Python元祖中的值可以通过多种方式串起来,包括使用字符串连接、列表推导式和内置的字符串方法等。下面将详细介绍几种常见的方法:使用字符串拼接、使用join方法、使用map函数等。 其中,使用join
方法是最常见和推荐的方法,因为它不仅语法简洁,而且效率较高。
使用字符串拼接
这种方法适用于小型元组或对性能要求不高的场景。你可以通过循环遍历元组中的每一个元素,然后将它们逐个拼接成一个字符串。
# 示例元组
tuple_example = ('Python', 'is', 'fun')
字符串拼接
result = ''
for item in tuple_example:
result += item + ' '
result = result.strip()
print(result) # 输出: 'Python is fun'
使用 join 方法
join
方法是连接字符串最常用和最有效的方法。它将元组中的所有元素连接成一个字符串,中间用指定的分隔符分隔。
# 示例元组
tuple_example = ('Python', 'is', 'fun')
使用 join 方法
result = ' '.join(tuple_example)
print(result) # 输出: 'Python is fun'
使用 map 函数
如果元组中的元素不是字符串类型,可以使用map
函数将所有元素转换成字符串,然后再使用join
方法进行连接。
# 示例元组
tuple_example = (1, 2, 3)
使用 map 函数和 join 方法
result = ' - '.join(map(str, tuple_example))
print(result) # 输出: '1 - 2 - 3'
使用列表推导式
列表推导式是一种简洁的语法,适用于将元组中的值转换为字符串,然后再使用join
方法进行连接。
# 示例元组
tuple_example = ('Python', 'is', 'fun')
使用列表推导式和 join 方法
result = ' '.join([str(item) for item in tuple_example])
print(result) # 输出: 'Python is fun'
使用 functools.reduce
对于一些需要更复杂处理的情况,可以使用functools.reduce
来实现字符串连接。尽管这种方法不如join
方法常用,但在某些特定情况下可能会有用。
from functools import reduce
示例元组
tuple_example = ('Python', 'is', 'fun')
使用 reduce 函数
result = reduce(lambda x, y: x + ' ' + y, tuple_example)
print(result) # 输出: 'Python is fun'
性能对比
在实际应用中,性能也是需要考虑的一个因素。下面提供一个简单的性能对比,以帮助你选择最合适的方法。
import timeit
示例元组
tuple_example = ('Python', 'is', 'fun')
定义各个方法
def using_join():
return ' '.join(tuple_example)
def using_map():
return ' '.join(map(str, tuple_example))
def using_loop():
result = ''
for item in tuple_example:
result += item + ' '
return result.strip()
def using_reduce():
from functools import reduce
return reduce(lambda x, y: x + ' ' + y, tuple_example)
测试各个方法的性能
print('Join:', timeit.timeit(using_join, number=1000000))
print('Map:', timeit.timeit(using_map, number=1000000))
print('Loop:', timeit.timeit(using_loop, number=1000000))
print('Reduce:', timeit.timeit(using_reduce, number=1000000))
总结
在Python中,将元组中的值串起来有多种方法。最推荐的方法是使用join
方法,因为它不仅语法简洁,而且性能优异。对于需要将非字符串类型的元素转换为字符串的情况,可以结合map
函数使用join
方法。对于特殊需求,还可以使用functools.reduce
或者列表推导式等方法。总之,根据具体的需求和场景选择合适的方法,才能实现最佳的代码效果。
相关问答FAQs:
如何将Python元组中的值连接成一个字符串?
在Python中,可以使用join()
方法将元组中的值连接成一个字符串。首先,需要确保元组中的所有元素都是字符串类型。如果元组中包含其他数据类型,可以使用map()
函数将它们转换为字符串。以下是一个示例代码:
my_tuple = ('Hello', 'world', 'from', 'Python')
result = ' '.join(my_tuple)
print(result) # 输出: Hello world from Python
在元组中包含非字符串元素时如何处理?
当元组包含非字符串元素时,可以使用map()
函数将所有元素转换为字符串。例如,假设元组包含整数和字符串,可以这样处理:
my_tuple = (1, 'apple', 3, 'banana')
result = ' '.join(map(str, my_tuple))
print(result) # 输出: 1 apple 3 banana
通过这种方式,可以确保所有元素都能够成功连接为一个字符串。
是否可以使用其他分隔符连接元组中的值?
当然可以!join()
方法允许使用任何字符串作为分隔符。例如,如果希望用逗号连接元组中的元素,只需将逗号字符串传递给join()
方法:
my_tuple = ('apple', 'banana', 'cherry')
result = ', '.join(my_tuple)
print(result) # 输出: apple, banana, cherry
这样就可以轻松更改分隔符,以满足不同的格式需求。
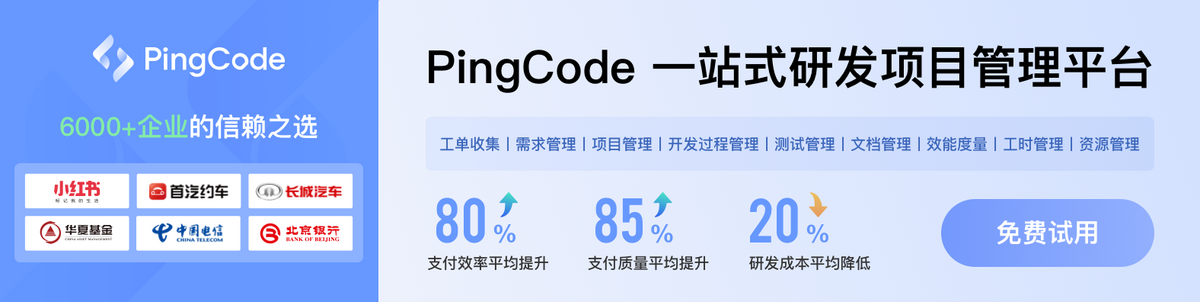