用Python计算两点距离的方法有多种,包括使用数学公式、内置库和第三方库。其中一种常用的方法是使用欧几里得距离公式来计算两点之间的直线距离。另一种方法是使用Python的内置库如math库,或者利用第三方库如NumPy。下面详细介绍如何使用这些方法计算两点之间的距离。
一、使用数学公式计算欧几里得距离
欧几里得距离是最常见的计算两点之间距离的方法。计算公式为:
[ \text{distance} = \sqrt{(x_2 – x_1)^2 + (y_2 – y_1)^2} ]
1.1、基础方法
首先,我们可以使用Python的基本数学运算来实现这一公式。以下是一个简单的例子:
import math
def calculate_distance(x1, y1, x2, y2):
distance = math.sqrt((x2 - x1)<strong>2 + (y2 - y1)</strong>2)
return distance
示例
x1, y1 = 0, 0
x2, y2 = 3, 4
print(calculate_distance(x1, y1, x2, y2)) # 输出:5.0
这个方法使用了math库中的sqrt函数来计算平方根,从而得到两点之间的距离。
1.2、扩展到三维空间
如果需要计算三维空间中的两点距离,可以扩展公式为:
[ \text{distance} = \sqrt{(x_2 – x_1)^2 + (y_2 – y_1)^2 + (z_2 – z_1)^2} ]
以下是一个三维距离计算的例子:
import math
def calculate_distance_3d(x1, y1, z1, x2, y2, z2):
distance = math.sqrt((x2 - x1)<strong>2 + (y2 - y1)</strong>2 + (z2 - z1)2)
return distance
示例
x1, y1, z1 = 0, 0, 0
x2, y2, z2 = 3, 4, 5
print(calculate_distance_3d(x1, y1, z1, x2, y2, z2)) # 输出:7.0710678118654755
二、使用内置库计算距离
Python的math库提供了丰富的数学函数,可以简化距离计算。
2.1、使用math.dist函数
从Python 3.8开始,math库引入了dist函数,可以直接用于计算两点之间的欧几里得距离。
import math
def calculate_distance(x1, y1, x2, y2):
distance = math.dist((x1, y1), (x2, y2))
return distance
示例
x1, y1 = 0, 0
x2, y2 = 3, 4
print(calculate_distance(x1, y1, x2, y2)) # 输出:5.0
这个方法比前面的手动计算更简洁和直观。
三、使用第三方库NumPy计算距离
NumPy是一个强大的科学计算库,提供了许多方便的函数来处理多维数组和矩阵运算。
3.1、使用NumPy的linalg.norm函数
NumPy的linalg模块提供了norm函数,可以用于计算向量的范数,包括欧几里得距离。
import numpy as np
def calculate_distance_numpy(x1, y1, x2, y2):
point1 = np.array([x1, y1])
point2 = np.array([x2, y2])
distance = np.linalg.norm(point1 - point2)
return distance
示例
x1, y1 = 0, 0
x2, y2 = 3, 4
print(calculate_distance_numpy(x1, y1, x2, y2)) # 输出:5.0
3.2、扩展到多维空间
NumPy的优势在于它可以轻松处理多维空间中的距离计算。
import numpy as np
def calculate_distance_multi_dim(point1, point2):
distance = np.linalg.norm(np.array(point1) - np.array(point2))
return distance
示例
point1 = [0, 0, 0]
point2 = [3, 4, 5]
print(calculate_distance_multi_dim(point1, point2)) # 输出:7.0710678118654755
四、其他距离计算方法
除了欧几里得距离,还有其他几种常见的距离计算方法,包括曼哈顿距离、切比雪夫距离和闵可夫斯基距离等。
4.1、曼哈顿距离
曼哈顿距离是指在一个网格状的路径上,两个点之间的距离。计算公式为:
[ \text{distance} = |x_2 – x_1| + |y_2 – y_1| ]
def calculate_manhattan_distance(x1, y1, x2, y2):
distance = abs(x2 - x1) + abs(y2 - y1)
return distance
示例
x1, y1 = 0, 0
x2, y2 = 3, 4
print(calculate_manhattan_distance(x1, y1, x2, y2)) # 输出:7
4.2、切比雪夫距离
切比雪夫距离是指在棋盘上的两个点之间的最大步数。计算公式为:
[ \text{distance} = \max(|x_2 – x_1|, |y_2 – y_1|) ]
def calculate_chebyshev_distance(x1, y1, x2, y2):
distance = max(abs(x2 - x1), abs(y2 - y1))
return distance
示例
x1, y1 = 0, 0
x2, y2 = 3, 4
print(calculate_chebyshev_distance(x1, y1, x2, y2)) # 输出:4
4.3、闵可夫斯基距离
闵可夫斯基距离是欧几里得距离和曼哈顿距离的泛化形式。计算公式为:
[ \text{distance} = \left( \sum_{i=1}^{n} |x_i – y_i|^p \right)^{1/p} ]
def calculate_minkowski_distance(x1, y1, x2, y2, p):
distance = (abs(x2 - x1)<strong>p + abs(y2 - y1)</strong>p)(1/p)
return distance
示例
x1, y1 = 0, 0
x2, y2 = 3, 4
print(calculate_minkowski_distance(x1, y1, x2, y2, 3)) # 输出:4.497941445275415
五、实际应用中的距离计算
在实际应用中,距离计算常用于数据分析、机器学习和地理信息系统等领域。
5.1、数据分析中的距离计算
在数据分析中,距离计算可以用于聚类分析、异常检测和最近邻搜索等。
from sklearn.metrics.pairwise import euclidean_distances
def calculate_euclidean_distances(data):
distances = euclidean_distances(data)
return distances
示例
data = [[0, 0], [3, 4], [6, 8]]
print(calculate_euclidean_distances(data))
输出:
[[ 0. 5. 10.]
