Python 初始化矩形顶点坐标的方法有很多种,常用的包括:直接定义顶点坐标、使用类封装、通过函数生成、利用库函数等。 其中,最简单的方式是直接定义顶点坐标,也就是手动输入矩形的四个顶点。本文将详细介绍这些不同的方法,并提供相应的示例代码。
一、直接定义顶点坐标
直接定义顶点坐标是最直接、最简单的方法。假设我们有一个矩形,其左上角的顶点坐标为 (x1, y1),右下角的顶点坐标为 (x2, y2),我们可以直接定义这四个顶点的坐标。
# 左上角
x1, y1 = 1, 2
右下角
x2, y2 = 4, 5
计算其他两个顶点
x3, y3 = x1, y2
x4, y4 = x2, y1
矩形的四个顶点坐标
vertices = [(x1, y1), (x3, y3), (x2, y2), (x4, y4)]
print(vertices)
这种方式适用于简单的情况,当矩形的顶点坐标已知时,可以直接使用。
二、使用类封装
为了更好的组织代码和提高可读性,我们可以使用类来封装矩形顶点坐标的初始化。这样不仅可以更方便地管理矩形对象,还可以在类中添加更多的功能。
class Rectangle:
def __init__(self, x1, y1, x2, y2):
self.x1, self.y1 = x1, y1
self.x2, self.y2 = x2, y2
self.vertices = self.calculate_vertices()
def calculate_vertices(self):
x3, y3 = self.x1, self.y2
x4, y4 = self.x2, self.y1
return [(self.x1, self.y1), (x3, y3), (self.x2, self.y2), (x4, y4)]
def __repr__(self):
return f"Rectangle vertices: {self.vertices}"
创建矩形对象
rect = Rectangle(1, 2, 4, 5)
print(rect)
使用类封装后,矩形的顶点坐标初始化和管理变得更加方便。
三、通过函数生成
如果你不需要创建一个类来管理矩形,那么可以使用一个简单的函数来生成矩形的顶点坐标。
def create_rectangle_vertices(x1, y1, x2, y2):
x3, y3 = x1, y2
x4, y4 = x2, y1
return [(x1, y1), (x3, y3), (x2, y2), (x4, y4)]
vertices = create_rectangle_vertices(1, 2, 4, 5)
print(vertices)
这种方法适用于只需要一次性生成矩形顶点的情况,不需要创建复杂的类结构。
四、利用库函数
在一些复杂的应用中,可以利用第三方库来初始化矩形顶点坐标。例如,使用 numpy
来处理矩形的顶点坐标。
import numpy as np
def create_rectangle_using_numpy(x1, y1, x2, y2):
vertices = np.array([[x1, y1], [x1, y2], [x2, y2], [x2, y1]])
return vertices
vertices = create_rectangle_using_numpy(1, 2, 4, 5)
print(vertices)
使用 numpy
可以方便地进行矩阵操作,适合需要进行大量矩形坐标处理的情况。
五、综合应用
在实际应用中,可能需要结合以上几种方法来初始化矩形顶点坐标。例如,在一个绘图程序中,你可能需要定义多个矩形,并且需要对这些矩形进行操作和管理。
import numpy as np
class Rectangle:
def __init__(self, x1, y1, x2, y2):
self.vertices = np.array([[x1, y1], [x1, y2], [x2, y2], [x2, y1]])
def translate(self, dx, dy):
self.vertices += np.array([dx, dy])
def __repr__(self):
return f"Rectangle vertices: {self.vertices.tolist()}"
创建多个矩形对象
rect1 = Rectangle(1, 2, 4, 5)
rect2 = Rectangle(2, 3, 5, 6)
平移矩形
rect1.translate(1, 1)
rect2.translate(2, 2)
print(rect1)
print(rect2)
这种综合应用可以更灵活地处理矩形顶点的初始化和操作,适用于复杂的应用场景。
总结一下,初始化矩形顶点坐标的方法有很多种,最简单的是直接定义顶点坐标,使用类封装可以提高代码的组织性,通过函数生成适用于一次性生成顶点的情况,利用库函数可以方便地进行矩阵操作,综合应用可以结合多种方法来处理复杂的应用场景。 选择合适的方法取决于具体的应用需求和代码复杂度。
相关问答FAQs:
如何在Python中定义矩形的顶点坐标?
