Python调用DLL库中的函数的方法有多种,常见的方法包括使用ctypes
、cffi
、swig
等工具。其中,最常用和最简单的方法是使用ctypes
库。以下将详细介绍如何使用ctypes
库来调用DLL中的函数。
1、准备工作
在开始调用DLL中的函数之前,需要确保以下几点:
- 安装了Python环境。
- 有一个需要调用的DLL文件。
- 知道DLL中函数的名称、参数类型和返回类型。
2、使用ctypes库
ctypes
是Python的一个外部函数库,它提供了与C语言兼容的数据类型,并允许调用动态链接库中的函数。下面是使用ctypes
库调用DLL中函数的步骤:
加载DLL
首先,需要加载DLL文件。可以使用ctypes.windll
或ctypes.cdll
来加载DLL文件,具体取决于DLL的调用约定。
import ctypes
加载DLL文件
my_dll = ctypes.CDLL('path/to/your/dllfile.dll')
定义函数原型
加载DLL文件后,需要定义函数的原型,包括函数名、参数类型和返回类型。可以使用argtypes
和restype
属性来定义函数的参数类型和返回类型。
# 定义函数原型
my_dll.my_function.argtypes = [ctypes.c_int, ctypes.c_double]
my_dll.my_function.restype = ctypes.c_double
调用函数
定义好函数原型后,就可以调用DLL中的函数了。
# 调用函数
result = my_dll.my_function(10, 20.5)
print(result)
3、详细介绍
加载DLL文件
在使用ctypes
库加载DLL文件时,可以使用ctypes.CDLL
、ctypes.windll
或ctypes.cdll
。这三者的区别在于调用约定不同。
ctypes.CDLL
:适用于标准C调用约定的DLL。ctypes.windll
:适用于Windows API调用约定的DLL。ctypes.cdll
:与ctypes.CDLL
类似,主要用于兼容性。
import ctypes
加载DLL文件
my_dll = ctypes.CDLL('path/to/your/dllfile.dll')
定义函数原型
在调用DLL中的函数之前,需要定义函数的原型。可以使用argtypes
和restype
属性来定义函数的参数类型和返回类型。
argtypes
:一个包含参数类型的列表。restype
:函数的返回类型。
# 定义函数原型
my_dll.my_function.argtypes = [ctypes.c_int, ctypes.c_double]
my_dll.my_function.restype = ctypes.c_double
调用函数
定义好函数原型后,就可以调用DLL中的函数了。调用函数时,传递的参数类型必须与定义的参数类型一致。
# 调用函数
result = my_dll.my_function(10, 20.5)
print(result)
4、处理复杂数据类型
有时,DLL函数的参数或返回类型可能是复杂数据类型,如结构体、数组、指针等。ctypes
库提供了相应的数据类型来处理这些复杂数据类型。
处理结构体
可以使用ctypes.Structure
来定义结构体。
class MyStruct(ctypes.Structure):
_fields_ = [('field1', ctypes.c_int),
('field2', ctypes.c_double)]
定义函数原型
my_dll.my_function.argtypes = [ctypes.POINTER(MyStruct)]
my_dll.my_function.restype = ctypes.c_int
创建结构体实例
my_struct = MyStruct()
my_struct.field1 = 10
my_struct.field2 = 20.5
调用函数
result = my_dll.my_function(ctypes.byref(my_struct))
print(result)
处理数组
可以使用ctypes
提供的数组类型来处理数组。
# 定义数组类型
ArrayType = ctypes.c_int * 5
定义函数原型
my_dll.my_function.argtypes = [ArrayType]
my_dll.my_function.restype = ctypes.c_int
创建数组实例
my_array = ArrayType(1, 2, 3, 4, 5)
调用函数
result = my_dll.my_function(my_array)
print(result)
处理指针
可以使用ctypes.POINTER
来处理指针。
# 定义函数原型
my_dll.my_function.argtypes = [ctypes.POINTER(ctypes.c_int)]
my_dll.my_function.restype = ctypes.c_int
创建指针实例
my_int = ctypes.c_int(10)
my_int_ptr = ctypes.pointer(my_int)
调用函数
result = my_dll.my_function(my_int_ptr)
