pywhatkit
song = command.replace("play", "").strip()
pywhatkit.playonyt(song)
四、文本转语音
为了让语音助手与用户进行语音交流,我们需要一个文本转语音(TTS)引擎。这里我们使用pyttsx3库来实现TTS功能。
1、初始化TTS引擎
首先,导入并初始化pyttsx3库:
import pyttsx3
初始化TTS引擎
engine = pyttsx3.init()
def speak(text):
engine.say(text)
engine.runAndWait()
2、结合TTS和命令处理
将TTS功能集成到命令处理中,使语音助手能够用语音回应用户:
def tell_time():
from datetime import datetime
current_time = datetime.now().strftime("%I:%M %p")
speak(f"The time is {current_time}")
def tell_date():
from datetime import datetime
current_date = datetime.now().strftime("%B %d, %Y")
speak(f"Today's date is {current_date}")
def search_wikipedia(command):
import wikipedia
query = command.replace("search", "").strip()
result = wikipedia.summary(query, sentences=2)
speak(result)
def play_youtube(command):
import pywhatkit
song = command.replace("play", "").strip()
speak(f"Playing {song} on YouTube")
pywhatkit.playonyt(song)
五、主函数
最后,将所有功能集成到主函数中,使语音助手可以持续监听用户命令并进行处理:
def main():
while True:
command = listen()
handle_command(command)
if __name__ == "__main__":
main()
六、优化和增强功能
在基本功能实现后,可以进一步优化和增强语音助手的功能。例如:
1、添加更多命令
你可以添加更多的命令处理函数,例如天气查询、闹钟设置、邮件发送等:
def check_weather():
import requests
api_key = "your_api_key"
location = "your_location"
url = f"http://api.openweathermap.org/data/2.5/weather?q={location}&appid={api_key}"
response = requests.get(url).json()
weather = response['weather'][0]['description']
temp = response['main']['temp']
speak(f"The weather in {location} is {weather} with a temperature of {temp} degrees Celsius.")
2、优化语音识别
通过调整SpeechRecognition库的参数、使用不同的语音识别引擎或训练自定义模型,可以提高语音识别的准确性:
# 使用不同的语音识别引擎
recognizer.recognize_sphinx(audio)
3、提高响应速度
通过优化代码、减少不必要的计算和调用,可以提高语音助手的响应速度。例如,调整音频录制和处理的参数:
audio = recognizer.listen(source, timeout=5, phrase_time_limit=10)
七、总结
开发个人语音助手Python的过程包括语音识别、自然语言处理和文本转语音三个主要部分。通过合理配置和优化这些部分,可以构建一个功能强大、响应迅速的语音助手。除了基础功能之外,还可以通过添加更多命令和功能、优化语音识别和提高响应速度来进一步提升语音助手的性能和用户体验。
相关问答FAQs:
如何开始开发个人语音助手的基础知识?
在开发个人语音助手之前,了解一些基础知识是非常重要的。您需要掌握Python编程语言的基本概念,包括数据结构、控制流和函数。同时,熟悉一些常用的库,如SpeechRecognition、gTTS(Google Text-to-Speech)和PyAudio,可以帮助您在项目中实现语音识别和语音合成功能。
在开发过程中如何处理语音识别的准确性问题?
语音识别的准确性会受到多种因素的影响,包括环境噪声、用户的口音以及语速等。为提高识别准确性,您可以考虑使用高质量的麦克风设备,选择适合您的项目需求的语音识别库,甚至可以训练自己的模型以适应特定用户的发音。此外,加入一些数据预处理步骤,例如消除背景噪音,也能显著改善识别效果。
如何为我的语音助手添加个性化功能?
个性化功能可以通过收集用户的偏好和历史数据来实现。例如,您可以设计一个简单的数据库来存储用户的常用指令、喜好设置和日常任务。根据这些数据,助手可以提供更贴合用户需求的服务,如推荐音乐、提醒事项或定制化的日程安排。通过持续学习用户的行为,您的助手将变得愈发智能。
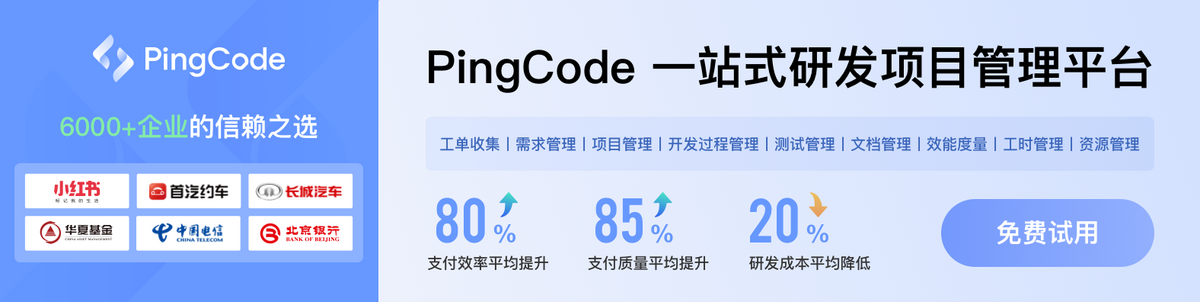