在Python中,可以使用一个简单的元组或列表来定义矩形的四个顶点坐标。通常,矩形的顶点可以用左下角和右上角的坐标来表示。例如,可以将矩形的左下角定义为 (x1, y1)
,右上角定义为 (x2, y2)
。这样,矩形的其他两个顶点分别是 (x1, y2)
和 (x2, y1)
。以下是一个示例代码:
# 定义矩形的左下角和右上角坐标
bottom_left = (1, 2)
top_right = (4, 5)
# 计算其他两个顶点
top_left = (bottom_left[0], top_right[1])
bottom_right = (top_right[0], bottom_left[1])
print("矩形的顶点坐标为:", bottom_left, top_left, top_right, bottom_right)
在使用Python进行图形编程时,如何有效管理矩形的坐标?
在进行图形编程时,使用类来管理矩形的坐标是一种有效的方法。创建一个矩形类,可以让你更加清晰地处理矩形的属性和方法。例如,类可以包含初始化函数、计算面积和周长的方法等。这样可以提高代码的可读性和重用性。以下是一个示例:
class Rectangle:
def __init__(self, bottom_left, top_right):
self.bottom_left = bottom_left
self.top_right = top_right
def area(self):
width = self.top_right[0] - self.bottom_left[0]
height = self.top_right[1] - self.bottom_left[1]
return width * height
def perimeter(self):
width = self.top_right[0] - self.bottom_left[0]
height = self.top_right[1] - self.bottom_left[1]
return 2 * (width + height)
# 使用矩形类
rect = Rectangle((1, 2), (4, 5))
print("矩形的面积:", rect.area())
print("矩形的周长:", rect.perimeter())
如何在Python中处理矩形的坐标转换?
在某些应用中,可能需要对矩形的坐标进行转换,例如从屏幕坐标系到数学坐标系。在Python中,可以使用简单的数学公式进行转换。例如,如果屏幕坐标的原点在左上角而数学坐标的原点在左下角,则需要对y坐标进行转换。以下是一个示例:
def convert_to_math_coordinates(screen_coords, screen_height):
x, y = screen_coords
# 将y坐标进行转换
y_math = screen_height - y
return (x, y_math)
# 示例
screen_height = 600
screen_coord = (100, 200)
math_coord = convert_to_math_coordinates(screen_coord, screen_height)
print("转换后的数学坐标为:", math_coord)
通过这些方式,可以有效地初始化和管理矩形的顶点坐标,同时进行必要的坐标转换,帮助你更好地处理图形编程中的矩形问题。
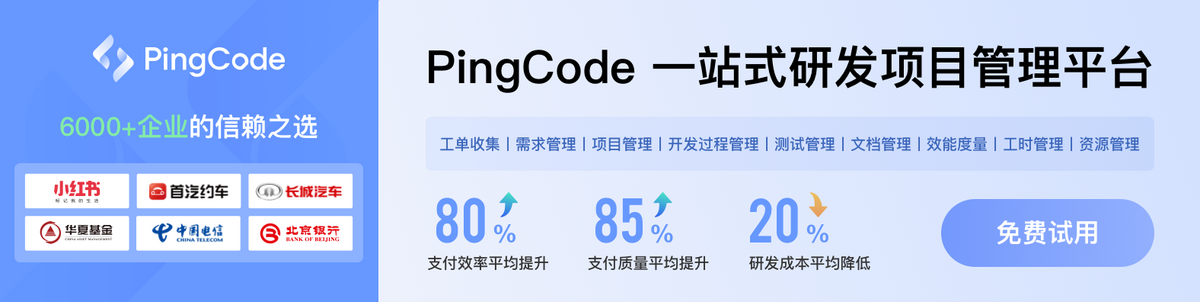