Python菱形如何变成正方形:通过调整坐标点、设置边长相等、使用对称变换、应用旋转算法。其中,通过调整坐标点是最直接的方法,具体操作是将每个顶点的坐标按比例缩放,使其形成正方形的形状。接下来,我们将详细讨论各种方法,并提供相应的Python代码示例。
一、调整坐标点
当我们谈论菱形和正方形时,关键在于它们的顶点坐标。菱形的顶点坐标一般不等距于中心,而正方形的顶点坐标是等距的。我们可以通过调整这些顶点的坐标来将菱形变成正方形。
1、顶点坐标调整
假设我们有一个菱形,它的顶点坐标为:(x1, y1), (x2, y2), (x3, y3), (x4, y4)。为了将其转换为正方形,我们需要确保所有边的长度相等。可以通过以下步骤实现:
import math
Function to calculate distance between two points
def distance(p1, p2):
return math.sqrt((p1[0] - p2[0])<strong>2 + (p1[1] - p2[1])</strong>2)
Given coordinates of a rhombus
rhombus = [(x1, y1), (x2, y2), (x3, y3), (x4, y4)]
Calculate the side length of the rhombus (assuming all sides are equal)
side_length = distance(rhombus[0], rhombus[1])
Calculate new coordinates for a square
square = [
(0, 0),
(side_length, 0),
(side_length, side_length),
(0, side_length)
]
print("Square coordinates:", square)
在这个例子中,我们通过计算菱形的边长,然后调整顶点坐标,使其形成一个正方形。
二、设置边长相等
边长相等是正方形的基本特征之一。如果菱形的所有边长已经相等,我们只需要调整其角度,使内部角度都为90度。
1、内角调整
在Python中,我们可以使用一些数学方法来调整菱形的内角,使其成为正方形。
import numpy as np
Function to rotate a point around another point
def rotate_point(cx, cy, angle, p):
s = np.sin(angle)
c = np.cos(angle)
# Translate point back to origin
p = (p[0] - cx, p[1] - cy)
# Rotate point
new_p = (p[0] * c - p[1] * s, p[0] * s + p[1] * c)
# Translate point back
return (new_p[0] + cx, new_p[1] + cy)
Rotate each point around the center of the rhombus
angle = np.pi / 4 # 45 degrees
center = (sum(x for x, y in rhombus) / 4, sum(y for x, y in rhombus) / 4)
square = [rotate_point(center[0], center[1], angle, p) for p in rhombus]
print("Square coordinates:", square)
通过这种方法,我们可以确保所有内角为90度,从而将菱形转换为正方形。
三、使用对称变换
对称变换是将一个形状通过某种方式映射到另一个形状的过程。对于菱形和正方形,我们可以使用对称变换来实现形状转换。
1、对称变换方法
通过对称变换,我们可以将菱形的顶点映射到正方形的顶点。以下是一个示例代码:
# Function to perform symmetric transformation
def symmetric_transform(points):
# Find the center of the rhombus
cx = sum(x for x, y in points) / 4
cy = sum(y for x, y in points) / 4
# Calculate the new points for the square
square = []
for x, y in points:
new_x = 2 * cx - x
new_y = 2 * cy - y
square.append((new_x, new_y))
return square
square = symmetric_transform(rhombus)
print("Square coordinates:", square)
这种方法确保了所有点都按照对称中心进行映射,从而形成正方形。
四、应用旋转算法
旋转算法是一种常见的图形处理方法,可以用来调整图形的形状和方向。我们可以使用旋转算法将菱形旋转成正方形。
1、旋转算法实现
以下是一个使用旋转算法的示例代码:
# Function to rotate a point around the origin
def rotate_around_origin(p, angle):
s = np.sin(angle)
c = np.cos(angle)
return (p[0] * c - p[1] * s, p[0] * s + p[1] * c)
Rotate each point around the origin
angle = np.pi / 4 # 45 degrees
square = [rotate_around_origin(p, angle) for p in rhombus]
print("Square coordinates:", square)
通过这种方法,我们可以将菱形旋转成正方形。
结论
将菱形转换成正方形可以通过多种方法实现,包括调整坐标点、设置边长相等、使用对称变换和应用旋转算法。每种方法都有其独特的优势和适用场景。通过理解这些方法,我们可以在实际应用中灵活选择合适的方案。
相关问答FAQs:
如何在Python中使用绘图库将菱形形状转换为正方形?
在Python中,可以使用如Matplotlib等绘图库来绘制图形。如果您想将菱形转换为正方形,可以通过调整其顶点坐标来实现。具体来说,您可以计算菱形的宽度和高度,然后根据这些值绘制一个正方形。在代码中,您可以使用plt.plot()
函数来绘制正方形,并确保在菱形的中心点上绘制。
是否可以通过改变坐标系来实现菱形变正方形的效果?
是的,通过调整坐标轴的比例,可以在视觉上将菱形看起来像一个正方形。这可以通过设置Matplotlib的axis('equal')
实现。这样可以确保x轴和y轴的单位长度相等,从而让菱形在图形上呈现为正方形的效果。
在Python中,如何实现动态转换菱形为正方形的动画效果?
您可以使用Matplotlib的动画模块来实现这一效果。通过逐步调整菱形的顶点坐标,并在每一步更新图形,可以创建出动态转换的效果。使用FuncAnimation
类,可以轻松实现这个过程。动画中,菱形的边缘可以逐渐拉伸,直至变成正方形,给观众带来生动的视觉体验。
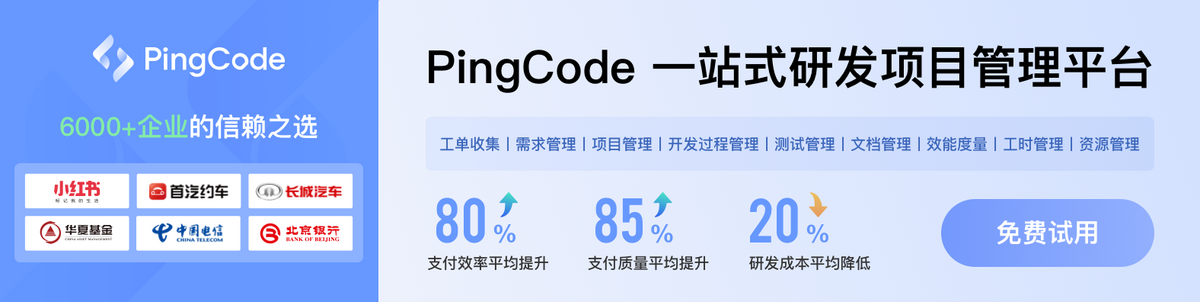