一、Python如何输入列表输出得分
Python可以通过input函数、文件读取、API调用等方式输入列表,通过循环、条件判断等逻辑处理计算得分、通过内置函数或库函数实现输出。 其中,常见的方式是通过input()
函数获取用户输入的列表,然后利用循环和条件语句对列表中的元素进行处理,计算出得分并输出结果。详细描述其中一种方法:使用input()
函数获取用户输入,将其转换为列表并计算得分。
例子:用户输入一组考试成绩,程序将这些成绩存储在一个列表中,然后计算出这些成绩的总分或平均分,并将结果输出。以下是实现这一功能的Python代码示例:
# 获取用户输入的成绩列表
scores_input = input("请输入成绩列表(用逗号分隔):")
将输入的字符串转换为列表
scores_list = [int(score) for score in scores_input.split(',')]
计算总分
total_score = sum(scores_list)
计算平均分
average_score = total_score / len(scores_list)
print(f"总分:{total_score}")
print(f"平均分:{average_score:.2f}")
在上面的示例中,用户输入的成绩通过input()
函数获取,并使用split()
方法将其分割成一个字符串列表。然后,通过列表推导式将每个字符串元素转换为整数,并存储在scores_list
中。接下来,使用sum()
函数计算总分,并计算平均分,最后输出结果。
二、从文件读取列表并计算得分
除了从用户输入获取列表,还可以从文件中读取列表。假设有一个包含成绩的文件,每行一个成绩。我们可以使用Python的文件处理功能读取文件内容,并计算得分。
# 打开文件
with open('scores.txt', 'r') as file:
# 读取文件内容并转换为列表
scores_list = [int(line.strip()) for line in file]
计算总分
total_score = sum(scores_list)
计算平均分
average_score = total_score / len(scores_list)
print(f"总分:{total_score}")
print(f"平均分:{average_score:.2f}")
在这个示例中,使用open()
函数打开文件,并通过列表推导式将每行内容转换为整数并存储在scores_list
中。接下来的步骤与前面的示例相同,计算总分和平均分并输出结果。
三、通过API获取列表并计算得分
有时候,列表数据可能需要通过API获取。我们可以使用Python的requests
库来发送HTTP请求并获取数据。
import requests
发送HTTP请求获取数据
response = requests.get('https://api.example.com/scores')
假设API返回的是JSON格式的数据
data = response.json()
提取成绩列表
scores_list = data['scores']
计算总分
total_score = sum(scores_list)
计算平均分
average_score = total_score / len(scores_list)
print(f"总分:{total_score}")
print(f"平均分:{average_score:.2f}")
在这个示例中,使用requests.get()
函数发送HTTP GET请求,并使用json()
方法将响应内容解析为Python字典。然后,从字典中提取成绩列表,计算总分和平均分并输出结果。
四、使用函数封装输入列表和计算得分
为了提高代码的复用性和可维护性,可以将输入列表和计算得分的逻辑封装到函数中。
def get_scores_from_input():
scores_input = input("请输入成绩列表(用逗号分隔):")
return [int(score) for score in scores_input.split(',')]
def get_scores_from_file(file_path):
with open(file_path, 'r') as file:
return [int(line.strip()) for line in file]
def get_scores_from_api(url):
import requests
response = requests.get(url)
data = response.json()
return data['scores']
def calculate_total_and_average(scores_list):
total_score = sum(scores_list)
average_score = total_score / len(scores_list)
return total_score, average_score
示例调用
scores_list = get_scores_from_input()
total_score, average_score = calculate_total_and_average(scores_list)
print(f"总分:{total_score}")
print(f"平均分:{average_score:.2f}")
在这个示例中,定义了四个函数:get_scores_from_input()
、get_scores_from_file()
、get_scores_from_api()
和 calculate_total_and_average()
。每个函数负责一个特定的任务,使得代码更加清晰和模块化。
五、处理不同类型的得分
在实际应用中,不同类型的得分可能需要不同的处理方法。例如,考试成绩、比赛成绩和评级分数可能有不同的计算规则。
def calculate_exam_scores(scores_list):
return sum(scores_list), sum(scores_list) / len(scores_list)
def calculate_competition_scores(scores_list):
return max(scores_list), min(scores_list)
def calculate_rating_scores(scores_list):
return sum(scores_list) / len(scores_list)
示例调用
exam_scores = [80, 85, 90, 75, 88]
competition_scores = [9.5, 8.7, 9.0, 9.8]
rating_scores = [4.5, 4.0, 4.2, 4.8, 4.7]
total_exam, average_exam = calculate_exam_scores(exam_scores)
max_competition, min_competition = calculate_competition_scores(competition_scores)
average_rating = calculate_rating_scores(rating_scores)
print(f"考试总分:{total_exam},平均分:{average_exam:.2f}")
