Python读取文件某一行的方法有多种,可以使用读取整个文件后按行分割、使用迭代器逐行读取、以及使用特定库等方式。其中,最常用的方式是读取整个文件后按行分割。这种方法简单易用,并且适用于大多数场景。下面我们将详细介绍几种常用的方法,并提供代码示例和注意事项。
一、使用readlines()
方法
使用readlines()
方法是最常见的方法之一。该方法会将文件的所有行读取到一个列表中,然后我们可以通过索引来访问特定的行。
def read_specific_line(file_path, line_number):
with open(file_path, 'r') as file:
lines = file.readlines()
if line_number < 1 or line_number > len(lines):
raise ValueError("Line number is out of range")
return lines[line_number - 1]
file_path = 'example.txt'
line_number = 3
print(read_specific_line(file_path, line_number))
详细描述:
在上面的代码中,readlines()
方法会将文件的所有行读取到一个列表中。然后,通过检查line_number
是否在有效范围内(即大于等于1并且小于等于文件的总行数),可以安全地访问特定的行。如果line_number
超出了范围,则会抛出一个ValueError
。
二、使用迭代器逐行读取
对于大文件,使用readlines()
方法可能会占用大量内存。此时,可以使用迭代器逐行读取文件,并在找到目标行时停止读取。
def read_specific_line(file_path, line_number):
with open(file_path, 'r') as file:
for current_line_number, line in enumerate(file, start=1):
if current_line_number == line_number:
return line
raise ValueError("Line number is out of range")
file_path = 'example.txt'
line_number = 3
print(read_specific_line(file_path, line_number))
详细描述:
在这个方法中,使用enumerate()
函数对文件对象进行迭代,并跟踪当前的行号。当找到目标行时,返回该行内容。如果迭代结束后仍未找到目标行,则抛出一个ValueError
。
三、使用linecache
模块
linecache
模块提供了一种便捷的方法来随机访问文本文件中的特定行。它会将文件内容缓存起来,以便后续访问更快。
import linecache
def read_specific_line(file_path, line_number):
line = linecache.getline(file_path, line_number)
if not line:
raise ValueError("Line number is out of range")
return line
file_path = 'example.txt'
line_number = 3
print(read_specific_line(file_path, line_number))
详细描述:
在这个方法中,linecache.getline()
函数可以直接获取文件中的特定行。如果行号超出了范围,该函数会返回一个空字符串,因此需要进行检查,并在必要时抛出ValueError
。
四、使用pandas
库
对于结构化文件(如CSV文件),使用pandas
库可以更方便地读取特定行的数据。
import pandas as pd
def read_specific_line(file_path, line_number):
df = pd.read_csv(file_path)
if line_number < 1 or line_number > len(df):
raise ValueError("Line number is out of range")
return df.iloc[line_number - 1]
file_path = 'example.csv'
line_number = 3
print(read_specific_line(file_path, line_number))
详细描述:
在这个方法中,pandas.read_csv()
函数会将CSV文件读取为一个DataFrame
对象。然后,通过检查line_number
是否在有效范围内,可以使用iloc
属性安全地访问特定的行。如果line_number
超出了范围,则会抛出一个ValueError
。
五、使用gzip
模块读取压缩文件
如果文件是gzip压缩文件,可以使用gzip
模块进行读取。
import gzip
def read_specific_line(file_path, line_number):
with gzip.open(file_path, 'rt') as file:
for current_line_number, line in enumerate(file, start=1):
if current_line_number == line_number:
return line
raise ValueError("Line number is out of range")
file_path = 'example.txt.gz'
line_number = 3
print(read_specific_line(file_path, line_number))
详细描述:
在这个方法中,gzip.open()
函数可以打开gzip压缩文件,并返回一个文件对象。通过使用迭代器逐行读取文件,可以在找到目标行时返回该行内容。如果迭代结束后仍未找到目标行,则抛出一个ValueError
。
注意事项
- 文件路径:确保提供的文件路径正确,并且文件存在。如果文件路径不正确或文件不存在,打开文件时会抛出
FileNotFoundError
。 - 行号范围:在读取特定行时,始终检查行号是否在有效范围内。如果行号超出范围,抛出适当的错误(如
ValueError
)。 - 内存占用:对于大文件,尽量使用逐行读取的方法,以避免占用过多内存。
- 文件类型:根据文件类型选择合适的读取方法。例如,对于CSV文件,可以使用
pandas
库;对于gzip压缩文件,可以使用gzip
模块。
通过上述几种方法,您可以根据具体需求选择合适的方式来读取文件的特定行。每种方法都有其优缺点,选择时需要综合考虑文件大小、内存占用、代码复杂度等因素。
相关问答FAQs:
如何使用Python读取文件中特定行的内容?
要读取文件中特定行的内容,可以使用Python的内置功能。打开文件后,可以使用readlines()
方法将文件的每一行读取为列表,然后根据索引选择所需的行。例如,如果想要读取第三行,可以使用lines[2]
。确保在读取之前检查文件是否存在以及行数是否足够。
在Python中,如何高效地读取大型文件的特定行?
对于大型文件,使用readlines()
可能会消耗大量内存。可以考虑逐行读取文件,使用enumerate()
来跟踪当前行号。这样可以在读取到目标行时立即处理,而无需加载整个文件到内存中,从而提高效率。
是否可以使用Python的库来简化文件行读取的操作?
是的,有一些第三方库可以简化读取文件的特定行。例如,使用pandas
库,可以通过pandas.read_csv()
读取CSV文件并轻松选择特定行。对于文本文件,也可以使用fileinput
模块,它允许您在处理文件时简化行的读取和操作。
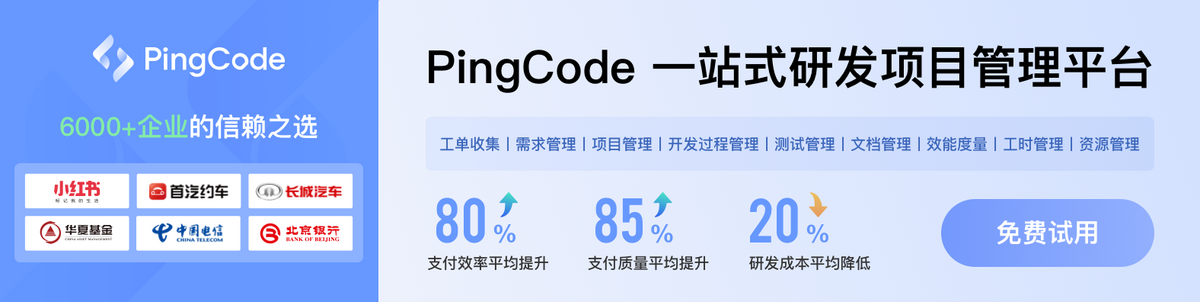