用类创建方法在Python中,可以通过定义类、创建实例、定义方法、调用方法等方式实现。定义类是创建方法的基础,通过类的实例化来生成对象,定义类中的方法以提供对象的功能,最后通过实例化对象调用这些方法。
Python的面向对象编程(OOP)使得代码更加结构化和模块化。下面将详细介绍如何在Python中用类创建方法,并结合实例进行说明。
一、定义类
类是创建方法的基础。在Python中,使用class
关键字来定义一个类。类名通常使用驼峰命名法,即每个单词的首字母大写,比如MyClass
。类中可以包含属性和方法。
class MyClass:
pass
二、初始化方法(构造函数)
在定义类时,通常会定义一个特殊的初始化方法__init__
,用于在创建实例时初始化对象的属性。这个方法是类的构造函数,在创建实例时自动调用。
class MyClass:
def __init__(self, attribute1, attribute2):
self.attribute1 = attribute1
self.attribute2 = attribute2
三、定义方法
在类中定义方法,用于实现对象的行为。方法是类中的函数,第一个参数通常是self
,用于引用类的实例。
class MyClass:
def __init__(self, attribute1, attribute2):
self.attribute1 = attribute1
self.attribute2 = attribute2
def my_method(self):
return f"Attribute 1 is {self.attribute1}, Attribute 2 is {self.attribute2}"
四、创建类的实例
实例化类是创建对象的过程。使用类名加上括号来创建实例,并传递必要的参数。
instance = MyClass("value1", "value2")
五、调用方法
通过实例调用类中的方法,使用点号.
来访问方法。
result = instance.my_method()
print(result) # 输出: Attribute 1 is value1, Attribute 2 is value2
六、示例:创建一个简单的银行账户类
下面是一个更详细的示例,展示如何定义一个表示银行账户的类,并包含存款和取款的方法。
class BankAccount:
def __init__(self, owner, balance=0):
self.owner = owner
self.balance = balance
def deposit(self, amount):
self.balance += amount
return f"Deposit of {amount} successful. New balance is {self.balance}"
def withdraw(self, amount):
if amount > self.balance:
return f"Insufficient funds. Current balance is {self.balance}"
else:
self.balance -= amount
return f"Withdrawal of {amount} successful. New balance is {self.balance}"
def get_balance(self):
return f"Current balance is {self.balance}"
创建一个银行账户实例
account = BankAccount("Alice", 1000)
存款
print(account.deposit(500)) # 输出: Deposit of 500 successful. New balance is 1500
取款
print(account.withdraw(200)) # 输出: Withdrawal of 200 successful. New balance is 1300
查询余额
print(account.get_balance()) # 输出: Current balance is 1300
七、类的继承
类的继承是面向对象编程的重要特性。通过继承,子类可以重用父类的代码,并在此基础上进行扩展和修改。
class SavingsAccount(BankAccount):
def __init__(self, owner, balance=0, interest_rate=0.01):
super().__init__(owner, balance)
self.interest_rate = interest_rate
def add_interest(self):
interest = self.balance * self.interest_rate
self.balance += interest
return f"Interest of {interest} added. New balance is {self.balance}"
创建一个储蓄账户实例
savings_account = SavingsAccount("Bob", 2000, 0.05)
添加利息
print(savings_account.add_interest()) # 输出: Interest of 100.0 added. New balance is 2100
八、类的封装与私有属性
在类中,可以使用双下划线__
来定义私有属性,防止外部直接访问。可以通过定义公共方法来访问和修改私有属性。
class EncapsulatedAccount:
def __init__(self, owner, balance=0):
self.__owner = owner
self.__balance = balance
def deposit(self, amount):
self.__balance += amount
return f"Deposit of {amount} successful. New balance is {self.__balance}"
def withdraw(self, amount):
if amount > self.__balance:
return f"Insufficient funds. Current balance is {self.__balance}"
else:
self.__balance -= amount
return f"Withdrawal of {amount} successful. New balance is {self.__balance}"
def get_balance(self):
return f"Current balance is {self.__balance}"
创建一个封装的账户实例
encapsulated_account = EncapsulatedAccount("Charlie", 3000)
尝试直接访问私有属性(会报错)
print(encapsulated_account.__balance)
通过方法访问私有属性
print(encapsulated_account.get_balance()) # 输出: Current balance is 3000
九、类方法和静态方法
类方法使用@classmethod
装饰器定义,第一个参数是cls
,表示类本身。静态方法使用@staticmethod
装饰器定义,不需要传递self
或cls
参数。
class MyClass:
class_variable = "class variable value"
def __init__(self, instance_variable):
self.instance_variable = instance_variable
@classmethod
def class_method(cls):
return f"Class method called. Class variable is {cls.class_variable}"
@staticmethod
def static_method():
return "Static method called"
调用类方法
print(MyClass.class_method()) # 输出: Class method called. Class variable is class variable value
调用静态方法
print(MyClass.static_method()) # 输出: Static method called
十、总结
使用类创建方法在Python中是面向对象编程的核心。通过定义类、创建实例、定义方法、调用方法,可以实现代码的模块化和重用性。面向对象编程还包括类的继承、封装以及类方法和静态方法的使用,这些特性使得代码更加灵活和易于维护。掌握这些概念和技巧,将有助于你在Python编程中编写更高效和结构化的代码。
相关问答FAQs:
如何在Python中定义和使用类的方法?
在Python中,类的方法是封装在类内部的函数。要定义一个方法,可以在类内部使用def
关键字,并确保方法的第一个参数是self
,这代表类的实例。调用方法时,可以通过实例访问它,例如:实例名.方法名()
。
类的方法与函数有什么区别?
类的方法是与类的实例相关联的,而普通函数则是独立的。方法可以访问实例的属性并对其进行修改,这使得它们在面向对象编程中更为强大。例如,方法可以通过self
访问和修改类的实例变量,而独立函数无法做到这一点。
如何在类中使用静态方法和类方法?
静态方法使用@staticmethod
装饰器定义,不需要self
参数,可以在不依赖类实例的情况下调用。类方法使用@classmethod
装饰器定义,接受类作为第一个参数(通常命名为cls
),可以通过类本身调用,适合于需要访问类属性的情况。这两种方法都为代码结构提供了灵活性。
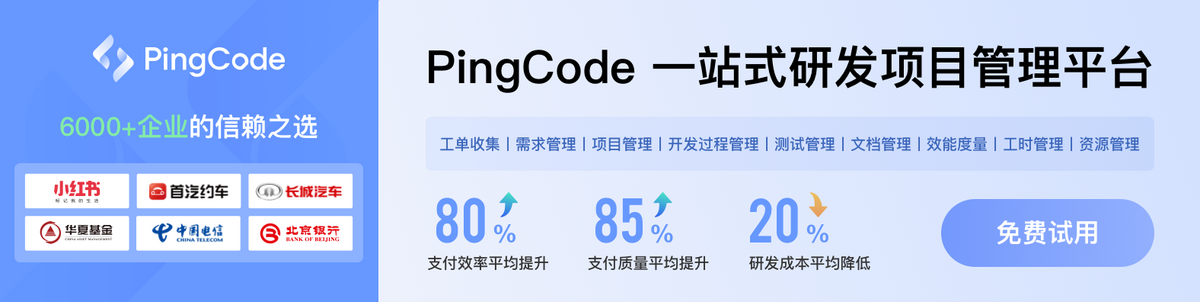