Python 读文本如何读取某一行
Python读取文本文件中特定的某一行可以通过多种方式实现,如使用文件对象的readlines方法、逐行遍历文件、以及使用第三方库等。 其中一种常见的方法是使用文件对象的readlines方法,将文件中的所有行读取到一个列表中,并通过索引访问特定的行。另一种方法是使用逐行遍历文件,通过计数器找到特定行。这两种方法各有优劣,具体选择可以根据实际需求来定。
其中一种方法是使用文件对象的readlines方法,将文件中的所有行读取到一个列表中,并通过索引访问特定的行。这种方法的优点是简单直接,适合文件行数较少的情况。下面是该方法的详细描述。
一、使用 readlines
方法读取特定行
readlines
方法会将文件的所有行读取到一个列表中,我们可以通过索引访问特定的行。以下是一个示例代码:
def read_specific_line(file_path, line_number):
try:
with open(file_path, 'r') as file:
lines = file.readlines()
if line_number <= len(lines):
return lines[line_number - 1].strip()
else:
return f"Error: The file has only {len(lines)} lines."
except Exception as e:
return f"Error: {str(e)}"
file_path = 'example.txt'
line_number = 3
print(read_specific_line(file_path, line_number))
这个函数 read_specific_line
接受文件路径和行号作为参数,使用 readlines
方法读取文件的所有行,并返回特定行。如果行号超过文件的总行数,则返回错误信息。
二、逐行遍历文件读取特定行
在处理大文件时,使用 readlines
方法可能会占用大量内存。这时可以使用逐行遍历文件的方法,通过计数器找到特定行。以下是示例代码:
def read_specific_line(file_path, line_number):
try:
with open(file_path, 'r') as file:
for current_line_number, line in enumerate(file, start=1):
if current_line_number == line_number:
return line.strip()
return f"Error: The file has fewer than {line_number} lines."
except Exception as e:
return f"Error: {str(e)}"
file_path = 'example.txt'
line_number = 3
print(read_specific_line(file_path, line_number))
这个函数 read_specific_line
同样接受文件路径和行号作为参数,通过逐行遍历文件并计数,找到特定行并返回。如果行号超过文件的总行数,则返回错误信息。
三、使用 itertools
模块读取特定行
Python 的 itertools
模块提供了一个方便的方法来处理迭代器,可以用来读取文件的特定行。以下是示例代码:
import itertools
def read_specific_line(file_path, line_number):
try:
with open(file_path, 'r') as file:
line = next(itertools.islice(file, line_number - 1, line_number), None)
if line:
return line.strip()
else:
return f"Error: The file has fewer than {line_number} lines."
except Exception as e:
return f"Error: {str(e)}"
file_path = 'example.txt'
line_number = 3
print(read_specific_line(file_path, line_number))
这个函数 read_specific_line
使用 itertools.islice
方法从文件中切片,读取特定行并返回。如果行号超过文件的总行数,则返回错误信息。
四、使用 pandas
库读取特定行
如果文件是一个结构化的文本文件(如 CSV 文件),可以使用 pandas
库来读取特定行。以下是示例代码:
import pandas as pd
def read_specific_line(file_path, line_number):
try:
df = pd.read_csv(file_path, header=None)
if line_number <= len(df):
return df.iloc[line_number - 1].to_string(index=False)
else:
return f"Error: The file has only {len(df)} lines."
except Exception as e:
return f"Error: {str(e)}"
file_path = 'example.csv'
line_number = 3
print(read_specific_line(file_path, line_number))
这个函数 read_specific_line
使用 pandas
库读取 CSV 文件,并通过 iloc
方法访问特定行。如果行号超过文件的总行数,则返回错误信息。
五、处理特殊情况
在实际应用中,可能会遇到一些特殊情况,例如文件编码问题、文件不存在等。在编写读取特定行的函数时,应该考虑这些情况,并添加相应的错误处理机制。以下是一个更为完善的示例代码:
def read_specific_line(file_path, line_number):
try:
with open(file_path, 'r', encoding='utf-8') as file:
for current_line_number, line in enumerate(file, start=1):
if current_line_number == line_number:
return line.strip()
return f"Error: The file has fewer than {line_number} lines."
except FileNotFoundError:
return "Error: The file does not exist."
except UnicodeDecodeError:
return "Error: Unable to decode the file with 'utf-8' encoding."
except Exception as e:
return f"Error: {str(e)}"
file_path = 'example.txt'
line_number = 3
print(read_specific_line(file_path, line_number))
这个函数 read_specific_line
添加了文件不存在、文件编码错误等异常处理,更加健壮可靠。
总结
通过上述几种方法,可以灵活地读取文本文件中的特定行。具体选择哪种方法,可以根据文件的大小、文件格式、以及对性能的要求来决定。总的来说,使用 readlines
方法适合小文件,逐行遍历适合大文件,itertools
模块提供了简洁的切片方式,pandas
库适合处理结构化文本文件。 而在实际应用中,添加错误处理机制是保证程序健壮性的关键。
相关问答FAQs:
如何使用Python读取文本文件中的特定行?
要读取文本文件中的特定行,您可以使用Python的内置文件处理功能。打开文件后,可以使用readlines()
方法读取所有行并将其存储在列表中,然后通过索引访问所需的行。例如,如果您希望读取第二行,可以这样做:
with open('file.txt', 'r') as file:
lines = file.readlines()
second_line = lines[1] # 索引从0开始
这种方法简单且直观,但要注意文件的大小,如果文件很大,可能会影响性能。
是否可以直接读取文本文件的特定行而不加载整个文件?
是的,您可以通过逐行读取文件来直接获取特定行。这种方法更为高效,特别是对于大型文件。可以使用enumerate()
函数来遍历文件的每一行,并在达到所需行时立即返回结果。示例代码如下:
with open('file.txt', 'r') as file:
for index, line in enumerate(file):
if index == 1: # 读取第二行
second_line = line
break
这种方法可以节省内存,并提高性能,尤其是在处理大文件时。
如何处理文件中不存在的行?
在读取特定行时,如果该行不存在,您应该考虑异常处理来避免程序崩溃。可以使用try-except
结构来捕获索引错误,并提供用户友好的反馈。例如:
with open('file.txt', 'r') as file:
lines = file.readlines()
try:
specific_line = lines[10] # 假设我们想读取第11行
except IndexError:
specific_line = "指定的行不存在。"
这种方式确保程序在遇到问题时仍能正常运行并告知用户错误信息。
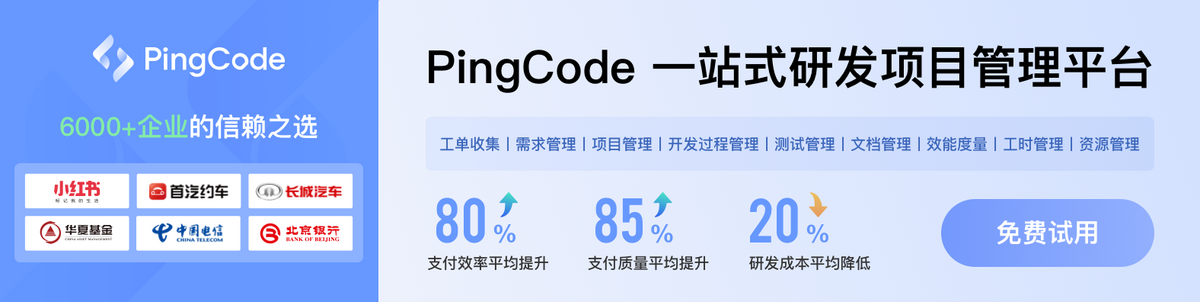