print(result)
5、处理返回值
在调用DLL中的函数时,需要处理返回值。返回值的类型必须与定义的返回类型一致。
返回基本数据类型
如果函数返回基本数据类型,可以直接使用。
# 定义函数原型
my_dll.my_function.restype = ctypes.c_int
调用函数
result = my_dll.my_function()
print(result)
返回结构体
如果函数返回结构体,可以使用ctypes.Structure
来定义返回类型。
class MyStruct(ctypes.Structure):
_fields_ = [('field1', ctypes.c_int),
('field2', ctypes.c_double)]
定义函数原型
my_dll.my_function.restype = MyStruct
调用函数
result = my_dll.my_function()
print(result.field1, result.field2)
返回指针
如果函数返回指针,可以使用ctypes.POINTER
来定义返回类型。
# 定义函数原型
my_dll.my_function.restype = ctypes.POINTER(ctypes.c_int)
调用函数
result = my_dll.my_function()
print(result.contents.value)
6、错误处理
在调用DLL中的函数时,可能会遇到错误。可以使用ctypes
提供的错误处理机制来捕获和处理错误。
捕获异常
可以使用ctypes
的get_last_error
和set_last_error
函数来捕获和处理异常。
import ctypes
try:
# 调用函数
result = my_dll.my_function()
except Exception as e:
error_code = ctypes.get_last_error()
print(f"Error code: {error_code}, Error message: {str(e)}")
设置错误处理函数
可以使用ctypes
的errcheck
属性来设置错误处理函数。
def error_handler(result, func, args):
if result == 0:
raise Exception("Function call failed")
return result
定义函数原型
my_dll.my_function.restype = ctypes.c_int
my_dll.my_function.errcheck = error_handler
try:
# 调用函数
result = my_dll.my_function()
except Exception as e:
print(f"Error: {str(e)}")
7、小结
通过以上步骤,可以使用Python的ctypes
库来调用DLL中的函数。ctypes
库提供了丰富的数据类型和错误处理机制,可以方便地处理各种复杂数据类型和错误情况。在实际使用中,需要根据具体的DLL文件和函数定义,灵活使用ctypes
库提供的功能。
总结一下,使用ctypes
库调用DLL中的函数的步骤包括:加载DLL文件、定义函数原型、调用函数、处理复杂数据类型和返回值、错误处理等。通过这些步骤,可以方便地使用Python调用DLL中的各种函数,实现与其他编程语言的互操作。
相关问答FAQs:
1. 在Python中,如何加载一个DLL文件?
要在Python中加载DLL文件,可以使用ctypes
或cffi
库。以ctypes
为例,首先需要导入ctypes
模块,然后使用ctypes.CDLL()
函数加载DLL文件。示例代码如下:
import ctypes
my_dll = ctypes.CDLL('path_to_your_dll_file.dll')
请确保DLL文件的路径正确,并且该文件可以被访问。
2. 如何在Python中调用DLL中的特定函数?
加载DLL之后,可以通过属性访问DLL中的函数。为了调用特定函数,需确保正确设置参数和返回值的类型。示例如下:
my_function = my_dll.function_name
my_function.argtypes = [ctypes.c_int, ctypes.c_double] # 设置参数类型
my_function.restype = ctypes.c_double # 设置返回值类型
result = my_function(5, 3.14) # 调用函数
确保根据DLL中定义的函数签名设置适当的参数类型和返回类型。
3. 如何处理DLL函数中出现的错误或异常?
在调用DLL函数时,可能会遇到错误或异常情况。可以通过使用Python的异常处理机制(try
和except
)来捕捉这些错误。例如:
try:
result = my_function(5, 3.14)
except Exception as e:
print(f"Error occurred: {e}")
确保在调用DLL函数时,捕捉并处理可能出现的任何异常,以便于调试和错误追踪。
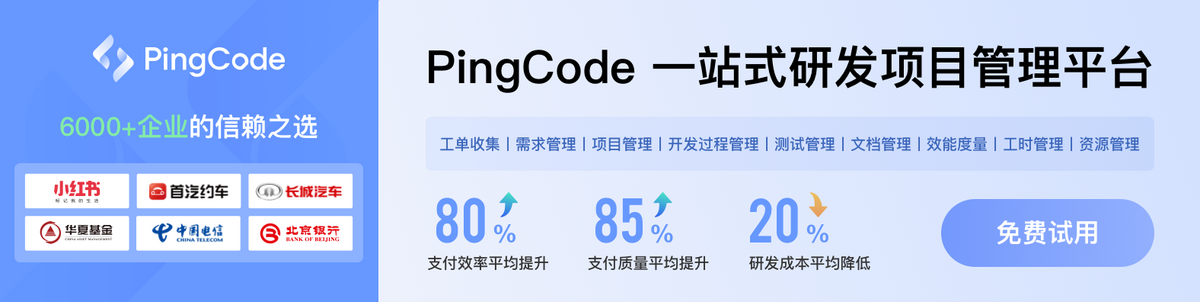