使用Python循环遍历文件夹中的图片,可以通过使用os库、glob库、或者pathlib库等来实现。首先,我们使用os库来遍历文件夹中的所有图片文件。其次,可以使用glob库来匹配特定的文件模式。最后,pathlib库提供了一种面向对象的方式来处理文件系统路径。接下来,我们将详细讲解如何使用这三种方法来遍历文件夹中的图片。
一、使用os库遍历文件夹中的图片
使用os库可以遍历文件夹中的所有文件,并通过检查文件扩展名来确定哪些文件是图片。以下是一个示例:
import os
def traverse_images_with_os(directory):
for root, dirs, files in os.walk(directory):
for file in files:
if file.lower().endswith(('.png', '.jpg', '.jpeg', '.gif', '.bmp')):
print(os.path.join(root, file))
示例用法
traverse_images_with_os('/path/to/your/directory')
os.walk(directory) 生成目录树下的所有目录和文件名,检查文件扩展名来判断是否是图片文件。
二、使用glob库遍历文件夹中的图片
glob库允许使用通配符模式来匹配文件名,方便地找到特定类型的文件。以下是一个示例:
import glob
def traverse_images_with_glob(directory):
image_files = glob.glob(os.path.join(directory, '', '*.[pP][nN][gG]'), recursive=True)
image_files.extend(glob.glob(os.path.join(directory, '', '*.[jJ][pP][gG]'), recursive=True))
image_files.extend(glob.glob(os.path.join(directory, '', '*.[jJ][pP][eE][gG]'), recursive=True))
image_files.extend(glob.glob(os.path.join(directory, '', '*.[gG][iI][fF]'), recursive=True))
image_files.extend(glob.glob(os.path.join(directory, '', '*.[bB][mM][pP]'), recursive=True))
for file in image_files:
print(file)
示例用法
traverse_images_with_glob('/path/to/your/directory')
glob.glob(pattern, recursive=True) 可以递归地匹配所有符合特定模式的文件,使用通配符模式来匹配不同扩展名的图片文件。
三、使用pathlib库遍历文件夹中的图片
pathlib库提供了一种面向对象的方式来处理文件系统路径,以下是一个示例:
from pathlib import Path
def traverse_images_with_pathlib(directory):
path = Path(directory)
for file in path.rglob('*'):
if file.suffix.lower() in ['.png', '.jpg', '.jpeg', '.gif', '.bmp']:
print(file)
示例用法
traverse_images_with_pathlib('/path/to/your/directory')
pathlib.Path(directory).rglob('*') 递归地遍历目录中的所有文件,并检查文件扩展名来判断是否是图片文件。
四、结合PIL库读取和处理图片
在遍历文件夹中的图片后,可以结合Pillow(PIL)库来读取和处理这些图片。以下是一个示例:
from PIL import Image
import os
def process_images(directory):
for root, dirs, files in os.walk(directory):
for file in files:
if file.lower().endswith(('.png', '.jpg', '.jpeg', '.gif', '.bmp')):
image_path = os.path.join(root, file)
with Image.open(image_path) as img:
print(f'Processing image: {image_path}')
# 在这里可以对图像进行处理,例如调整大小、旋转等
img = img.resize((128, 128))
img.show()
示例用法
process_images('/path/to/your/directory')
PIL.Image.open(image_path) 用于打开图像文件,img.resize((128, 128)) 调整图像大小,img.show() 显示图像。
五、使用多线程提高遍历效率
在遍历和处理大量图片时,可以使用多线程来提高效率。以下是一个示例:
import os
