Python在txt文件中寻找关键词的方法有:读取文件内容、使用字符串方法查找、使用正则表达式等。其中,使用字符串方法查找最为简单和常用。
要在Python中找到txt文件中的关键词,首先需要读取文件内容,然后可以使用Python的字符串方法进行查找,也可以使用正则表达式进行更复杂的匹配。下面将详细介绍这两种方法,以及一些实际应用中的技巧和注意事项。
一、读取文件内容
在开始寻找关键词之前,首先需要读取txt文件的内容。Python提供了内置的open
函数来实现这一点。
def read_file(file_path):
with open(file_path, 'r', encoding='utf-8') as file:
content = file.read()
return content
上面的代码展示了如何读取文件的全部内容,并将其存储在一个字符串变量中。file_path
是txt文件的路径,encoding
参数可以根据实际情况进行调整。
二、使用字符串方法查找
读取文件内容后,可以使用Python字符串的内置方法来查找关键词。这些方法包括find
、index
、count
等。
1、find方法
find
方法用于查找子字符串在字符串中的位置,如果找不到则返回-1。
def find_keyword(content, keyword):
position = content.find(keyword)
return position
这个函数会返回关键词在内容中的位置,如果返回-1,说明关键词不存在。
2、index方法
index
方法与find
方法类似,但如果找不到子字符串会引发ValueError
异常。
def index_keyword(content, keyword):
try:
position = content.index(keyword)
return position
except ValueError:
return -1
3、count方法
count
方法用于统计子字符串在字符串中出现的次数。
def count_keyword(content, keyword):
return content.count(keyword)
三、使用正则表达式查找
对于更复杂的匹配需求,可以使用Python的re
模块来进行正则表达式查找。正则表达式在处理模式匹配时非常强大。
import re
def find_keyword_regex(content, pattern):
matches = re.finditer(pattern, content)
positions = [match.start() for match in matches]
return positions
上面的代码会返回所有匹配模式的起始位置列表,pattern
是正则表达式模式。
四、结合具体案例
为了更好地理解上述方法,下面结合具体案例进行演示。
1、案例一:查找单个关键词
假设有一个txt文件example.txt
,内容如下:
Python是一种广泛使用的高级编程语言。
Python的设计哲学强调代码的可读性。
Python支持多种编程范式,包括过程式、面向对象和函数式编程。
我们希望查找关键词“Python”在文件中的位置。
file_path = 'example.txt'
keyword = 'Python'
content = read_file(file_path)
position = find_keyword(content, keyword)
print(f"关键词'{keyword}'的位置: {position}")
2、案例二:统计关键词出现次数
count = count_keyword(content, keyword)
print(f"关键词'{keyword}'出现的次数: {count}")
3、案例三:使用正则表达式查找
假设我们希望查找所有以“编程”结尾的词语。
pattern = r'\b\w*编程\b'
positions = find_keyword_regex(content, pattern)
print(f"匹配模式'{pattern}'的位置: {positions}")
五、处理大文件
对于大文件,读取全部内容可能会占用大量内存,因此可以考虑按行处理。
def find_keyword_in_large_file(file_path, keyword):
positions = []
with open(file_path, 'r', encoding='utf-8') as file:
for line_number, line in enumerate(file):
position = line.find(keyword)
if position != -1:
positions.append((line_number, position))
return positions
这个函数会返回关键词在每一行中的位置。
六、总结
通过以上内容,我们详细介绍了如何在Python中查找txt文件中的关键词,包括读取文件内容、使用字符串方法查找、使用正则表达式查找等。这些方法在不同的场景下各有优劣,可以根据具体需求进行选择。在处理大文件时,需要特别注意内存的使用,可以选择按行处理的方式。
Python的强大之处在于其丰富的标准库和简洁的语法,使得文本处理变得非常高效和灵活。通过本文的介绍,希望能够帮助读者更好地理解和掌握在txt文件中寻找关键词的方法。
相关问答FAQs:
如何使用Python读取txt文件中的内容?
在Python中,可以使用内置的open()
函数来读取txt文件。通过read()
方法可以读取整个文件内容,或者使用readlines()
方法逐行读取。以下是一个简单的示例代码:
with open('yourfile.txt', 'r', encoding='utf-8') as file:
content = file.read()
确保在打开文件时指定正确的编码,以避免乱码问题。
Python中如何实现关键词搜索功能?
在Python中,可以通过使用字符串的in
运算符来检查一个关键词是否存在于文本中。比如,可以结合读取文件的内容,利用循环逐行检查关键词。示例代码如下:
keyword = "your_keyword"
with open('yourfile.txt', 'r', encoding='utf-8') as file:
for line in file:
if keyword in line:
print(line)
这种方法可以有效地找到包含特定关键词的行。
如何提高关键词搜索的效率?
在处理大文件时,可以考虑使用正则表达式来提高搜索效率。Python的re
模块提供了强大的字符串搜索能力。使用正则表达式可以实现更复杂的搜索需求,比如搜索多个关键词或模式匹配。以下是一个示例代码:
import re
pattern = r'your_keyword|another_keyword'
with open('yourfile.txt', 'r', encoding='utf-8') as file:
for line in file:
if re.search(pattern, line):
print(line)
这种方法适合需要处理复杂搜索条件的场景。
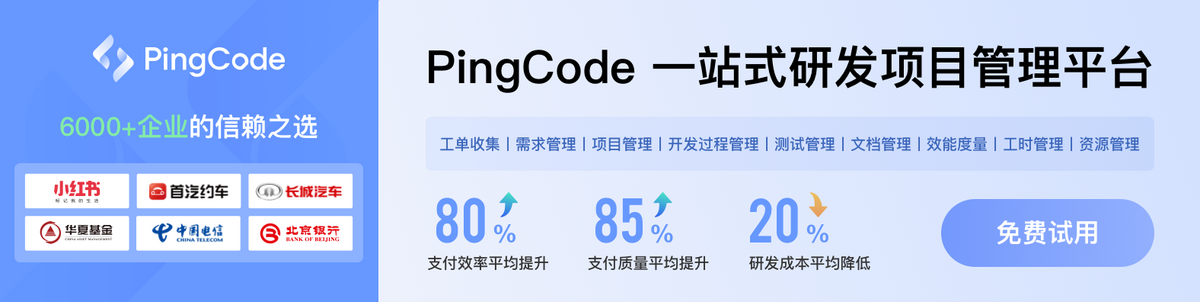