使用Python批量产生txt文件有多种方法:使用循环、创建文件名模板、写入文件内容、使用上下文管理器等。 其中,使用循环来创建文件名和写入内容是最常见的方法。我们可以通过Python的os
模块来操作文件路径,通过with open
语句来确保文件正确地打开和关闭。下面将详细介绍其中一个方法。
一、使用循环创建文件名
要批量生成txt文件,首先需要确定文件名的命名规则,比如可以使用数字递增的方式。使用Python的for
循环,能够轻松实现这一点。
import os
def create_txt_files(directory, num_files):
if not os.path.exists(directory):
os.makedirs(directory)
for i in range(num_files):
file_name = f"file_{i+1}.txt"
file_path = os.path.join(directory, file_name)
with open(file_path, 'w') as file:
file.write(f"This is file number {i+1}")
print(f"{num_files} text files have been created in {directory}")
create_txt_files("output_files", 10)
二、创建文件夹并检查其存在性
在上面的代码中,使用os.makedirs(directory)
来创建存放txt文件的文件夹。如果文件夹已经存在,则无需重复创建。通过这种方式,可以避免因文件夹不存在而导致的错误。
三、使用上下文管理器写入文件内容
上下文管理器(使用with open
语句)可以确保文件在写入操作完成后自动关闭,从而避免资源泄漏。每次循环中,创建一个新的文件并写入内容。这样可以确保每个文件都包含我们预期的内容。
四、动态生成文件内容
在生成文件时,可以根据需求动态生成文件内容。例如,可以根据文件编号、时间戳或其他数据来定制每个文件的内容。
import os
import datetime
def create_txt_files_with_dynamic_content(directory, num_files):
if not os.path.exists(directory):
os.makedirs(directory)
for i in range(num_files):
file_name = f"file_{i+1}.txt"
file_path = os.path.join(directory, file_name)
current_time = datetime.datetime.now().strftime("%Y-%m-%d %H:%M:%S")
with open(file_path, 'w') as file:
file.write(f"This is file number {i+1}\n")
file.write(f"Created on {current_time}\n")
file.write("This content can be customized as needed.")
print(f"{num_files} text files with dynamic content have been created in {directory}")
create_txt_files_with_dynamic_content("output_files_with_dynamic_content", 10)
五、处理大批量文件
当需要生成大量文件时,可以考虑使用多线程或多进程来提高效率。Python的concurrent.futures
模块提供了便捷的接口来实现并行处理。
import os
from concurrent.futures import ThreadPoolExecutor
def create_single_file(file_path, content):
with open(file_path, 'w') as file:
file.write(content)
def create_txt_files_concurrently(directory, num_files):
if not os.path.exists(directory):
os.makedirs(directory)
content_list = [f"This is file number {i+1}" for i in range(num_files)]
file_paths = [os.path.join(directory, f"file_{i+1}.txt") for i in range(num_files)]
with ThreadPoolExecutor(max_workers=10) as executor:
executor.map(create_single_file, file_paths, content_list)
print(f"{num_files} text files have been created in {directory} using concurrent processing")
create_txt_files_concurrently("concurrent_output_files", 100)
六、总结
通过以上方法,我们可以高效地使用Python批量产生txt文件。首先,使用循环创建文件名和写入内容。其次,使用上下文管理器确保文件正确关闭。最后,通过多线程或多进程处理来提高效率,特别是在需要生成大量文件时。希望这些方法能够帮助你在实际项目中更好地管理和生成txt文件。
相关问答FAQs:
如何使用Python快速创建多个txt文件?
您可以使用Python内置的open()
函数结合循环来批量创建txt文件。通过指定文件名和写入内容,您可以轻松生成多个文件。以下是一个简单的示例代码:
for i in range(1, 11): # 生成10个文件
with open(f'file_{i}.txt', 'w') as f:
f.write(f'This is file number {i}')
这段代码会在当前目录下创建10个txt文件,文件名为file_1.txt到file_10.txt。
可以自定义生成的txt文件内容吗?
当然可以!您可以在write()
方法中添加您想要的内容。无论是文本、数字还是其他格式的数据,只需在循环中修改f.write()
的内容即可。例如,您可以将文件内容设为从列表中获取的字符串,或者读取其他文件内容并写入新文件。
如何指定生成的txt文件存放目录?
您可以在open()
函数中指定完整的文件路径来控制文件的存放位置。例如,如果您希望将文件存储在名为output
的文件夹中,可以使用以下代码:
import os
# 创建output文件夹
os.makedirs('output', exist_ok=True)
# 生成txt文件
for i in range(1, 11):
with open(f'output/file_{i}.txt', 'w') as f:
f.write(f'This is file number {i}')
以上代码会在当前目录下创建一个名为output
的文件夹,并将生成的txt文件存储在该文件夹内。
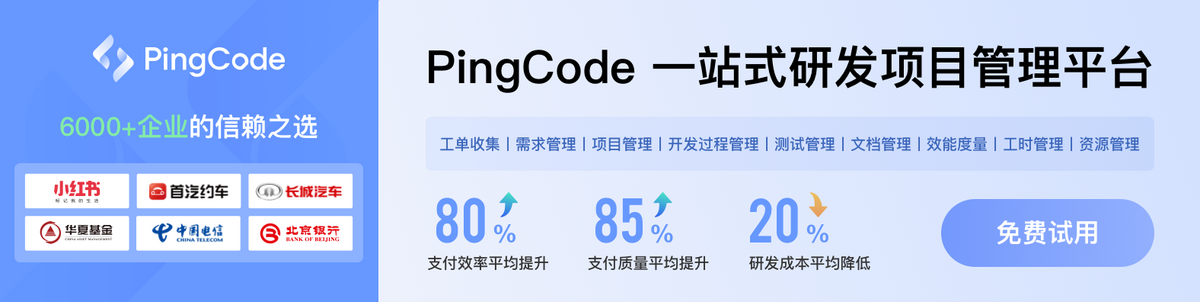