import threading
from PIL import Image
def process_image(image_path):
with Image.open(image_path) as img:
print(f'Processing image: {image_path}')
img = img.resize((128, 128))
img.show()
def traverse_images_with_threads(directory):
for root, dirs, files in os.walk(directory):
for file in files:
if file.lower().endswith(('.png', '.jpg', '.jpeg', '.gif', '.bmp')):
image_path = os.path.join(root, file)
threading.Thread(target=process_image, args=(image_path,)).start()
示例用法
traverse_images_with_threads('/path/to/your/directory')
threading.Thread(target=process_image, args=(image_path,)).start() 创建并启动一个新线程来处理每个图片文件。
六、处理遍历中的异常情况
在遍历和处理图片时,可能会遇到各种异常情况,如文件损坏、权限不足等。可以使用try-except块来处理这些异常。以下是一个示例:
import os
from PIL import Image
def process_images_with_exception_handling(directory):
for root, dirs, files in os.walk(directory):
for file in files:
if file.lower().endswith(('.png', '.jpg', '.jpeg', '.gif', '.bmp')):
image_path = os.path.join(root, file)
try:
with Image.open(image_path) as img:
print(f'Processing image: {image_path}')
img = img.resize((128, 128))
img.show()
except Exception as e:
print(f'Failed to process image {image_path}: {e}')
示例用法
process_images_with_exception_handling('/path/to/your/directory')
try-except块 捕获并处理异常,确保程序在遇到错误时不会崩溃。
七、总结
遍历文件夹中的图片可以通过多种方法来实现,包括使用os库、glob库和pathlib库。结合Pillow库,可以方便地读取和处理图片。为了提高效率,可以使用多线程来并行处理图片文件。在实际应用中,还需要处理各种可能的异常情况,确保程序的健壮性。通过以上方法,可以高效地遍历和处理文件夹中的图片文件。
相关问答FAQs:
如何使用Python读取文件夹中的所有图片?
要读取文件夹中的所有图片,您可以使用os
模块和PIL
库(Python Imaging Library)。首先,确保安装了PIL
(可以通过pip install pillow
命令安装)。接着,您可以使用os.listdir()
方法获取目录中的所有文件,结合PIL
库打开并处理这些图片。例如:
import os
from PIL import Image
folder_path = '您的文件夹路径'
for file_name in os.listdir(folder_path):
if file_name.endswith(('.png', '.jpg', '.jpeg', '.gif')): # 根据需要添加图片格式
img_path = os.path.join(folder_path, file_name)
img = Image.open(img_path)
img.show() # 显示图片
如何在Python中处理每一张图片?
处理每一张图片可以包括多种操作,比如调整大小、转换格式或应用滤镜。利用PIL
库,您可以轻松地进行这些操作。例如,您可以使用resize()
方法调整图片大小:
img = Image.open(img_path)
img_resized = img.resize((800, 600)) # 将图片调整为800x600
img_resized.save('新文件路径') # 保存调整后的图片
如何确保只处理特定类型的图片文件?
在遍历文件时,可以通过文件扩展名来过滤需要处理的图片。可以使用条件语句来检查文件名后缀,以确保只处理支持的图片格式。以下是一个示例:
if file_name.lower().endswith(('.png', '.jpg', '.jpeg', '.gif')):
# 处理图片
以上代码确保只处理以.png
、.jpg
、.jpeg
或.gif
结尾的文件,从而避免错误或不必要的文件处理。
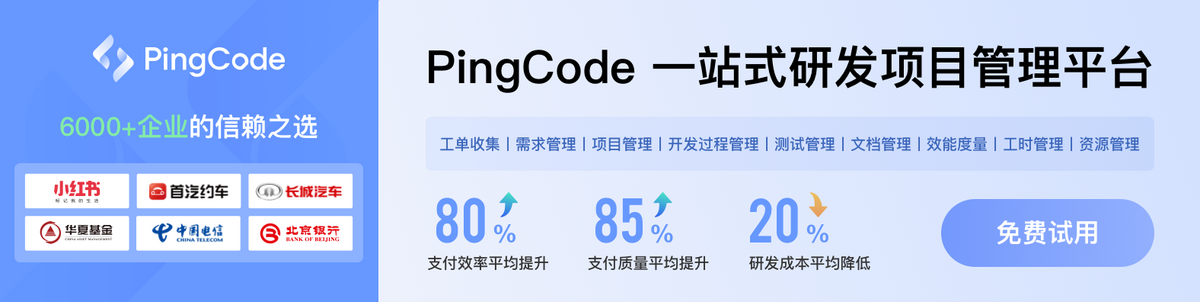