Python可以通过多种方法将输出的字居中,例如使用字符串的内置方法center()
、格式化字符串以及第三方库如rich
。 推荐使用字符串的内置方法center()
,因为它简洁且功能强大。下面会详细介绍如何使用这些方法来实现文字居中。
一、使用center()
方法
Python的字符串方法center()
可以让你非常方便地将字符串居中。center()
方法需要两个参数:一个是字符串的总长度,另一个是填充字符(默认为空格)。使用这个方法,你可以很容易地将文本居中。下面是一个简单的例子:
text = "Hello, World!"
width = 20
centered_text = text.center(width)
print(centered_text)
在这个例子中,"Hello, World!"
将会被居中在一个宽度为20的字符串中,剩余的空白部分将会被填充空格。你也可以使用其他字符来填充,例如:
centered_text = text.center(width, '*')
print(centered_text)
这将会在空白部分填充星号(*
)。
二、使用格式化字符串
Python的格式化字符串提供了更灵活的方式来将文本居中。你可以使用f-string
以及格式化说明符来实现这一点。下面是一个例子:
text = "Hello, World!"
width = 20
centered_text = f"{text:^{width}}"
print(centered_text)
在这个例子中,{text:^{width}}
中的^
符号表示居中对齐,width
表示总宽度。
三、使用format()
方法
format()
方法也可以用来将字符串居中。与f-string
类似,你可以使用格式化说明符来控制文本的对齐方式:
text = "Hello, World!"
width = 20
centered_text = "{:^{width}}".format(text, width=width)
print(centered_text)
四、使用第三方库rich
如果你需要更复杂的文本处理功能,例如在终端中输出彩色文本,可以使用第三方库rich
。rich
库提供了丰富的功能来处理文本格式,包括文本居中:
from rich.console import Console
from rich.text import Text
console = Console()
text = Text("Hello, World!")
console.print(text.center(20))
在这个例子中,我们使用rich
库的Text
对象来创建一个可以居中的文本,并使用console.print()
来输出它。
五、在GUI应用中的居中显示
如果你在开发GUI应用,文本居中显示的方式可能会有所不同。以下是一些常用的GUI库及其实现方法:
1、Tkinter
在Tkinter中,你可以使用Label
组件并设置anchor
和justify
属性来实现文本居中:
import tkinter as tk
root = tk.Tk()
label = tk.Label(root, text="Hello, World!", width=20, anchor='center', justify='center')
label.pack()
root.mainloop()
2、PyQt5
在PyQt5中,你可以使用QLabel
组件并设置其对齐方式:
from PyQt5.QtWidgets import QApplication, QLabel, QWidget, QVBoxLayout
from PyQt5.QtCore import Qt
app = QApplication([])
window = QWidget()
layout = QVBoxLayout()
label = QLabel("Hello, World!")
label.setAlignment(Qt.AlignCenter)
layout.addWidget(label)
window.setLayout(layout)
window.show()
app.exec_()
六、在HTML和Web应用中的居中显示
如果你在开发Web应用,通常会使用HTML和CSS来实现文本居中。以下是一些常用的方法:
1、使用CSS
你可以使用CSS的text-align
属性来将文本居中:
<!DOCTYPE html>
<html>
<head>
<style>
.centered {
text-align: center;
}
</style>
</head>
<body>
<div class="centered">Hello, World!</div>
</body>
</html>
2、使用Flexbox
Flexbox是一种强大的布局模型,可以用来实现各种对齐方式,包括文本居中:
<!DOCTYPE html>
<html>
<head>
<style>
.container {
display: flex;
justify-content: center;
align-items: center;
height: 100vh;
}
</style>
</head>
<body>
<div class="container">
<div>Hello, World!</div>
</div>
</body>
</html>
七、在文档中的居中显示
如果你在编写文档,可能会使用Markdown或LaTeX来格式化文本。以下是一些常用的方法:
1、Markdown
Markdown本身并不支持文本居中,但你可以使用HTML标签来实现:
<p style="text-align: center;">Hello, World!</p>
2、LaTeX
在LaTeX中,你可以使用center
环境来将文本居中:
\documentclass{article}
\begin{document}
\begin{center}
Hello, World!
