在Python中判断字符串长度的核心方法是使用内置函数len()
。此外,可以通过迭代字符串、使用正则表达式、以及其他内置模块的方法来实现。其中最常用和直接的方法是使用len()
函数。下面将详细介绍这些方法以及如何在不同场景下应用它们。
一、使用 len()
函数
len()
函数是 Python 中最常用和最直接的方式来获取字符串的长度。它返回字符串中字符的数量。
string = "Hello, World!"
length = len(string)
print("The length of the string is:", length)
在上面的代码中,我们定义了一个字符串 string
,然后使用 len()
函数来获取它的长度,并将结果存储在变量 length
中,最后打印出字符串的长度。
二、使用循环迭代
除了 len()
函数,我们还可以通过迭代字符串中的每个字符来计算字符串的长度。这种方法虽然不如 len()
函数简洁,但可以帮助理解字符串的结构。
string = "Hello, World!"
length = 0
for char in string:
length += 1
print("The length of the string is:", length)
在上面的代码中,我们通过一个 for
循环迭代字符串中的每个字符,并在每次迭代时将变量 length
的值加 1。最后,length
变量中存储的值就是字符串的长度。
三、使用正则表达式
正则表达式是一种强大的工具,可以用于字符串匹配和操作。虽然使用正则表达式计算字符串长度并不是最有效的方法,但在某些特殊情况下可能会有所帮助。
import re
string = "Hello, World!"
pattern = re.compile(r'.')
matches = pattern.findall(string)
length = len(matches)
print("The length of the string is:", length)
在上面的代码中,我们使用正则表达式 r'.'
匹配字符串中的每个字符,并使用 re.compile()
函数编译正则表达式。然后,使用 findall()
方法找到所有匹配的字符,并将它们存储在 matches
列表中。最后,通过计算 matches
列表的长度来获取字符串的长度。
四、使用 sys.getsizeof()
方法
虽然 sys.getsizeof()
方法通常用于获取对象的内存大小,但它也可以用于字符串长度的间接判断。需要注意的是,这种方法返回的是对象的内存大小,而不是字符数。
import sys
string = "Hello, World!"
size = sys.getsizeof(string)
print("The size of the string object in bytes is:", size)
在上面的代码中,我们使用 sys.getsizeof()
方法获取字符串对象在内存中的大小,并将其存储在变量 size
中。需要注意的是,这种方法返回的是字节数,而不是字符数。
五、使用 reduce()
函数
reduce()
函数是 functools
模块中的一个高阶函数,它可以对序列中的元素进行累计操作。我们可以使用 reduce()
函数来计算字符串的长度。
from functools import reduce
string = "Hello, World!"
length = reduce(lambda x, y: x + 1, string, 0)
print("The length of the string is:", length)
在上面的代码中,我们使用 reduce()
函数对字符串中的每个字符进行累计操作。初始值为 0,每次迭代时将累计值加 1。最后,length
变量中存储的值就是字符串的长度。
六、使用 map()
函数
map()
函数是另一个高阶函数,它可以对序列中的每个元素应用一个函数。我们可以使用 map()
函数将字符串中的每个字符映射为 1,然后计算这些 1 的总和。
string = "Hello, World!"
length = sum(map(lambda x: 1, string))
print("The length of the string is:", length)
在上面的代码中,我们使用 map()
函数将字符串中的每个字符映射为 1,然后使用 sum()
函数计算这些 1 的总和。最后,length
变量中存储的值就是字符串的长度。
七、使用 collections.Counter
collections
模块中的 Counter
类是一个方便的工具,可以用于计数对象。我们可以使用 Counter
类来计算字符串中的字符数。
from collections import Counter
string = "Hello, World!"
counter = Counter(string)
length = sum(counter.values())
print("The length of the string is:", length)
在上面的代码中,我们使用 Counter
类对字符串中的每个字符进行计数,并将结果存储在 counter
对象中。然后,通过计算 counter
对象中所有值的总和来获取字符串的长度。
八、使用 itertools
itertools
模块提供了一些用于迭代操作的工具。我们可以使用 itertools
模块中的 count()
函数来计算字符串的长度。
import itertools
string = "Hello, World!"
length = sum(1 for _ in itertools.count() if _ < len(string))
print("The length of the string is:", length)
在上面的代码中,我们使用 itertools.count()
函数生成一个无限计数器,然后通过一个生成器表达式来计算字符串的长度。
九、使用递归
递归是一种解决问题的强大技术,它可以将问题分解为更小的子问题。我们可以使用递归来计算字符串的长度。
def string_length(string):
if not string:
return 0
return 1 + string_length(string[1:])
string = "Hello, World!"
