如何用Python做圆柱体教程
使用Python进行圆柱体建模可以通过多个库和工具实现,如matplotlib、numpy、和mayavi等,每个工具都有其独特的优势和应用场景。在这篇文章中,我们将详细介绍如何使用这些工具来创建和展示一个圆柱体模型。
一、使用Matplotlib绘制圆柱体
Matplotlib是Python中一个非常强大的2D绘图库,尽管它主要用于生成静态的图表,但也可以用于3D绘图。
1.1、安装和导入必要的库
首先,我们需要安装并导入必要的库,包括matplotlib和numpy:
pip install matplotlib numpy
import matplotlib.pyplot as plt
import numpy as np
from mpl_toolkits.mplot3d import Axes3D
1.2、生成圆柱体的坐标
我们需要生成圆柱体的顶面和底面的坐标:
height = 10
radius = 5
resolution = 100
theta = np.linspace(0, 2 * np.pi, resolution)
z = np.linspace(0, height, resolution)
theta, z = np.meshgrid(theta, z)
x = radius * np.cos(theta)
y = radius * np.sin(theta)
1.3、绘制圆柱体
使用matplotlib的3D绘图功能,我们可以绘制出圆柱体:
fig = plt.figure()
ax = fig.add_subplot(111, projection='3d')
ax.plot_surface(x, y, z, alpha=0.7, rstride=100, cstride=100)
ax.set_xlabel('X')
ax.set_ylabel('Y')
ax.set_zlabel('Z')
plt.show()
二、使用Mayavi绘制圆柱体
Mayavi是一个功能强大的3D科学数据可视化工具,适合用于更复杂的3D绘图。
2.1、安装和导入必要的库
首先,我们需要安装mayavi:
pip install mayavi
然后导入必要的库:
from mayavi import mlab
import numpy as np
2.2、生成圆柱体的坐标
与matplotlib类似,我们需要生成圆柱体的顶面和底面的坐标:
height = 10
radius = 5
resolution = 100
theta = np.linspace(0, 2 * np.pi, resolution)
z = np.linspace(0, height, resolution)
theta, z = np.meshgrid(theta, z)
x = radius * np.cos(theta)
y = radius * np.sin(theta)
2.3、绘制圆柱体
使用mayavi的mlab模块来绘制圆柱体:
mlab.mesh(x, y, z)
mlab.show()
三、使用Numpy生成圆柱体数据
有时候,我们可能需要在没有可视化工具的情况下生成圆柱体的数据,Numpy可以帮助我们实现这一点。
3.1、生成圆柱体的顶面和底面的坐标
使用Numpy生成圆柱体的顶面和底面的坐标:
height = 10
radius = 5
resolution = 100
theta = np.linspace(0, 2 * np.pi, resolution)
z = np.linspace(0, height, resolution)
theta, z = np.meshgrid(theta, z)
x = radius * np.cos(theta)
y = radius * np.sin(theta)
3.2、保存数据
可以使用Numpy将生成的数据保存到文件中,以便于后续处理:
np.savez('cylinder_data.npz', x=x, y=y, z=z)
3.3、加载数据
在需要的时候,可以随时加载这些数据:
data = np.load('cylinder_data.npz')
x = data['x']
y = data['y']
z = data['z']
四、总结
通过上述方法,我们可以使用Python中的不同库和工具实现圆柱体的建模和可视化。Matplotlib适合用于简单的3D绘图,Mayavi适合用于更复杂的3D科学数据可视化,而Numpy则可以用于生成和处理3D数据。根据具体的需求和应用场景,可以选择合适的工具来实现圆柱体的建模。
在实际应用中,可能需要根据项目的具体需求进行更多的参数调整和优化。此外,熟悉这些工具的使用方法和技巧,可以帮助我们更高效地进行3D数据的处理和可视化。希望这篇教程能够帮助到大家,更好地理解和掌握如何使用Python进行圆柱体的建模和绘制。
相关问答FAQs:
如何在Python中绘制圆柱体的图形?
要在Python中绘制圆柱体的图形,可以使用Matplotlib库。首先,安装Matplotlib库,然后使用3D绘图工具创建圆柱体。以下是一个简单的示例代码:
import numpy as np
import matplotlib.pyplot as plt
from mpl_toolkits.mplot3d import Axes3D
fig = plt.figure()
ax = fig.add_subplot(111, projection='3d')
z = np.linspace(0, 1, 100)
theta = np.linspace(0, 2 * np.pi, 100)
theta_grid, z_grid = np.meshgrid(theta, z)
x_grid = np.cos(theta_grid)
y_grid = np.sin(theta_grid)
ax.plot_surface(x_grid, y_grid, z_grid)
ax.set_xlabel('X')
ax.set_ylabel('Y')
ax.set_zlabel('Z')
plt.show()
运行这段代码即可在3D空间中看到圆柱体的效果。
在Python中如何计算圆柱体的体积和表面积?
计算圆柱体的体积和表面积可以使用以下公式:
- 体积 V = πr²h
- 表面积 A = 2πrh + 2πr²
在Python中,可以使用math库来实现这些计算。以下是示例代码:
import math
def cylinder_volume(radius, height):
return math.pi * radius ** 2 * height
def cylinder_surface_area(radius, height):
return 2 * math.pi * radius * height + 2 * math.pi * radius ** 2
radius = 5
height = 10
print("体积:", cylinder_volume(radius, height))
print("表面积:", cylinder_surface_area(radius, height))
这段代码将输出指定半径和高度的圆柱体的体积和表面积。
在Python中如何处理圆柱体的用户输入?
通过使用input函数,可以让用户输入圆柱体的半径和高度。接着,可以将这些输入值传递给计算体积和表面积的函数。下面是一个示例:
radius = float(input("请输入圆柱体的半径: "))
height = float(input("请输入圆柱体的高度: "))
print("圆柱体的体积为:", cylinder_volume(radius, height))
print("圆柱体的表面积为:", cylinder_surface_area(radius, height))
这段代码不仅计算圆柱体的体积和表面积,还能根据用户的输入动态生成结果。
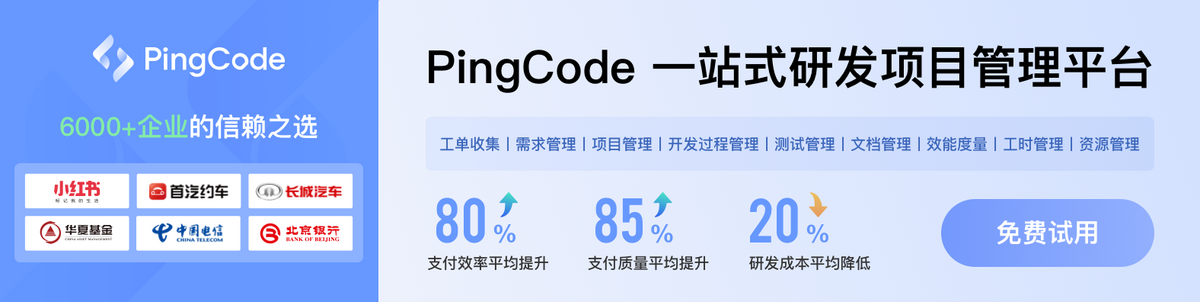