Python 生成横向柱状图的方法包括:使用Matplotlib、Seaborn、Plotly等库进行绘图。Matplotlib 是最常用的库,因为它功能强大且易于使用。下面将详细讲解如何使用 Matplotlib 生成横向柱状图。
一、使用 Matplotlib 生成横向柱状图
Matplotlib 是 Python 最常用的绘图库之一,用于创建各种图表。生成横向柱状图的主要函数是 barh()
。下面是详细步骤:
1. 安装 Matplotlib
首先,确保已安装 Matplotlib。如果未安装,可以使用 pip 命令进行安装:
pip install matplotlib
2. 导入 Matplotlib
在 Python 脚本或 Jupyter Notebook 中导入 Matplotlib 库。
import matplotlib.pyplot as plt
3. 准备数据
准备好要绘制的数据。假设我们有一组数据表示不同类别的值:
categories = ['A', 'B', 'C', 'D', 'E']
values = [3, 7, 2, 5, 6]
4. 绘制横向柱状图
使用 barh()
函数绘制横向柱状图。
plt.barh(categories, values)
plt.xlabel('Values')
plt.ylabel('Categories')
plt.title('Horizontal Bar Chart Example')
plt.show()
在上面的代码中,barh()
函数生成一个横向柱状图,xlabel()
和 ylabel()
用于设置轴标签,title()
用于设置图表标题,show()
用于显示图表。
5. 自定义图表
你可以通过多种方式自定义图表,例如更改颜色、添加网格线、显示数值标签等。
更改颜色
通过 color
参数更改柱子的颜色。
plt.barh(categories, values, color='skyblue')
添加网格线
使用 grid()
函数添加网格线。
plt.barh(categories, values, color='skyblue')
plt.grid(True)
显示数值标签
显示每个柱子旁边的数值标签。
plt.barh(categories, values, color='skyblue')
for index, value in enumerate(values):
plt.text(value, index, str(value))
二、使用 Seaborn 生成横向柱状图
Seaborn 是基于 Matplotlib 之上的高级绘图库,提供了更美观和简洁的绘图 API。
1. 安装 Seaborn
首先,确保已安装 Seaborn。如果未安装,可以使用 pip 命令进行安装:
pip install seaborn
2. 导入 Seaborn
在 Python 脚本或 Jupyter Notebook 中导入 Seaborn 库。
import seaborn as sns
import matplotlib.pyplot as plt
3. 准备数据
使用与 Matplotlib 相同的数据。
categories = ['A', 'B', 'C', 'D', 'E']
values = [3, 7, 2, 5, 6]
4. 绘制横向柱状图
使用 barplot()
函数,并将 orient
参数设置为 'h'
。
sns.barplot(x=values, y=categories, orient='h')
plt.xlabel('Values')
plt.ylabel('Categories')
plt.title('Horizontal Bar Chart Example using Seaborn')
plt.show()
5. 自定义图表
你可以通过多种方式自定义 Seaborn 图表,例如更改配色方案、添加网格线等。
更改配色方案
通过 palette
参数更改配色方案。
sns.barplot(x=values, y=categories, orient='h', palette='viridis')
添加网格线
使用 grid()
函数添加网格线。
