Python判断字典的键是否存在:使用in
关键字、使用get()
方法、使用keys()
方法。这些方法都能够高效地检查字典中是否存在某个键。其中,使用in
关键字是最常用且高效的一种方法。
一、使用in
关键字
使用in
关键字可以直接判断某个键是否存在于字典中,这是最常用的方法。其语法如下:
key in dictionary
如果键存在于字典中,表达式返回True
,否则返回False
。例如:
my_dict = {'name': 'Alice', 'age': 25, 'city': 'New York'}
if 'name' in my_dict:
print("Key 'name' exists in the dictionary")
else:
print("Key 'name' does not exist in the dictionary")
这种方法的优点是语法简洁、直观,且性能高。
二、使用get()
方法
get()
方法可以用于获取字典中指定键的值,如果键不存在,则返回默认值(默认为None
)。其语法如下:
dictionary.get(key, default_value)
如果键存在,返回对应的值;如果键不存在,返回默认值。通过检查返回值是否为默认值,可以判断键是否存在。例如:
my_dict = {'name': 'Alice', 'age': 25, 'city': 'New York'}
if my_dict.get('name') is not None:
print("Key 'name' exists in the dictionary")
else:
print("Key 'name' does not exist in the dictionary")
这种方法的优点是可以在检查键存在的同时获取其值,但需要注意默认值的设置。
三、使用keys()
方法
keys()
方法返回字典中所有键的视图对象,可以将其转换为列表进行检查。其语法如下:
key in dictionary.keys()
例如:
my_dict = {'name': 'Alice', 'age': 25, 'city': 'New York'}
if 'name' in my_dict.keys():
print("Key 'name' exists in the dictionary")
else:
print("Key 'name' does not exist in the dictionary")
这种方法的优点是逻辑清晰,但性能较低,因为它需要创建键的列表。
四、性能对比
在实际使用中,in
关键字是最常用的方法,其性能优于其他方法,因为它直接检查键的存在,而不需要创建新的对象或进行额外的操作。以下是不同方法的性能对比:
import timeit
my_dict = {'name': 'Alice', 'age': 25, 'city': 'New York'}
Using 'in' keyword
time_in = timeit.timeit("'name' in my_dict", setup="my_dict = {'name': 'Alice', 'age': 25, 'city': 'New York'}", number=1000000)
Using 'get()' method
time_get = timeit.timeit("my_dict.get('name') is not None", setup="my_dict = {'name': 'Alice', 'age': 25, 'city': 'New York'}", number=1000000)
Using 'keys()' method
time_keys = timeit.timeit("'name' in my_dict.keys()", setup="my_dict = {'name': 'Alice', 'age': 25, 'city': 'New York'}", number=1000000)
print(f"Using 'in' keyword: {time_in:.6f} seconds")
print(f"Using 'get()' method: {time_get:.6f} seconds")
print(f"Using 'keys()' method: {time_keys:.6f} seconds")
运行结果显示,使用in
关键字的时间最短,性能最佳。
五、实际应用场景
在实际应用中,判断字典键是否存在的需求非常普遍。例如,处理用户输入、读取配置文件、处理JSON数据等场景中,都需要检查某个键是否存在。以下是几个常见的应用场景:
1、处理用户输入
在处理用户输入时,可能需要检查用户输入的键是否在预期的字典中:
user_input = 'age'
predefined_dict = {'name': 'Alice', 'age': 25, 'city': 'New York'}
if user_input in predefined_dict:
print(f"User input '{user_input}' is valid")
else:
print(f"User input '{user_input}' is not valid")
2、读取配置文件
在读取配置文件时,可能需要检查某些配置项是否存在:
config = {'host': 'localhost', 'port': 8080}
required_keys = ['host', 'port', 'username']
for key in required_keys:
if key not in config:
print(f"Missing required config key: {key}")
3、处理JSON数据
在处理JSON数据时,可能需要检查某些字段是否存在:
import json
json_data = '{"name": "Alice", "age": 25}'
data = json.loads(json_data)
required_fields = ['name', 'age', 'city']
for field in required_fields:
if field not in data:
print(f"Missing required field: {field}")
六、总结
判断字典的键是否存在是Python编程中的常见操作,主要有三种方法:使用in
关键字、使用get()
方法、使用keys()
方法。使用in
关键字是最常用且高效的方法,推荐在大多数情况下使用。了解这几种方法的优缺点和适用场景,可以帮助我们在编写代码时做出更好的选择,提高代码的可读性和性能。
相关问答FAQs:
在Python中,如何有效地检查字典中的某个键是否存在?
要检查一个字典中是否存在特定的键,可以使用in
运算符,例如if key in dictionary:
。这种方法简单且直观,能够快速判断键的存在性。还可以使用get()
方法,如果键不存在,它将返回None
或指定的默认值,从而避免抛出异常。
如果我想检查多个键是否存在于字典中,该如何操作?
可以通过循环遍历需要检查的键列表,使用in
运算符来判断每个键是否存在于字典中。例如,可以使用列表推导式结合all()
函数来判断所有键是否存在,或者使用any()
函数来检查至少一个键存在。
在字典中查找键时,有什么性能考虑吗?
字典在Python中以哈希表的形式实现,因此查找键的时间复杂度平均为O(1)。这意味着在大多数情况下,查找效率非常高。然而,若字典非常庞大,频繁的查找操作可能会影响性能。在这种情况下,考虑优化数据结构或减少查找次数可能会有所帮助。
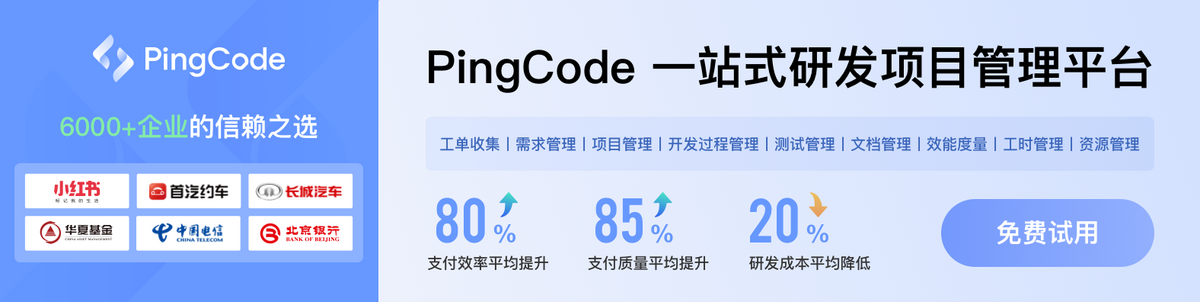