在Python中,使用替换字符串的方法主要有:使用replace()方法、使用re.sub()方法、使用translate()方法。其中,replace()方法是最常用的。以下详细介绍replace()方法的使用。
replace()方法详解:
replace()方法用于将字符串中的某些字符或子字符串替换为其他字符或子字符串。这个方法非常简单易用,适用于大多数字符串替换需求。replace()方法的语法如下:
str.replace(old, new, count)
- old: 需要被替换的旧子字符串。
- new: 替换为的新子字符串。
- count: 可选参数,指定最多替换的次数。如果不指定,默认替换所有匹配的子字符串。
例如:
text = "Hello World! Hello Everyone!"
new_text = text.replace("Hello", "Hi")
print(new_text) # 输出:Hi World! Hi Everyone!
一、replace()方法的基本使用
replace()方法是Python字符串对象自带的方法,可以方便地进行字符串替换。
1. 无限制替换
当不指定count参数时,replace()方法会替换所有匹配的子字符串。
text = "apple apple apple"
new_text = text.replace("apple", "orange")
print(new_text) # 输出:orange orange orange
2. 限制替换次数
通过指定count参数,可以控制替换的次数。
text = "apple apple apple"
new_text = text.replace("apple", "orange", 2)
print(new_text) # 输出:orange orange apple
二、re.sub()方法
在需要更复杂的替换操作时,可以使用re模块的sub()方法。该方法允许使用正则表达式进行替换。
1. 基本用法
import re
text = "Cats are smarter than dogs"
pattern = r'\bcats\b'
replacement = 'dogs'
new_text = re.sub(pattern, replacement, text, flags=re.IGNORECASE)
print(new_text) # 输出:dogs are smarter than dogs
2. 使用回调函数
re.sub()方法还支持使用回调函数进行替换。
import re
def replace_func(match):
return match.group(0).upper()
text = "I love programming"
pattern = r'\b\w+\b'
new_text = re.sub(pattern, replace_func, text)
print(new_text) # 输出:I LOVE PROGRAMMING
三、translate()方法
translate()方法适用于需要替换多个字符时,可以结合str.maketrans()方法使用。
1. 基本用法
text = "hello world"
translation_table = str.maketrans("aeiou", "12345")
new_text = text.translate(translation_table)
print(new_text) # 输出:h2ll4 w4rld
2. 删除字符
可以通过将目标字符映射为空字符串来删除字符。
text = "hello world"
translation_table = str.maketrans("", "", "aeiou")
new_text = text.translate(translation_table)
print(new_text) # 输出:hll wrld
四、字符串替换的应用场景
1. 数据清理
在处理数据时,常常需要将数据中的某些字符或子字符串替换为其他内容。例如,将数据中的特殊字符替换为空字符串。
data = "100,000.00 USD"
clean_data = data.replace(",", "")
print(clean_data) # 输出:100000.00 USD
2. 文本处理
在文本处理中,常需要进行某些特定的字符串替换操作。例如,将文章中的某些敏感词替换为其他词。
text = "This is a secret document."
new_text = text.replace("secret", "classified")
print(new_text) # 输出:This is a classified document.
3. 动态内容生成
在动态生成内容时,可以使用字符串替换来插入变量值。例如,生成包含用户信息的邮件内容。
template = "Hello {name}, your order {order_id} has been shipped."
name = "John"
order_id = "123456"
message = template.replace("{name}", name).replace("{order_id}", order_id)
print(message) # 输出:Hello John, your order 123456 has been shipped.