sns.barplot(x=values, y=categories, orient='h', palette='viridis')
plt.grid(True)
三、使用 Plotly 生成横向柱状图
Plotly 是一个用于创建交互式图表的库,适用于 Web 应用程序。
1. 安装 Plotly
首先,确保已安装 Plotly。如果未安装,可以使用 pip 命令进行安装:
pip install plotly
2. 导入 Plotly
在 Python 脚本或 Jupyter Notebook 中导入 Plotly 库。
import plotly.graph_objects as go
3. 准备数据
使用与 Matplotlib 相同的数据。
categories = ['A', 'B', 'C', 'D', 'E']
values = [3, 7, 2, 5, 6]
4. 绘制横向柱状图
使用 Bar
函数创建一个横向柱状图。
fig = go.Figure(go.Bar(
x=values,
y=categories,
orientation='h'
))
fig.update_layout(
title='Horizontal Bar Chart Example using Plotly',
xaxis_title='Values',
yaxis_title='Categories'
)
fig.show()
5. 自定义图表
你可以通过多种方式自定义 Plotly 图表,例如更改颜色、添加网格线、显示数值标签等。
更改颜色
通过 marker
参数更改柱子的颜色。
fig = go.Figure(go.Bar(
x=values,
y=categories,
orientation='h',
marker=dict(color='skyblue')
))
添加网格线
使用 update_xaxes
和 update_yaxes
函数添加网格线。
fig = go.Figure(go.Bar(
x=values,
y=categories,
orientation='h',
marker=dict(color='skyblue')
))
fig.update_layout(
title='Horizontal Bar Chart Example using Plotly',
xaxis_title='Values',
yaxis_title='Categories'
)
fig.update_xaxes(showgrid=True)
fig.update_yaxes(showgrid=True)
fig.show()
四、总结
在本文中,我们详细介绍了如何使用 Matplotlib、Seaborn 和 Plotly 生成横向柱状图。Matplotlib 是最常用的库,因为它功能强大且易于使用;Seaborn 提供了更美观和简洁的绘图 API;Plotly 适用于创建交互式图表。你可以根据具体需求选择合适的库,并通过多种方式自定义图表,例如更改颜色、添加网格线、显示数值标签等。希望本文能帮助你更好地理解和使用 Python 生成横向柱状图。
相关问答FAQs:
如何在Python中选择合适的库来生成横向柱状图?
在Python中,常用的库有Matplotlib、Seaborn和Pandas等。Matplotlib是最基础的库,适合初学者。Seaborn建立在Matplotlib之上,提供更美观的默认样式,适合需要更复杂可视化的用户。Pandas则适合数据处理和简单可视化。如果你希望快速生成横向柱状图,可以选择Matplotlib或Seaborn。
生成横向柱状图时,如何设置轴标签和标题?
在使用Matplotlib时,可以使用plt.xlabel()
和plt.ylabel()
来设置横纵轴的标签,而plt.title()
用于添加图表标题。确保标签和标题简洁明了,以便观众快速理解图表所传达的信息。
如何调整横向柱状图的颜色和样式以提升可读性?
在Matplotlib中,可以通过color
参数自定义柱子的颜色。建议使用对比明显的颜色组合,避免使用过于鲜艳或相近的颜色,以提高可读性。此外,可以使用edgecolor
参数为柱子添加边框,从而使图表更加清晰。
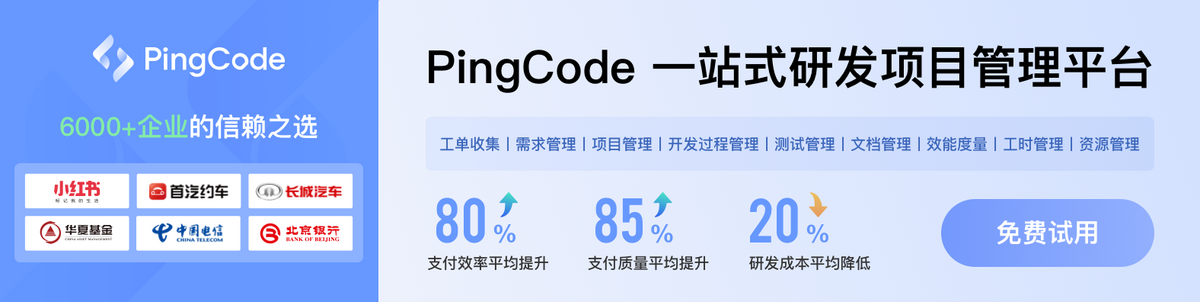