在Python中,你可以使用多种方法来创建新字符串,包括使用字符串连接、格式化、切片、替换等。最常用的方法包括使用加号(+)连接、join()方法、格式化字符串、切片和replace()方法。其中,字符串连接是最直接的一种方法,它通过将两个或多个字符串拼接在一起,形成一个新的字符串。接下来我们将详细介绍这些方法,并提供示例代码。
一、字符串连接
字符串连接是最基本的字符串操作之一,使用加号(+)可以将两个或多个字符串拼接在一起,生成一个新的字符串。
示例代码:
str1 = "Hello"
str2 = "World"
new_str = str1 + " " + str2
print(new_str) # 输出:Hello World
二、使用join()
方法
join()
方法是字符串方法之一,用于将序列中的元素以指定的分隔符连接成一个新的字符串。它比简单的加号连接更高效,特别是当需要连接大量字符串时。
示例代码:
str_list = ["Hello", "World", "Python"]
separator = " "
new_str = separator.join(str_list)
print(new_str) # 输出:Hello World Python
三、格式化字符串
Python提供了多种字符串格式化方式,包括%
操作符、str.format()
方法和f-strings(格式化字符串字面量)。
%
操作符:
name = "Alice"
age = 30
new_str = "Name: %s, Age: %d" % (name, age)
print(new_str) # 输出:Name: Alice, Age: 30
str.format()
方法:
name = "Alice"
age = 30
new_str = "Name: {}, Age: {}".format(name, age)
print(new_str) # 输出:Name: Alice, Age: 30
f-strings(Python 3.6+):
name = "Alice"
age = 30
new_str = f"Name: {name}, Age: {age}"
print(new_str) # 输出:Name: Alice, Age: 30
四、字符串切片
切片是从现有字符串中提取子字符串的一种方法。通过指定起始和结束索引,可以创建新的字符串。
示例代码:
original_str = "Hello, World!"
new_str = original_str[7:12]
print(new_str) # 输出:World
五、使用replace()
方法
replace()
方法用于替换字符串中的指定子字符串,从而生成一个新的字符串。
示例代码:
original_str = "Hello, World!"
new_str = original_str.replace("World", "Python")
print(new_str) # 输出:Hello, Python!
六、使用+
连接多个字符串
除了直接使用+
号进行连接,我们还可以将多个字符串变量和常量连接起来。
示例代码:
str1 = "Hello"
str2 = "World"
str3 = "Python"
new_str = str1 + " " + str2 + " " + str3
print(new_str) # 输出:Hello World Python
七、使用三重引号创建多行字符串
三重引号('''
或"""
)允许我们创建包含多行文本的字符串,适用于需要在字符串中包含换行符的情况。
示例代码:
multi_line_str = """This is a multi-line
string that spans
multiple lines."""
print(multi_line_str)
八、字符串重复
使用*
运算符可以将字符串重复指定的次数。
示例代码:
str1 = "Hello"
new_str = str1 * 3
print(new_str) # 输出:HelloHelloHello
九、字符串模板类
Python的string
模块提供了Template
类,可以通过占位符进行字符串替换。
示例代码:
from string import Template
template = Template("Hello, $name!")
new_str = template.substitute(name="World")
print(new_str) # 输出:Hello, World!
十、组合多个方法
在实际应用中,我们可以组合使用多种方法来创建新的字符串,以适应不同的需求。
示例代码:
name = "Alice"
age = 30
hobby_list = ["reading", "hiking", "coding"]
使用格式化字符串和 join() 方法
new_str = f"Name: {name}, Age: {age}, Hobbies: {', '.join(hobby_list)}"
print(new_str) # 输出:Name: Alice, Age: 30, Hobbies: reading, hiking, coding
十一、使用正则表达式替换字符串
正则表达式提供了强大的字符串搜索和替换功能,可以通过re
模块使用。
示例代码:
import re
original_str = "The quick brown fox jumps over the lazy dog."
pattern = r"\bfox\b"
replacement = "cat"
new_str = re.sub(pattern, replacement, original_str)
print(new_str) # 输出:The quick brown cat jumps over the lazy dog.
十二、使用字符串插值
在某些特定场景下,字符串插值可以提供更简洁的字符串创建方法。
示例代码:
name = "Alice"
hobby = "reading"
new_str = "My name is %s and I love %s." % (name, hobby)
print(new_str) # 输出:My name is Alice and I love reading.
