Python下载文件的方法有多种,常用的方法包括使用requests库、urllib库、和wget库。 其中,requests库是最常用且易于使用的。下面将详细介绍如何使用这几种方法来下载文件,并给出每种方法的代码示例。
一、使用requests库
requests库是Python中最常用的HTTP库之一,它使得HTTP请求变得非常简单。使用requests库下载文件的步骤如下:
- 导入requests库。
- 使用requests.get()方法发送HTTP GET请求。
- 检查响应状态码,确保请求成功。
- 以二进制模式打开文件,并将响应内容写入文件中。
import requests
def download_file(url, local_filename):
# 发送HTTP GET请求
response = requests.get(url, stream=True)
# 检查响应状态码
if response.status_code == 200:
with open(local_filename, 'wb') as f:
for chunk in response.iter_content(chunk_size=8192):
f.write(chunk)
else:
print(f"Failed to download file: {response.status_code}")
示例使用
url = 'https://example.com/somefile.zip'
local_filename = 'somefile.zip'
download_file(url, local_filename)
二、使用urllib库
urllib库是Python的标准库之一,提供了操作URL(统一资源定位符)的功能。使用urllib库下载文件的步骤如下:
- 导入urllib.request模块。
- 使用urllib.request.urlretrieve()方法直接下载文件。
import urllib.request
def download_file(url, local_filename):
try:
urllib.request.urlretrieve(url, local_filename)
print(f"Downloaded {local_filename}")
except Exception as e:
print(f"Failed to download file: {e}")
示例使用
url = 'https://example.com/somefile.zip'
local_filename = 'somefile.zip'
download_file(url, local_filename)
三、使用wget库
wget是一个小巧的命令行下载工具,在Python中也有相应的库。使用wget库下载文件的步骤如下:
- 安装wget库(如果未安装)。
- 导入wget模块。
- 使用wget.download()方法下载文件。
import wget
def download_file(url, local_filename):
try:
wget.download(url, local_filename)
print(f"Downloaded {local_filename}")
except Exception as e:
print(f"Failed to download file: {e}")
示例使用
url = 'https://example.com/somefile.zip'
local_filename = 'somefile.zip'
download_file(url, local_filename)
四、下载大文件
对于大文件下载,我们需要考虑内存使用和下载速度,推荐使用requests库的stream模式。下面是一个更完整的示例,展示了如何下载大文件:
import requests
def download_large_file(url, local_filename):
with requests.get(url, stream=True) as r:
r.raise_for_status()
with open(local_filename, 'wb') as f:
for chunk in r.iter_content(chunk_size=8192):
if chunk:
f.write(chunk)
示例使用
url = 'https://example.com/largefile.zip'
local_filename = 'largefile.zip'
download_large_file(url, local_filename)
五、处理下载进度条
为了提升用户体验,可以在下载过程中显示进度条。我们可以使用tqdm库来实现这一功能。以下是一个示例:
import requests
from tqdm import tqdm
def download_file_with_progress(url, local_filename):
response = requests.get(url, stream=True)
total_size = int(response.headers.get('content-length', 0))
block_size = 1024
with open(local_filename, 'wb') as file, tqdm(
desc=local_filename,
total=total_size,
unit='iB',
unit_scale=True,
unit_divisor=1024,
) as bar:
for data in response.iter_content(block_size):
file.write(data)
bar.update(len(data))
示例使用
url = 'https://example.com/largefile.zip'
local_filename = 'largefile.zip'
download_file_with_progress(url, local_filename)
六、总结
Python提供了多种方法来根据链接下载文件,常用的方法包括requests库、urllib库和wget库。requests库由于其简单易用性和强大的功能,是最常用的选择。对于大文件下载,可以使用requests库的stream模式,并结合tqdm库实现下载进度条,以提升用户体验。无论是哪种方法,确保正确处理HTTP响应和异常情况都是非常重要的。通过上述示例代码,您可以根据实际需求选择适合的下载方法。
相关问答FAQs:
如何使用Python下载特定类型的文件?
在Python中,您可以使用内置的requests
库来下载特定类型的文件。例如,如果您想下载一个PDF文件,只需设置请求头并使用requests.get()
方法。以下是一个基本的示例代码:
import requests
url = 'https://example.com/file.pdf'
response = requests.get(url)
with open('file.pdf', 'wb') as file:
file.write(response.content)
确保文件的URL正确无误,并根据需要修改文件名和路径。
下载文件时如何处理网络错误或异常?
在下载文件的过程中,可能会遇到网络错误或其他异常情况。为了提高代码的健壮性,可以使用try-except
块来捕获异常,并处理错误。例如:
try:
response = requests.get(url)
response.raise_for_status() # 检查请求是否成功
with open('file.pdf', 'wb') as file:
file.write(response.content)
except requests.exceptions.RequestException as e:
print(f"下载文件时发生错误: {e}")
这样可以确保即使在遇到问题时,程序也不会崩溃。
如何使用Python下载大文件而不占用过多内存?
当下载大文件时,直接将整个文件内容载入内存可能会导致内存不足的情况。为了避免这种情况,可以逐块下载文件并写入磁盘。以下是一个示例:
with requests.get(url, stream=True) as response:
response.raise_for_status()
with open('large_file.zip', 'wb') as file:
for chunk in response.iter_content(chunk_size=8192):
file.write(chunk)
通过设置stream=True
,可以逐块读取数据,从而有效地管理内存使用。
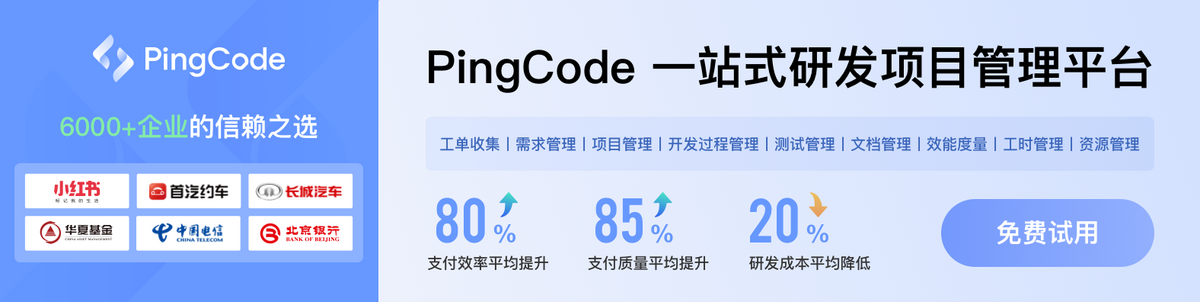