Python可以通过多种方式下载文件,如使用requests
库、urllib
库、以及wget
库等。本文将详细介绍如何使用这些方法,并提供代码示例来帮助你更好地理解和应用。
一、使用requests库下载文件
requests
库是Python中一个流行的HTTP库,可以轻松地发送HTTP请求。使用它下载文件非常简单。首先,你需要安装requests
库:
pip install requests
1、基础用法
你可以使用requests.get
方法下载文件,然后将内容写入本地文件中。
import requests
url = 'https://example.com/somefile.txt'
response = requests.get(url)
with open('somefile.txt', 'wb') as file:
file.write(response.content)
2、处理大文件
对于大文件,建议分块下载以避免内存占用过高。
import requests
url = 'https://example.com/largefile.zip'
response = requests.get(url, stream=True)
with open('largefile.zip', 'wb') as file:
for chunk in response.iter_content(chunk_size=8192):
file.write(chunk)
二、使用urllib库下载文件
urllib
是Python内置的HTTP库,可以用来处理URL以及下载文件。你无需额外安装第三方库。
1、基础用法
使用urllib.request.urlretrieve
方法可以直接下载文件并保存到本地。
import urllib.request
url = 'https://example.com/somefile.txt'
urllib.request.urlretrieve(url, 'somefile.txt')
2、处理大文件
对于大文件,可以使用urllib.request.urlopen
方法和shutil.copyfileobj
方法进行分块下载。
import urllib.request
import shutil
url = 'https://example.com/largefile.zip'
with urllib.request.urlopen(url) as response, open('largefile.zip', 'wb') as out_file:
shutil.copyfileobj(response, out_file)
三、使用wget库下载文件
wget
库是一个Python封装的库,提供类似于Linux中wget
命令的功能。你需要先安装wget
库:
pip install wget
1、基础用法
使用wget.download
方法可以直接下载文件。
import wget
url = 'https://example.com/somefile.txt'
wget.download(url, 'somefile.txt')
四、使用第三方库(如aiohttp)进行异步下载
如果你需要同时下载多个文件,异步下载可以极大地提高效率。aiohttp
是一个支持异步HTTP请求的库。
1、安装aiohttp
pip install aiohttp
2、异步下载示例
使用aiohttp
进行异步下载,需要编写异步函数。
import aiohttp
import asyncio
async def download_file(url, filename):
async with aiohttp.ClientSession() as session:
async with session.get(url) as response:
with open(filename, 'wb') as file:
while True:
chunk = await response.content.read(1024)
if not chunk:
break
file.write(chunk)
async def main():
urls = [
('https://example.com/file1.txt', 'file1.txt'),
('https://example.com/file2.txt', 'file2.txt'),
]
tasks = [download_file(url, filename) for url, filename in urls]
await asyncio.gather(*tasks)
asyncio.run(main())
五、处理下载过程中的错误
在下载文件时,可能会遇到各种错误,如网络问题、文件不存在等。你可以使用异常处理来捕获和处理这些错误。
1、使用requests处理错误
import requests
url = 'https://example.com/somefile.txt'
try:
response = requests.get(url)
response.raise_for_status()
with open('somefile.txt', 'wb') as file:
file.write(response.content)
except requests.exceptions.RequestException as e:
print(f"Error downloading file: {e}")
2、使用urllib处理错误
import urllib.request
url = 'https://example.com/somefile.txt'
try:
urllib.request.urlretrieve(url, 'somefile.txt')
except urllib.error.URLError as e:
print(f"Error downloading file: {e}")
六、总结
通过以上几种方法,你可以在Python中轻松地下载文件。根据具体需求选择合适的库和方法,可以让你的文件下载任务更加高效和可靠。对于大文件下载,建议使用分块下载以避免内存占用过高;在下载过程中,处理可能出现的错误可以提高程序的健壮性。希望本文对你有所帮助。
相关问答FAQs:
如何使用Python下载特定类型的文件?
在Python中,下载特定类型的文件(如图片、PDF或文本文件)可以使用requests
库。首先,你需要安装这个库,可以通过pip install requests
来安装。接着,使用requests.get()
方法获取文件的内容,并将其写入本地文件中。例如,对于下载一张图片,可以使用如下代码:
import requests
url = 'https://example.com/image.jpg'
response = requests.get(url)
with open('image.jpg', 'wb') as file:
file.write(response.content)
这样就能将指定网址的图片文件下载到本地。
在下载文件时如何处理异常情况?
在下载文件的过程中,可能会出现网络问题或链接失效等异常情况。为了确保程序的稳健性,建议使用try
和except
语句来捕捉这些异常。可以通过检查响应状态码来确认下载是否成功。如果状态码不是200,表示请求失败,程序应进行相应的处理。例如:
try:
response = requests.get(url)
response.raise_for_status() # 检查请求是否成功
with open('file.pdf', 'wb') as file:
file.write(response.content)
except requests.exceptions.HTTPError as err:
print(f'HTTP错误: {err}')
except Exception as e:
print(f'其他错误: {e}')
这样可以有效防止程序因错误而崩溃。
如何下载大文件并显示下载进度?
下载大文件时,通常希望能够查看下载进度。可以通过stream=True
参数来逐块下载文件,并计算下载进度。以下是一个示例代码:
url = 'https://example.com/largefile.zip'
response = requests.get(url, stream=True)
total_size = int(response.headers.get('content-length', 0))
with open('largefile.zip', 'wb') as file:
for data in response.iter_content(chunk_size=1024):
file.write(data)
downloaded_size = file.tell()
print(f'下载进度: {downloaded_size / total_size * 100:.2f}%')
这种方法不仅能有效处理大文件下载,还能实时反馈用户下载的进度。
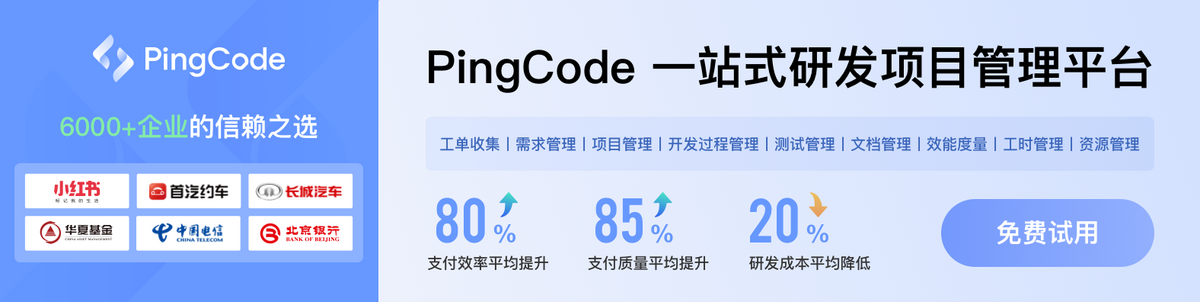