\end{center}
\end{document}
八、在日志文件中的居中显示
如果你在编写日志文件,可能需要将一些关键信息居中显示,以便更容易阅读。你可以使用字符串的center()
方法来实现这一点:
log_message = "ERROR: Something went wrong"
width = 50
centered_log_message = log_message.center(width, '-')
print(centered_log_message)
在这个例子中,日志消息将会被居中,并且空白部分将会被填充为破折号(-
)。
九、在数据分析中的居中显示
如果你在进行数据分析,可能需要将一些数值或文本居中显示,以便于查看。例如,在使用Pandas库时,可以将DataFrame中的某一列居中显示:
import pandas as pd
data = {'Name': ['Alice', 'Bob', 'Charlie'],
'Age': [25, 30, 35]}
df = pd.DataFrame(data)
Apply a custom function to center the text
df['Name'] = df['Name'].apply(lambda x: x.center(10))
print(df)
十、在报表生成中的居中显示
如果你在生成报表,可能需要将一些关键信息居中显示。例如,在使用ReportLab库生成PDF报表时,可以将文本居中:
from reportlab.lib.pagesizes import letter
from reportlab.pdfgen import canvas
c = canvas.Canvas("report.pdf", pagesize=letter)
width, height = letter
text = "Hello, World!"
Calculate the position to center the text
text_width = c.stringWidth(text, "Helvetica", 12)
x_position = (width - text_width) / 2
c.drawString(x_position, height / 2, text)
c.save()
十一、在游戏开发中的居中显示
如果你在开发游戏,可能需要将一些UI元素或文本居中显示。例如,在使用Pygame库时,可以将文本居中:
import pygame
pygame.init()
screen = pygame.display.set_mode((800, 600))
font = pygame.font.Font(None, 74)
text = font.render("Hello, World!", True, (255, 255, 255))
text_rect = text.get_rect(center=(400, 300))
running = True
while running:
for event in pygame.event.get():
if event.type == pygame.QUIT:
running = False
screen.fill((0, 0, 0))
screen.blit(text, text_rect)
pygame.display.flip()
pygame.quit()
十二、在命令行工具中的居中显示
如果你在编写命令行工具,可能需要将一些输出信息居中显示,以便于用户阅读。例如,在使用click
库时,可以将一些输出信息居中:
import click
@click.command()
def hello():
text = "Hello, World!"
width = click.get_terminal_size()[0]
centered_text = text.center(width)
click.echo(centered_text)
if __name__ == '__main__':
hello()
总结
将文本居中显示在Python中有多种方法,具体选择哪种方法取决于你的具体需求和使用场景。无论是简单的字符串操作,还是复杂的GUI应用,甚至是日志文件、数据分析、报表生成、游戏开发和命令行工具,都有相应的解决方案。希望本篇文章能为你提供全面的参考,帮助你在不同场景下实现文本居中显示。
相关问答FAQs:
如何在Python中实现文本居中输出?
在Python中,可以使用字符串的center()
方法来实现文本的居中输出。该方法接受两个参数,第一个是总宽度,第二个是用于填充的字符(默认为空格)。例如,print("Hello".center(20, '*'))
会在输出中将“Hello”居中,并用星号填充到20个字符宽。
是否可以使用格式化方法来居中输出文本?
当然可以!Python的格式化字符串(f-string)或str.format()
方法也支持文本居中。使用f-string时,可以通过{text:^width}
来实现居中。例如,print(f"{'Hello':^20}")
将会在20个字符宽的区域内居中显示“Hello”。
在命令行中输出的文本可以居中吗?
是的,您可以在命令行中使用上述方法来居中输出文本。无论是在Windows还是Linux终端,只需确保设定的宽度适应您的终端窗口。例如,如果您的终端宽度为80个字符,可以将字符串居中输出,命令为:print("Hello".center(80))
。这样可以确保文本在视觉上居中。
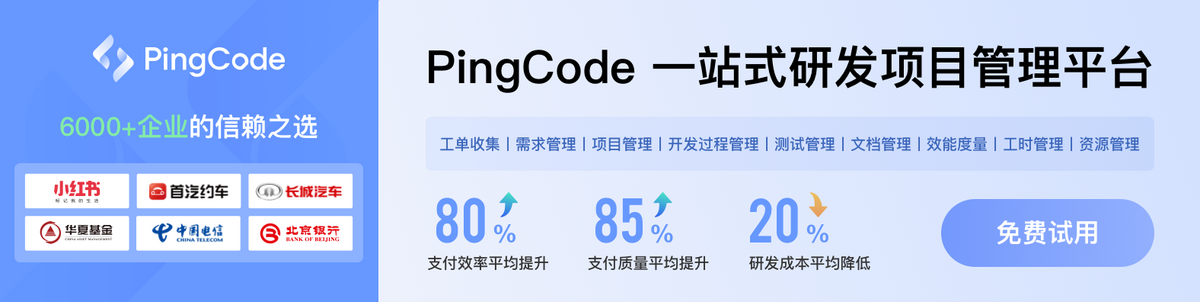