在Python中去掉字符串中的换行符(\n
)的方法有很多,最常用的是使用字符串的内置方法。strip()、rstrip()、replace()都可以用来去除字符串中的换行符,其中strip()方法去除字符串开头和结尾的空白字符,包括换行符,是最常用的一种。
strip()方法去除字符串开头和结尾的空白字符,这些空白字符包括空格、制表符、换行符等。通过使用strip()方法,开发者能够轻松地清理字符串数据,确保字符串的内容在处理和存储时不包含多余的空白字符,从而提高数据的质量和一致性。
例如,考虑一个包含换行符的字符串:
string_with_newline = "Hello, World!\n"
cleaned_string = string_with_newline.strip()
print(cleaned_string)
在上面的例子中,strip()
方法被用来去除字符串开头和结尾的换行符,因此输出将是"Hello, World!",不再包含换行符。
接下来,我们将详细介绍Python中去掉字符串中的换行符的多种方法,并探讨它们的优缺点和适用场景。
一、使用strip()方法
strip()
方法用于去除字符串开头和结尾的空白字符,包括换行符、制表符、空格等。它是去除字符串开头和结尾空白字符最简单和最常用的方法。
string_with_newline = "Hello, World!\n"
cleaned_string = string_with_newline.strip()
print(cleaned_string)
在这个例子中,strip()
方法去掉了字符串末尾的换行符,输出为"Hello, World!"。该方法适用于需要同时去除字符串开头和结尾的空白字符的情况。
需要注意的是,strip()
方法不会影响字符串中间的空白字符:
string_with_internal_newline = "Hello,\nWorld!"
cleaned_string = string_with_internal_newline.strip()
print(cleaned_string)
在这个例子中,字符串内部的换行符不会被去除,输出为"Hello,\nWorld!"。
二、使用rstrip()方法
rstrip()
方法用于去除字符串末尾的空白字符,包括换行符。它与strip()
方法类似,但只作用于字符串的末尾。
string_with_newline = "Hello, World!\n"
cleaned_string = string_with_newline.rstrip()
print(cleaned_string)
在这个例子中,rstrip()
方法去掉了字符串末尾的换行符,输出为"Hello, World!"。该方法适用于需要去除字符串末尾的空白字符,但保留开头空白字符的情况。
同样,rstrip()
方法不会影响字符串中间的空白字符:
string_with_internal_newline = "Hello,\nWorld!"
cleaned_string = string_with_internal_newline.rstrip()
print(cleaned_string)
在这个例子中,字符串内部的换行符不会被去除,输出为"Hello,\nWorld!"。
三、使用replace()方法
replace()
方法用于将字符串中的指定子字符串替换为其他子字符串。通过将换行符替换为空字符串,可以去除字符串中的换行符。
string_with_newline = "Hello, World!\n"
cleaned_string = string_with_newline.replace("\n", "")
print(cleaned_string)
在这个例子中,replace()
方法将字符串中的换行符替换为空字符串,输出为"Hello, World!"。该方法适用于需要去除字符串中所有换行符的情况。
需要注意的是,replace()
方法会影响字符串中所有匹配的子字符串:
string_with_internal_newline = "Hello,\nWorld!\n"
cleaned_string = string_with_internal_newline.replace("\n", "")
print(cleaned_string)
在这个例子中,字符串中的所有换行符都会被去除,输出为"Hello,World!"。
四、使用split()和join()方法
通过使用split()
方法将字符串按换行符分割为多个子字符串,再使用join()
方法将这些子字符串重新连接,可以去除字符串中的换行符。
string_with_newline = "Hello, World!\n"
cleaned_string = "".join(string_with_newline.split())
print(cleaned_string)
在这个例子中,split()
方法将字符串按换行符分割为多个子字符串,join()
方法将这些子字符串重新连接,输出为"Hello,World!"。该方法适用于需要去除字符串中所有换行符,并将字符串重新连接的情况。
需要注意的是,split()
方法会分割字符串中的所有空白字符,包括空格和制表符:
string_with_whitespace = "Hello, World! \n"
cleaned_string = "".join(string_with_whitespace.split())
print(cleaned_string)
在这个例子中,字符串中的所有空白字符都会被去除,输出为"Hello,World!"。
五、使用正则表达式(re模块)
通过使用Python的re
模块,可以使用正则表达式来匹配和替换字符串中的换行符。
import re
string_with_newline = "Hello, World!\n"
cleaned_string = re.sub(r"\n", "", string_with_newline)
print(cleaned_string)
在这个例子中,re.sub()
方法使用正则表达式\n
匹配换行符,并将其替换为空字符串,输出为"Hello, World!"。该方法适用于需要使用正则表达式匹配和替换字符串中的换行符的情况。
正则表达式提供了强大的字符串匹配和替换功能,可以处理更复杂的字符串操作:
string_with_multiple_newlines = "Hello, \nWorld!\n\n"
cleaned_string = re.sub(r"\n+", " ", string_with_multiple_newlines)
print(cleaned_string)
在这个例子中,正则表达式\n+
匹配一个或多个连续的换行符,并将其替换为空格,输出为"Hello, World! "。
六、使用translate()方法
translate()
方法用于将字符串中的指定字符映射为其他字符。通过使用str.maketrans()
方法创建字符映射,可以去除字符串中的换行符。
string_with_newline = "Hello, World!\n"
translation_table = str.maketrans("", "", "\n")
cleaned_string = string_with_newline.translate(translation_table)
print(cleaned_string)
在这个例子中,str.maketrans("", "", "\n")
方法创建一个字符映射,将换行符映射为空字符串,translate()
方法根据该映射去除字符串中的换行符,输出为"Hello, World!"。该方法适用于需要根据字符映射去除字符串中特定字符的情况。
七、总结
去掉字符串中的换行符可以通过多种方法实现,包括strip()
、rstrip()
、replace()
、split()
和join()
、正则表达式和translate()
方法。每种方法都有其优缺点和适用场景,开发者可以根据具体需求选择合适的方法。
- strip()方法:去除字符串开头和结尾的空白字符,包括换行符。
- rstrip()方法:去除字符串末尾的空白字符,包括换行符。
- replace()方法:将字符串中的指定子字符串替换为其他子字符串,适用于去除字符串中所有换行符。
- split()和join()方法:将字符串按换行符分割为多个子字符串,再将这些子字符串重新连接,适用于去除字符串中所有换行符并重新连接。
- 正则表达式(re模块):使用正则表达式匹配和替换字符串中的换行符,适用于处理更复杂的字符串操作。
- translate()方法:根据字符映射去除字符串中特定字符。
通过理解和掌握这些方法,开发者可以在不同的场景中灵活地去除字符串中的换行符,提高数据处理的效率和质量。
相关问答FAQs:
如何在Python中删除字符串末尾的特定字符?
在Python中,可以使用rstrip()
方法来删除字符串末尾的特定字符。如果想要去掉字符串末尾的'n'字符,可以使用如下代码:
string = "example stringn"
result = string.rstrip('n')
print(result) # 输出: "example string"
rstrip()
方法会删除字符串末尾的所有指定字符,直到遇到第一个不匹配的字符为止。
在Python中,是否可以使用正则表达式去掉末尾的字符?
是的,Python的re
模块可以利用正则表达式来删除字符串末尾的特定字符。示例如下:
import re
string = "example stringn"
result = re.sub('n$', '', string)
print(result) # 输出: "example string"
这里的n$
表示匹配字符串末尾的'n'字符,通过re.sub()
函数将其替换为空字符串。
有没有其他方法可以去掉字符串末尾的'n'字符?
除了rstrip()
和正则表达式,字符串切片也是一种有效的方法。例如:
string = "example stringn"
if string.endswith('n'):
result = string[:-1]
else:
result = string
print(result) # 输出: "example string"
这种方法通过检查字符串是否以'n'结尾,并利用切片来去掉最后一个字符。
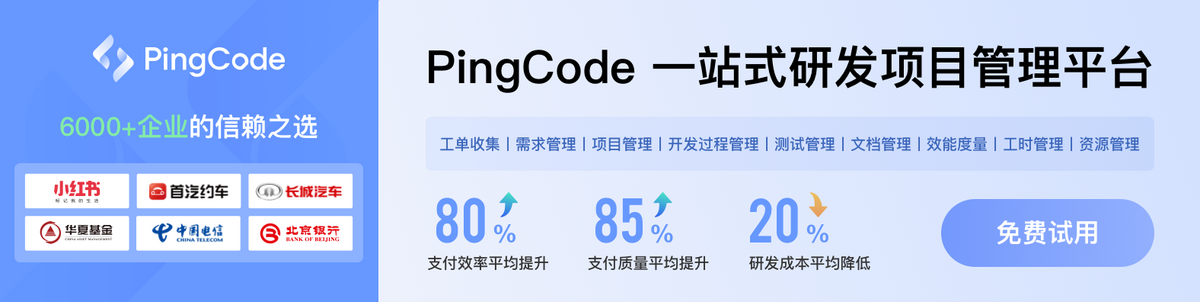