十三、从列表或字典创建新字符串
我们可以从列表或字典等数据结构中提取数据,并将其组合成新的字符串。
示例代码:
info_dict = {"name": "Alice", "age": 30, "hobbies": ["reading", "hiking", "coding"]}
使用字典解包和格式化字符串
new_str = "Name: {name}, Age: {age}, Hobbies: {hobbies}".format(info_dict)
print(new_str) # 输出:Name: Alice, Age: 30, Hobbies: ['reading', 'hiking', 'coding']
十四、使用内置str()
函数
str()
函数可以将其他数据类型转换为字符串。
示例代码:
num = 123
new_str = str(num)
print(new_str) # 输出:123
十五、结合文件操作
我们可以从文件中读取数据,并将其处理后创建新的字符串。
示例代码:
with open("example.txt", "r") as file:
data = file.read()
new_str = data.replace("old_word", "new_word")
print(new_str)
十六、使用字符串方法capitalize()
和title()
这些方法可以改变字符串的大小写,从而生成新的字符串。
示例代码:
original_str = "hello, world!"
new_str1 = original_str.capitalize()
new_str2 = original_str.title()
print(new_str1) # 输出:Hello, world!
print(new_str2) # 输出:Hello, World!
十七、使用字符串方法upper()
和lower()
这些方法可以将字符串转换为大写或小写,从而生成新的字符串。
示例代码:
original_str = "Hello, World!"
new_str1 = original_str.upper()
new_str2 = original_str.lower()
print(new_str1) # 输出:HELLO, WORLD!
print(new_str2) # 输出:hello, world!
十八、使用translate()
方法
translate()
方法可以通过映射表替换字符串中的字符,从而生成新的字符串。
示例代码:
original_str = "hello, world!"
trans_table = str.maketrans("aeiou", "12345")
new_str = original_str.translate(trans_table)
print(new_str) # 输出:h2ll4, w4rld!
十九、字符串常量拼接
使用字符串常量拼接可以减少代码中的硬编码,提高代码的可维护性。
示例代码:
greeting = "Hello"
target = "World"
new_str = f"{greeting}, {target}!"
print(new_str) # 输出:Hello, World!
二十、通过函数生成新字符串
定义函数来创建新的字符串可以提高代码的可读性和复用性。
示例代码:
def greet(name):
return f"Hello, {name}!"
new_str = greet("Alice")
print(new_str) # 输出:Hello, Alice!
通过以上多种方法,我们可以灵活地在Python中创建新的字符串,以适应不同的应用场景和需求。每种方法都有其独特的优势和适用场景,选择合适的方法可以提高代码的效率和可读性。
相关问答FAQs:
如何在Python中创建一个空字符串?
在Python中,可以通过简单的赋值来创建一个空字符串。使用双引号或单引号均可,例如:empty_string = ""
或 empty_string = ''
。这将创建一个不包含任何字符的字符串对象。
Python中字符串拼接的最佳方法是什么?
字符串拼接可以通过多种方式实现。常用的方法包括使用加号(+
)运算符、join()
方法和格式化字符串(如f-string)。例如,使用加号可以这样写:new_string = str1 + str2
。而使用join()
方法则适合合并多个字符串,如:new_string = ''.join([str1, str2])
,这在处理大量字符串时更高效。
如何在Python中对字符串进行修改或替换?
字符串在Python中是不可变的,这意味着一旦创建就无法修改。如果需要对字符串进行修改或替换,可以使用replace()
方法。示例代码如下:new_string = original_string.replace("old", "new")
,这将返回一个新的字符串,其中所有“old”被替换为“new”。此外,使用切片和连接也能实现类似效果。
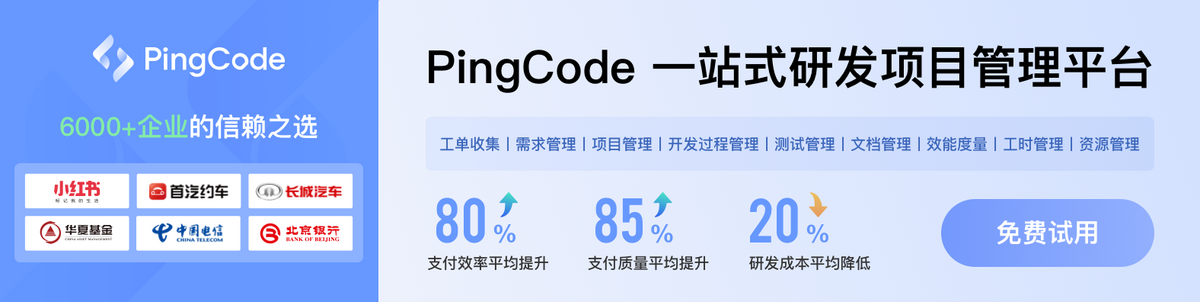