[ 5. 0. 5.]
[10. 5. 0.]]
5.2、地理信息系统中的距离计算
在地理信息系统中,距离计算可以用于计算地球上两点之间的距离。
from geopy.distance import geodesic
def calculate_geodesic_distance(coord1, coord2):
distance = geodesic(coord1, coord2).kilometers
return distance
示例
coord1 = (52.2296756, 21.0122287)
coord2 = (41.8919300, 12.5113300)
print(calculate_geodesic_distance(coord1, coord2)) # 输出:1318.1387158165856
六、总结
计算两点之间的距离在许多领域都有广泛的应用,从基础的数学公式到高级的库函数,Python提供了多种方法来实现这一功能。使用数学公式、内置库和第三方库如NumPy和geopy都可以有效地计算距离。根据实际需求选择合适的方法,可以极大地提高工作效率和计算精度。
相关问答FAQs:
使用Python计算两点距离的方法是什么?
在Python中,可以使用欧几里得距离公式来计算两点之间的距离。假设有两个点A(x1, y1)和B(x2, y2),可以利用数学库中的函数来实现。具体公式为:距离 = √((x2 – x1)² + (y2 – y1)²)。通过Python的math库,可以轻松实现这一计算。
Python中有哪些库可以帮助计算两点之间的距离?
除了使用math库外,Python中的NumPy和SciPy库也提供了简便的方法来计算距离。NumPy可以通过数组的方式处理数据,而SciPy则提供了更为丰富的距离计算函数,例如曼哈顿距离、余弦相似度等。这些库可以处理多维数据,并且提供了高效的计算性能。
如何处理三维空间中两点的距离计算?
在三维空间中,计算两点A(x1, y1, z1)和B(x2, y2, z2)的距离,只需扩展欧几里得距离公式。公式变为:距离 = √((x2 – x1)² + (y2 – y1)² + (z2 – z1)²)。同样可以使用Python的math库或其他科学计算库来实现这一功能,确保可以适应不同维度的数据计算需求。
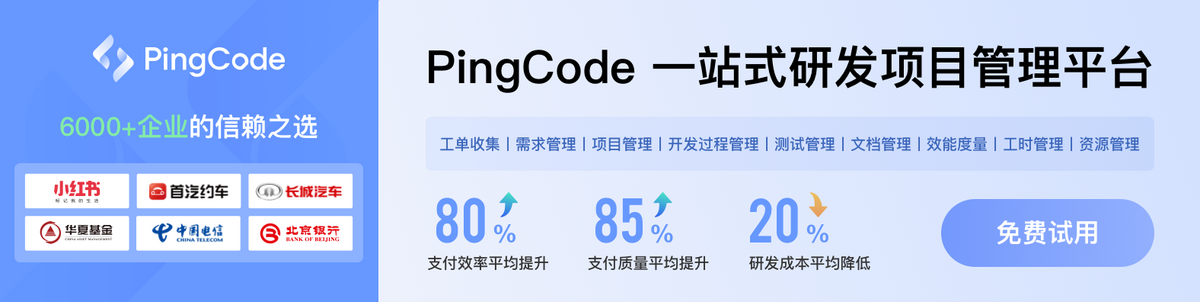