在Python3中复制文件的方法有多种,如使用shutil模块、使用os模块、使用pandas模块等。推荐使用shutil模块,因为它提供了简洁且高效的方法来进行文件复制操作。 其中,shutil模块的copy
和copy2
方法是最常用的。下面将详细介绍如何使用shutil模块来复制文件。
一、使用shutil模块
1、shutil.copy
shutil.copy
是一个简单且常用的方法,用于复制文件。它不仅复制文件内容,还复制权限位。
import shutil
source = 'source_file.txt'
destination = 'destination_file.txt'
shutil.copy(source, destination)
在上面的代码中,source_file.txt
是源文件路径,destination_file.txt
是目标文件路径。shutil.copy
会将源文件复制到目标文件。
2、shutil.copy2
shutil.copy2
除了复制文件内容和权限外,还复制文件的元数据(如文件的创建时间、修改时间等)。
import shutil
source = 'source_file.txt'
destination = 'destination_file.txt'
shutil.copy2(source, destination)
使用 shutil.copy2
可以保留更多的文件属性信息。
二、使用os模块
os
模块提供了低级别的文件操作方法,适合于更复杂的文件操作需求。以下是使用 os
模块复制文件的方法:
import os
source = 'source_file.txt'
destination = 'destination_file.txt'
with open(source, 'rb') as src_file:
with open(destination, 'wb') as dst_file:
dst_file.write(src_file.read())
这种方法适用于简单的文件复制需求,但不如 shutil
模块方便。
三、使用pandas模块
如果要复制的是数据文件(如csv文件),可以使用 pandas
模块来读取和写入文件内容:
import pandas as pd
source = 'source_file.csv'
destination = 'destination_file.csv'
data = pd.read_csv(source)
data.to_csv(destination, index=False)
这种方法适用于数据文件的复制,同时还可以进行数据预处理等操作。
四、使用Pathlib模块
pathlib
模块是Python 3.4引入的新的文件路径操作模块,提供了面向对象的路径操作方法。可以结合 shutil
模块使用:
from pathlib import Path
import shutil
source = Path('source_file.txt')
destination = Path('destination_file.txt')
shutil.copy(source, destination)
pathlib
模块使路径操作更加直观和简洁。
五、错误处理和异常捕获
在进行文件复制操作时,常常需要处理可能发生的错误和异常。以下是一个包含错误处理的文件复制示例:
import shutil
import os
source = 'source_file.txt'
destination = 'destination_file.txt'
try:
if os.path.exists(source):
shutil.copy(source, destination)
print(f'File copied successfully from {source} to {destination}')
else:
print(f'Source file {source} does not exist')
except PermissionError:
print(f'Permission denied: unable to copy {source} to {destination}')
except FileNotFoundError:
print(f'File not found: {source}')
except Exception as e:
print(f'An error occurred: {e}')
在这个示例中,使用 try
和 except
语句捕获并处理了文件复制过程中可能发生的各种异常。
六、总结
在Python3中复制文件的方法有多种,其中 shutil
模块提供了最简洁和高效的方法。shutil.copy
和 shutil.copy2
是最常用的文件复制方法,此外还可以使用 os
模块进行低级别的文件操作,使用 pandas
模块复制数据文件,或者结合 pathlib
模块进行路径操作。无论使用哪种方法,都应注意处理可能发生的错误和异常,以确保文件复制操作的可靠性和稳定性。
通过这些方法,您可以根据具体需求选择最适合的文件复制方式,提高开发效率和代码的可读性。
相关问答FAQs:
在Python3中,复制文件的最佳方法是什么?
在Python3中,复制文件的常用方法是使用shutil
模块中的shutil.copy()
函数。该函数不仅可以复制文件内容,还可以保留文件的权限。使用示例代码如下:
import shutil
shutil.copy('源文件路径', '目标文件路径')
确保提供的路径是有效的,并且目标路径所在的目录存在,以避免错误。
使用Python3复制文件时,如何处理文件不存在的情况?
在复制文件时,如果源文件不存在,程序将抛出FileNotFoundError
。可以通过使用os.path.exists()
函数来检查文件是否存在,从而避免程序崩溃。示例代码如下:
import os
import shutil
if os.path.exists('源文件路径'):
shutil.copy('源文件路径', '目标文件路径')
else:
print("源文件不存在,请检查路径。")
这种方法可以确保在执行复制操作之前验证文件的存在性。
在Python3中,复制文件时如何保持文件的元数据?
如果希望在复制文件时保留更多的元数据(例如时间戳),可以使用shutil.copy2()
函数。这个函数不仅会复制文件内容,还会复制文件的所有元数据。示例代码如下:
import shutil
shutil.copy2('源文件路径', '目标文件路径')
使用shutil.copy2()
可以确保文件的创建时间和修改时间等信息也被保留。
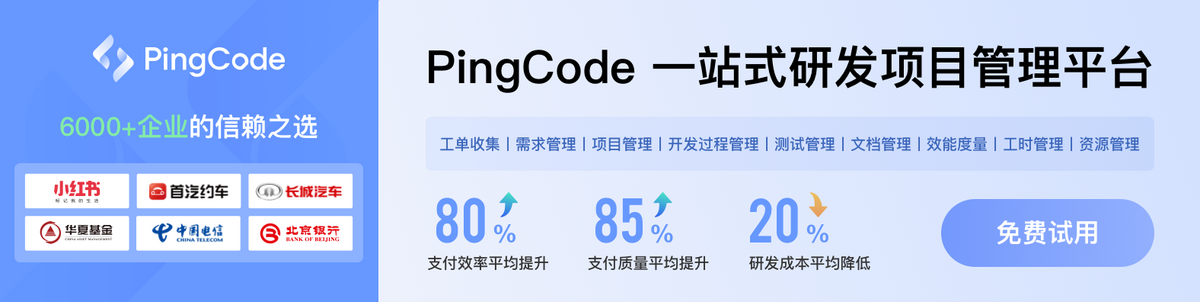