Python读取C盘中的文件可以通过几种常见的方法来实现,例如使用内置的 open()
函数、os
模块或者 pandas
库来读取不同格式的文件。 在本文中,我们将详细介绍这些方法,并列举具体的代码示例。
一、使用 open()
函数读取文件
Python 提供了一个内置的 open()
函数,用于打开文件。通过 open()
函数,我们可以读取文本文件和二进制文件。
1.1 打开并读取文本文件
# 打开并读取文本文件
file_path = r"C:\path\to\your\file.txt"
with open(file_path, 'r', encoding='utf-8') as file:
content = file.read()
print(content)
在上面的代码中,我们使用 open()
函数打开了一个文件,并指定了文件路径和读取模式('r'
表示读取模式)。通过 with
语句,确保文件在读取完毕后自动关闭,避免资源泄露。
1.2 按行读取文本文件
如果文件较大,可以按行读取文件内容:
file_path = r"C:\path\to\your\file.txt"
with open(file_path, 'r', encoding='utf-8') as file:
for line in file:
print(line.strip()) # 去除行尾的换行符
1.3 读取二进制文件
对于二进制文件,可以使用 'rb'
模式:
file_path = r"C:\path\to\your\file.jpg"
with open(file_path, 'rb') as file:
content = file.read()
print(content)
二、使用 os
模块读取文件
Python 的 os
模块提供了与操作系统进行交互的功能,可以用于读取文件。
2.1 使用 os.path
检查文件是否存在
在读取文件之前,最好先检查文件是否存在:
import os
file_path = r"C:\path\to\your\file.txt"
if os.path.exists(file_path):
with open(file_path, 'r', encoding='utf-8') as file:
content = file.read()
print(content)
else:
print(f"The file {file_path} does not exist.")
2.2 使用 os
模块遍历目录并读取文件
我们可以使用 os.listdir()
列出目录中的所有文件,并逐一读取:
import os
directory_path = r"C:\path\to\your\directory"
for filename in os.listdir(directory_path):
file_path = os.path.join(directory_path, filename)
if os.path.isfile(file_path):
with open(file_path, 'r', encoding='utf-8') as file:
content = file.read()
print(f"Content of {filename}:\n{content}\n")
三、使用 pandas
库读取文件
如果要读取 CSV 或 Excel 文件,pandas
库是一个非常强大的工具。
3.1 读取 CSV 文件
import pandas as pd
file_path = r"C:\path\to\your\file.csv"
df = pd.read_csv(file_path)
print(df.head()) # 打印前五行内容
3.2 读取 Excel 文件
import pandas as pd
file_path = r"C:\path\to\your\file.xlsx"
df = pd.read_excel(file_path)
print(df.head()) # 打印前五行内容
四、处理文件读取中的常见问题
4.1 文件路径中的反斜杠
在 Windows 系统中,文件路径使用反斜杠(),但在 Python 中,反斜杠是转义字符。所以,最好使用原始字符串(在字符串前加
r
)或者双反斜杠:
file_path = r"C:\path\to\your\file.txt" # 使用原始字符串
或者
file_path = "C:\\path\\to\\your\\file.txt" # 使用双反斜杠
4.2 文件编码问题
读取文本文件时,可能会遇到编码问题。常见的编码包括 UTF-8 和 GBK。可以在 open()
函数中指定编码:
with open(file_path, 'r', encoding='utf-8') as file:
content = file.read()
五、总结
Python 提供了多种读取文件的方法,包括内置的 open()
函数、os
模块和 pandas
库。在选择具体方法时,需要根据文件的格式和实际需求来决定。 open()
函数适合读取文本文件和二进制文件,os
模块适合与操作系统进行交互,而 pandas
库则适合处理结构化数据文件,如 CSV 和 Excel 文件。
通过本文的详细介绍,希望你能对 Python 读取 C 盘中的文件有一个全面的了解,并能在实际项目中灵活运用这些方法。
相关问答FAQs:
如何使用Python读取C盘中的特定文件类型?
在Python中,可以使用内置的open()
函数来读取特定文件类型,例如文本文件、CSV文件等。可以通过指定文件路径来读取C盘中的文件。示例代码如下:
with open('C:/path/to/your/file.txt', 'r') as file:
content = file.read()
print(content)
确保文件路径正确且文件存在,以避免出现FileNotFoundError。
在Python中读取C盘文件时,如何处理编码问题?
读取文件时,编码格式可能会导致问题。可以在open()
函数中指定encoding
参数,例如:
with open('C:/path/to/your/file.txt', 'r', encoding='utf-8') as file:
content = file.read()
常见的编码格式包括utf-8
和gbk
,选择合适的编码可以确保文件内容正确读取。
使用Python读取C盘中文件时,有哪些常见的错误及其解决方法?
在读取文件时,常见的错误包括FileNotFoundError(文件未找到)、PermissionError(权限错误)等。可以通过以下方式解决:
- 确保提供的文件路径是正确的,文件确实存在于指定位置。
- 检查文件的访问权限,确保当前用户有权限读取该文件。
- 使用异常处理来捕捉错误并提供友好的提示,示例如下:
try:
with open('C:/path/to/your/file.txt', 'r') as file:
content = file.read()
except FileNotFoundError:
print("文件未找到,请检查路径。")
except PermissionError:
print("权限不足,无法读取文件。")
通过这些措施,可以有效提高文件读取的成功率。
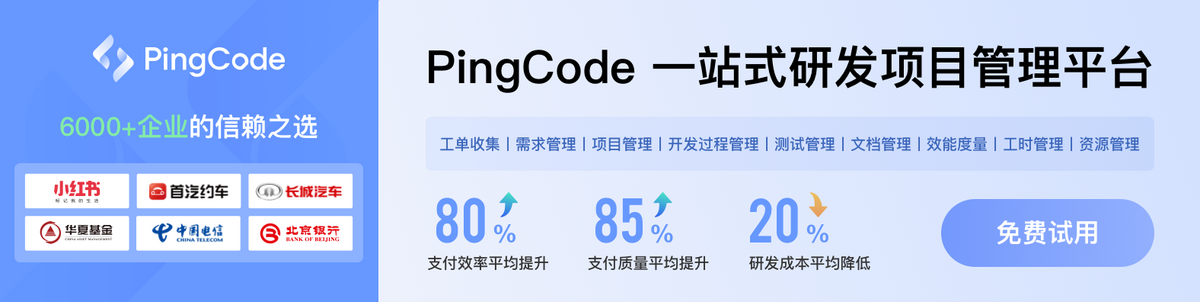