在 Python 类中调用外部函数可以通过多种方式来实现。主要的方法包括:直接调用外部函数、通过实例方法调用、使用类方法调用以及通过静态方法调用。直接导入函数、使用实例方法调用、使用类方法调用、使用静态方法调用。下面将详细解释其中的几种方法,并通过代码示例进行说明。
一、直接导入函数
在类的定义中,可以直接导入并调用外部函数。这种方式最为直接和简单。首先,你需要确保外部函数所在的模块已经被导入,然后在类的方法中直接调用这个函数即可。
# external_module.py
def external_function():
return "Hello from external function!"
# main.py
from external_module import external_function
class MyClass:
def call_external_function(self):
result = external_function()
print(result)
Usage
obj = MyClass()
obj.call_external_function()
在这个例子中,external_function
被直接导入,然后在 MyClass
的方法 call_external_function
中被调用。
二、使用实例方法调用
另一种方法是将外部函数作为参数传递给类的实例方法。这种方式可以在类的实例化过程中动态地决定要调用的外部函数。
# external_module.py
def external_function():
return "Hello from external function!"
# main.py
from external_module import external_function
class MyClass:
def __init__(self, func):
self.func = func
def call_external_function(self):
result = self.func()
print(result)
Usage
obj = MyClass(external_function)
obj.call_external_function()
在这个例子中,external_function
被作为参数传递给 MyClass
的构造方法,然后在 call_external_function
方法中被调用。
三、使用类方法调用
你还可以使用类方法来调用外部函数。这种方法适用于不需要实例化类就能调用外部函数的情况。
# external_module.py
def external_function():
return "Hello from external function!"
# main.py
from external_module import external_function
class MyClass:
@classmethod
def call_external_function(cls):
result = external_function()
print(result)
Usage
MyClass.call_external_function()
在这个例子中,external_function
被 MyClass
的类方法 call_external_function
直接调用。
四、使用静态方法调用
静态方法是另一种调用外部函数的方式。这种方法与类方法类似,但它不会隐式地传递类对象或实例对象。
# external_module.py
def external_function():
return "Hello from external function!"
# main.py
from external_module import external_function
class MyClass:
@staticmethod
def call_external_function():
result = external_function()
print(result)
Usage
MyClass.call_external_function()
在这个例子中,external_function
被 MyClass
的静态方法 call_external_function
直接调用。
五、通过内部封装调用
有时,外部函数可能属于其他类或模块。这时,可以通过内部封装的方法来调用这些外部函数。
# external_module.py
class ExternalClass:
@staticmethod
def external_function():
return "Hello from external function!"
# main.py
from external_module import ExternalClass
class MyClass:
def call_external_function(self):
result = ExternalClass.external_function()
print(result)
Usage
obj = MyClass()
obj.call_external_function()
在这个例子中,ExternalClass
的静态方法 external_function
被 MyClass
的方法 call_external_function
调用。
六、通过依赖注入调用
依赖注入是一种设计模式,可以用来提高代码的可维护性和可测试性。你可以通过依赖注入来调用外部函数。
# external_module.py
def external_function():
return "Hello from external function!"
# main.py
from external_module import external_function
class MyClass:
def __init__(self, external_func):
self.external_func = external_func
def call_external_function(self):
result = self.external_func()
print(result)
Usage
obj = MyClass(external_function)
obj.call_external_function()
在这个例子中,外部函数 external_function
被注入到 MyClass
的实例中,并在 call_external_function
方法中调用。
七、通过闭包调用
闭包是一种函数内部定义函数的方式,可以用来封装外部函数,并在类中调用。
# external_module.py
def external_function():
return "Hello from external function!"
# main.py
def create_closure(external_func):
def inner_function():
return external_func()
return inner_function
from external_module import external_function
class MyClass:
def __init__(self, closure):
self.closure = closure
def call_external_function(self):
result = self.closure()
print(result)
Usage
closure = create_closure(external_function)
obj = MyClass(closure)
obj.call_external_function()
在这个例子中,外部函数 external_function
被封装在闭包 create_closure
中,并在 MyClass
的实例中调用。
八、通过装饰器调用
装饰器是一种高级的函数调用方式,可以用来在类中调用外部函数。
# external_module.py
def external_function():
return "Hello from external function!"
# main.py
from external_module import external_function
def my_decorator(func):
def wrapper():
return func()
return wrapper
class MyClass:
@my_decorator
def call_external_function():
pass
Usage
result = MyClass.call_external_function()
print(result)
在这个例子中,外部函数 external_function
被装饰器 my_decorator
包装,并在 MyClass
的方法 call_external_function
中调用。
总结
在 Python 类中调用外部函数有多种方法,包括直接导入、使用实例方法、类方法、静态方法、内部封装、依赖注入、闭包和装饰器。每种方法都有其适用的场景和优点,具体选择哪种方法取决于你的需求和代码结构。
通过了解和掌握这些方法,你可以在编写 Python 程序时更加灵活地调用外部函数,提高代码的可维护性和可读性。
相关问答FAQs:
如何在Python类中调用外部函数?
在Python中,可以通过在类的方法中直接调用外部定义的函数来实现。只需确保外部函数的作用域可访问,并且在调用时传递正确的参数。例如,可以在类的构造函数中导入外部函数,并在需要的地方调用它。
在类方法中传递参数给外部函数的正确方式是什么?
在类的方法中,可以通过将参数传递给外部函数来实现灵活性。确保在调用外部函数时,使用类的实例属性或方法中的局部变量作为参数。这样可以保持方法间的良好耦合和数据传递。
是否可以在类中使用外部函数的返回值?
绝对可以!在类的方法中调用外部函数后,可以将返回值存储在类的属性中,或直接在方法中使用。这样,可以在类的其他方法中使用这些返回值,增强代码的复用性和逻辑性。
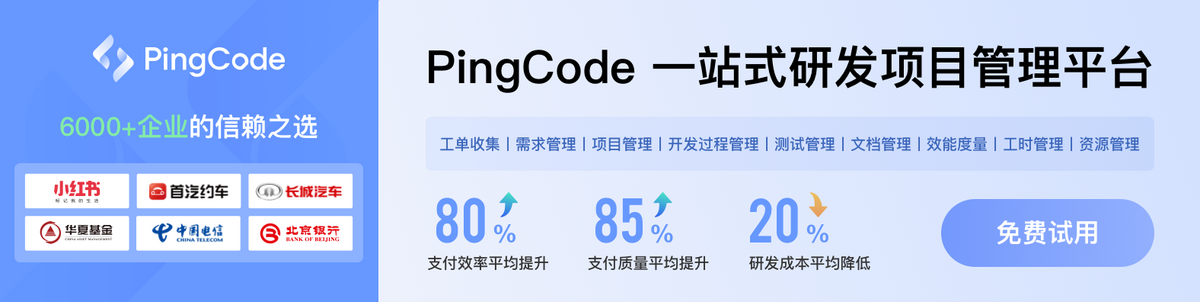