五、注意事项
在进行字符串替换时,需要注意以下几点:
1. 大小写敏感
replace()方法默认是大小写敏感的。如果需要进行不区分大小写的替换,可以使用re.sub()方法并指定re.IGNORECASE标志。
import re
text = "Hello World"
pattern = r'hello'
replacement = 'Hi'
new_text = re.sub(pattern, replacement, text, flags=re.IGNORECASE)
print(new_text) # 输出:Hi World
2. 防止意外替换
在替换时,需要确保不会意外替换其他内容。例如,使用replace()方法替换子字符串时,可能会替换到意料之外的内容。
text = "catastrophe"
new_text = text.replace("cat", "dog")
print(new_text) # 输出:dogastrophe
为了避免这种情况,可以使用正则表达式匹配完整单词进行替换。
import re
text = "catastrophe"
pattern = r'\bcat\b'
replacement = 'dog'
new_text = re.sub(pattern, replacement, text)
print(new_text) # 输出:catastrophe
六、性能考虑
在处理大量数据或频繁进行字符串替换时,需要注意性能问题。以下是一些优化建议:
1. 使用replace()方法
replace()方法是内置方法,性能较高。在可以满足需求的情况下,尽量使用replace()方法。
2. 使用translate()方法
translate()方法适用于需要替换多个字符的情况,性能优于多个replace()调用。
text = "hello world"
translation_table = str.maketrans("aeiou", "12345")
new_text = text.translate(translation_table)
print(new_text) # 输出:h2ll4 w4rld
3. 使用正则表达式预编译
在使用re.sub()方法时,可以预编译正则表达式,以提高性能。
import re
pattern = re.compile(r'\bcat\b', re.IGNORECASE)
text = "Cats are smarter than dogs"
replacement = 'dogs'
new_text = pattern.sub(replacement, text)
print(new_text) # 输出:dogs are smarter than dogs
七、总结
字符串替换是Python编程中常见的操作,合理选择替换方法可以提高代码的可读性和执行效率。replace()方法适用于简单的字符串替换,re.sub()方法适用于复杂的正则表达式替换,translate()方法适用于多个字符的替换。在实际应用中,需要根据具体需求选择合适的方法,同时注意大小写敏感、避免意外替换等问题,以确保替换操作的正确性和高效性。
相关问答FAQs:
如何在Python中替换字符串中的特定字符或子字符串?
在Python中,可以使用str.replace()
方法来替换字符串中的特定字符或子字符串。该方法接受两个参数,第一个是要被替换的字符或子字符串,第二个是替换成的新字符或子字符串。例如,my_string.replace("旧字符", "新字符")
会返回一个新的字符串,其中所有的“旧字符”都被“新字符”替换。
Python中是否有其他方法可以实现字符串替换?
除了str.replace()
方法,Python还支持使用正则表达式进行更复杂的字符串替换。可以通过re
模块中的re.sub()
函数实现这一功能。此方法允许用户使用正则表达式匹配模式,然后将匹配的部分替换为指定的字符串。这对于处理复杂的字符串模式非常有用。
在Python中替换字符串时,是否会影响原始字符串?
在Python中,字符串是不可变的,意味着每次进行替换操作时,都会生成一个新的字符串而不会修改原始字符串。要保存替换后的结果,用户需要将其赋值给一个新的变量或覆盖原有变量。例如,my_string = my_string.replace("旧字符", "新字符")
将更新my_string
变量,以反映替换后的内容。
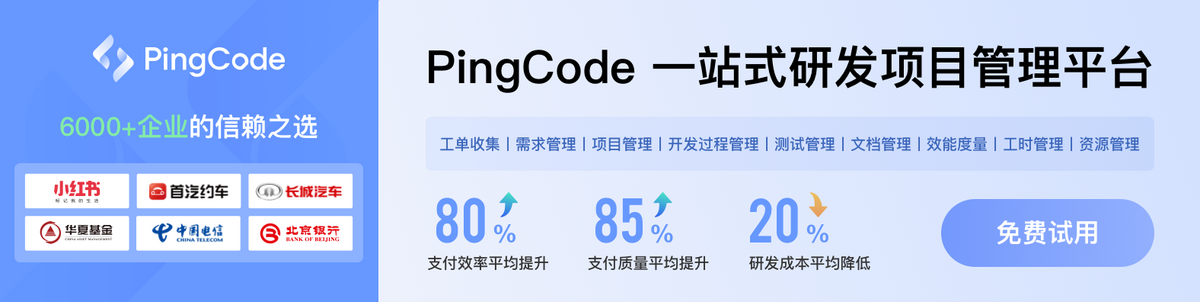