print(f"比赛最高分:{max_competition},最低分:{min_competition}")
print(f"评级平均分:{average_rating:.2f}")
在这个示例中,根据不同类型的得分定义了不同的计算函数,并分别调用这些函数处理对应的得分列表。这样可以根据具体需求灵活选择不同的计算方法。
六、处理异常情况
在输入列表和计算得分的过程中,可能会遇到一些异常情况,比如输入格式错误、文件不存在、API请求失败等。我们可以通过异常处理机制来处理这些情况。
def get_scores_from_input():
try:
scores_input = input("请输入成绩列表(用逗号分隔):")
return [int(score) for score in scores_input.split(',')]
except ValueError:
print("输入格式错误,请输入整数列表。")
return []
def get_scores_from_file(file_path):
try:
with open(file_path, 'r') as file:
return [int(line.strip()) for line in file]
except FileNotFoundError:
print(f"文件 {file_path} 不存在。")
return []
except ValueError:
print("文件内容格式错误,请检查文件内容。")
return []
def get_scores_from_api(url):
import requests
try:
response = requests.get(url)
response.raise_for_status()
data = response.json()
return data['scores']
except requests.RequestException as e:
print(f"API请求失败:{e}")
return []
def calculate_total_and_average(scores_list):
if not scores_list:
return 0, 0.0
total_score = sum(scores_list)
average_score = total_score / len(scores_list)
return total_score, average_score
示例调用
scores_list = get_scores_from_input()
total_score, average_score = calculate_total_and_average(scores_list)
print(f"总分:{total_score}")
print(f"平均分:{average_score:.2f}")
在这个示例中,通过try-except
块处理可能出现的异常情况,并在异常发生时输出相应的错误信息。这可以提高程序的健壮性和用户体验。
七、综合示例:实现一个完整的得分计算程序
最后,我们可以将上述所有内容整合到一个完整的程序中,实现一个多功能的得分计算程序。
import requests
def get_scores_from_input():
try:
scores_input = input("请输入成绩列表(用逗号分隔):")
return [int(score) for score in scores_input.split(',')]
except ValueError:
print("输入格式错误,请输入整数列表。")
return []
def get_scores_from_file(file_path):
try:
with open(file_path, 'r') as file:
return [int(line.strip()) for line in file]
except FileNotFoundError:
print(f"文件 {file_path} 不存在。")
return []
except ValueError:
print("文件内容格式错误,请检查文件内容。")
return []
def get_scores_from_api(url):
try:
response = requests.get(url)
response.raise_for_status()
data = response.json()
return data['scores']
except requests.RequestException as e:
print(f"API请求失败:{e}")
return []
def calculate_total_and_average(scores_list):
if not scores_list:
return 0, 0.0
total_score = sum(scores_list)
average_score = total_score / len(scores_list)
return total_score, average_score
def main():
print("请选择输入方式:")
print("1. 用户输入")
print("2. 从文件读取")
print("3. 从API获取")
choice = input("请输入选项(1/2/3):")
if choice == '1':
scores_list = get_scores_from_input()
elif choice == '2':
file_path = input("请输入文件路径:")
scores_list = get_scores_from_file(file_path)
elif choice == '3':
api_url = input("请输入API URL:")
scores_list = get_scores_from_api(api_url)
else:
print("无效选项。")
return
total_score, average_score = calculate_total_and_average(scores_list)
print(f"总分:{total_score}")
print(f"平均分:{average_score:.2f}")
if __name__ == "__main__":
main()
在这个综合示例中,实现了一个多功能的得分计算程序,用户可以选择从输入、文件或API获取成绩列表,然后计算总分和平均分并输出结果。程序通过异常处理机制处理可能出现的错误,确保程序的健壮性和可靠性。
相关问答FAQs:
如何在Python中输入一个列表并输出每个元素的得分?
在Python中,您可以通过定义一个列表并使用循环来遍历每个元素,结合一个评分函数来计算得分。例如,您可以使用字典来映射每个元素到其对应的得分,然后输出结果。
在Python中如何为列表中的元素定义得分规则?
得分规则可以通过创建一个字典来实现,其中键是列表元素,值是对应的得分。您可以根据需求调整得分的逻辑,例如基于元素的类型、数量或其他属性进行评分。
能否在Python中实现动态输入列表并自动计算得分?
当然可以,您可以使用input()
函数让用户输入元素,接着将输入转换为列表。然后,使用预设的评分规则来计算每个元素的得分,并输出总得分或详细得分。利用map()
函数或列表推导式可以简化得分计算的过程。
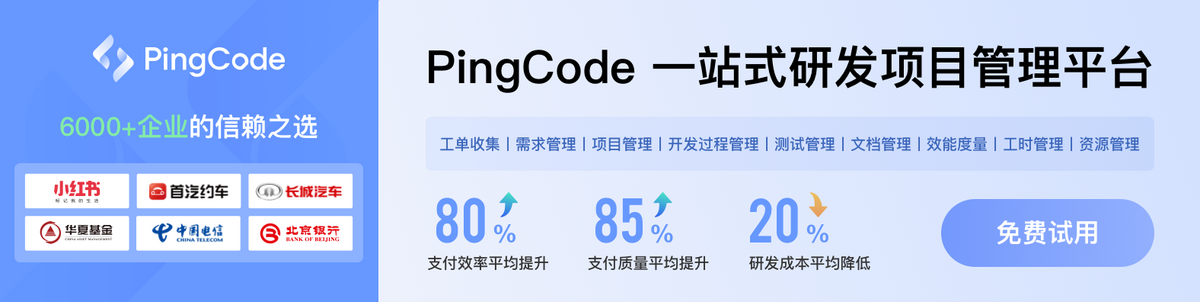