length = string_length(string)
print("The length of the string is:", length)
在上面的代码中,我们定义了一个递归函数 string_length()
,它在字符串为空时返回 0,否则返回 1 加上去掉第一个字符后字符串的长度。
十、使用 numpy
numpy
是一个用于科学计算的强大库,虽然它主要用于处理数组,但我们也可以使用它来计算字符串的长度。
import numpy as np
string = "Hello, World!"
length = np.char.str_len(string)
print("The length of the string is:", length)
在上面的代码中,我们使用 numpy
库中的 np.char.str_len()
函数来计算字符串的长度。
十一、使用 pandas
pandas
是一个用于数据分析的强大库,我们可以使用 pandas
库中的 str.len()
方法来计算字符串的长度。
import pandas as pd
string = "Hello, World!"
length = pd.Series([string]).str.len().iloc[0]
print("The length of the string is:", length)
在上面的代码中,我们使用 pandas
库中的 str.len()
方法来计算字符串的长度,并使用 iloc[0]
提取结果。
十二、性能比较
虽然有很多方法可以计算字符串的长度,但它们的性能可能会有所不同。我们可以使用 timeit
模块来比较不同方法的性能。
import timeit
string = "Hello, World!"
len() 方法
time_len = timeit.timeit('len(string)', globals=globals(), number=1000000)
print("len() method:", time_len)
循环迭代
time_loop = timeit.timeit('sum(1 for _ in string)', globals=globals(), number=1000000)
print("Loop iteration:", time_loop)
正则表达式
time_re = timeit.timeit('len(re.findall(".", string))', globals=globals(), setup='import re', number=1000000)
print("Regular expression:", time_re)
递归
time_recursive = timeit.timeit('string_length(string)', globals=globals(), setup='''def string_length(string):
if not string:
return 0
return 1 + string_length(string[1:])''', number=1000000)
print("Recursive function:", time_recursive)
在上面的代码中,我们使用 timeit.timeit()
方法来测量不同方法计算字符串长度的时间。通过比较这些时间,我们可以选择性能最好的方法来计算字符串的长度。
总结
在 Python 中,有多种方法可以用来判断字符串的长度,其中最常用和最直接的方法是使用内置的 len()
函数。其他方法如循环迭代、正则表达式、递归等也可以实现相同的功能,但在性能和简洁性方面可能不如 len()
函数。根据具体需求选择合适的方法,可以更高效地处理字符串长度的判断。
相关问答FAQs:
如何在Python中获取字符串的长度?
在Python中,可以使用内置函数len()
来获取字符串的长度。只需将字符串作为参数传递给len()
,它将返回字符串中的字符数。例如:
my_string = "Hello, World!"
length = len(my_string)
print(length) # 输出: 13
如果字符串包含空格,如何计算长度?
在Python中,len()
函数会计算字符串中的所有字符,包括空格和标点符号。例如,字符串"Hello World"的长度为11,因为它包含了空格。使用len()
时不会忽略这些字符。
my_string = "Hello World"
print(len(my_string)) # 输出: 11
字符串长度的判断在实际应用中有哪些重要性?
判断字符串长度在多个场景中都非常重要。例如,在处理用户输入时,可以确保输入的字符串符合特定的格式或长度要求。此外,在数据验证、密码强度检查以及文本处理等领域,了解字符串长度有助于提高程序的健壮性和用户体验。
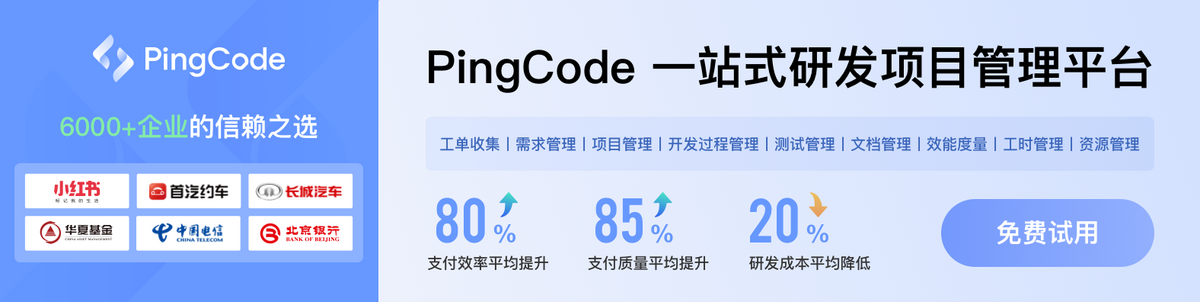