Python获取网页中的HTML元素方法有以下几种:使用requests库获取网页内容、使用BeautifulSoup解析HTML、使用lxml解析HTML、使用Selenium进行动态网页抓取。在这几种方法中,requests和BeautifulSoup是最常用的方式。下面将详细介绍如何使用requests库获取网页内容,并使用BeautifulSoup解析HTML元素。
一、使用Requests库获取网页内容
Requests是一个简单易用的HTTP库,用于发送HTTP请求。通过requests库,我们可以轻松地获取网页的HTML内容。
import requests
url = 'http://example.com'
response = requests.get(url)
if response.status_code == 200:
html_content = response.text
print(html_content)
else:
print('Failed to retrieve webpage')
在上面的代码中,我们首先导入requests库,然后通过requests.get()方法发送GET请求获取网页内容。通过检查响应状态码,我们可以确定请求是否成功,成功后可以获取网页的HTML内容。
二、使用BeautifulSoup解析HTML
BeautifulSoup是一个用于解析HTML和XML文档的Python库。通过BeautifulSoup,我们可以方便地提取网页中的HTML元素。
1、安装BeautifulSoup库
pip install beautifulsoup4
pip install lxml
2、解析HTML并提取元素
from bs4 import BeautifulSoup
import requests
url = 'http://example.com'
response = requests.get(url)
if response.status_code == 200:
html_content = response.text
soup = BeautifulSoup(html_content, 'lxml')
# 提取标题
title = soup.title.string
print('Title:', title)
# 提取所有段落
paragraphs = soup.find_all('p')
for p in paragraphs:
print(p.text)
else:
print('Failed to retrieve webpage')
在上面的代码中,我们首先获取网页内容,然后使用BeautifulSoup解析HTML内容。通过soup.title.string获取网页标题,通过soup.find_all('p')获取所有段落元素并打印其内容。
三、使用lxml解析HTML
lxml是一个高性能的Python库,用于解析和处理XML和HTML文档。通过lxml,我们可以高效地解析和提取HTML元素。
1、安装lxml库
pip install lxml
2、解析HTML并提取元素
from lxml import etree
import requests
url = 'http://example.com'
response = requests.get(url)
if response.status_code == 200:
html_content = response.text
parser = etree.HTMLParser()
tree = etree.fromstring(html_content, parser)
# 提取标题
title = tree.xpath('//title/text()')[0]
print('Title:', title)
# 提取所有段落
paragraphs = tree.xpath('//p/text()')
for p in paragraphs:
print(p)
else:
print('Failed to retrieve webpage')
在上面的代码中,我们首先获取网页内容,然后使用etree.HTMLParser()解析HTML内容。通过tree.xpath('//title/text()')获取网页标题,通过tree.xpath('//p/text()')获取所有段落元素并打印其内容。
四、使用Selenium进行动态网页抓取
Selenium是一个用于自动化Web浏览器操作的工具。通过Selenium,我们可以处理动态网页并提取HTML元素。
1、安装Selenium库和WebDriver
pip install selenium
下载适用于您浏览器的WebDriver,例如ChromeDriver,并将其添加到系统路径中。
2、使用Selenium进行网页抓取
from selenium import webdriver
from selenium.webdriver.common.by import By
url = 'http://example.com'
driver = webdriver.Chrome()
driver.get(url)
提取标题
title = driver.title
print('Title:', title)
提取所有段落
paragraphs = driver.find_elements(By.TAG_NAME, 'p')
for p in paragraphs:
print(p.text)
driver.quit()
在上面的代码中,我们首先导入Selenium库并启动Chrome浏览器。通过driver.get(url)访问网页,并通过driver.title获取网页标题。通过driver.find_elements(By.TAG_NAME, 'p')获取所有段落元素并打印其内容。最后,通过driver.quit()关闭浏览器。
总结
通过本文,我们介绍了如何使用Python获取网页中的HTML元素。我们详细介绍了四种方法:使用requests库获取网页内容、使用BeautifulSoup解析HTML、使用lxml解析HTML、使用Selenium进行动态网页抓取。Requests和BeautifulSoup是最常用的方式,适用于大多数静态网页抓取需求。lxml适用于高性能解析需求,而Selenium适用于处理动态网页。希望本文对您有所帮助,并能帮助您更好地抓取和解析网页内容。
相关问答FAQs:
如何使用Python库获取网页的HTML内容?
在Python中,可以使用多个库来获取网页的HTML内容,例如requests
和BeautifulSoup
。使用requests
库可以轻松发送HTTP请求并获取响应内容,而BeautifulSoup
则用于解析和提取HTML元素。以下是一个简单的示例:
import requests
from bs4 import BeautifulSoup
url = 'https://example.com'
response = requests.get(url)
soup = BeautifulSoup(response.text, 'html.parser')
print(soup.prettify())
这个代码段会打印出网页的格式化HTML内容,方便后续的分析和提取。
如何提取特定的HTML元素?
在获取到网页的HTML后,可以使用BeautifulSoup
提供的方法来提取特定的HTML元素。例如,如果想要获取所有的<h1>
标签,可以使用soup.find_all('h1')
。以下是一个示例:
h1_tags = soup.find_all('h1')
for tag in h1_tags:
print(tag.text)
通过这种方式,可以轻松地提取网页中特定的元素和它们的文本。
获取动态加载的HTML内容时应如何处理?
有些网页的内容是通过JavaScript动态加载的,使用requests
库无法直接获取这些内容。在这种情况下,可以考虑使用Selenium
库,它可以模拟浏览器的操作,从而获取动态加载的HTML。以下是一个简单的使用示例:
from selenium import webdriver
driver = webdriver.Chrome()
driver.get('https://example.com')
html_content = driver.page_source
print(html_content)
driver.quit()
通过这种方式,可以获取到页面中所有的动态内容,适合需要处理复杂网页的场景。
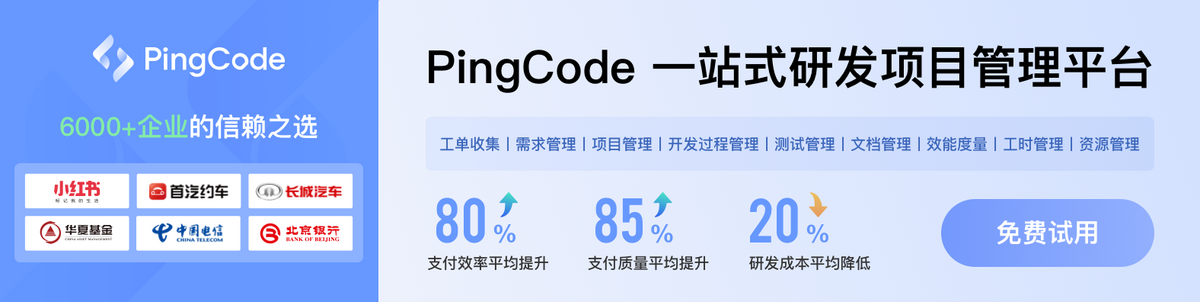